App model in an Ionic app.
Introduction
Models help define the data structure for an app in order to simplify development. In general, a model allows mimicking real-life scenarios in your app. A model is a kind of a custom structure that can be used to create new objects based on itself. For instance, if you are building an app that allows you to make orders, you can define a Customer object. Variables created in Scope can also use models as structure templates so a single customer, or a list of customers, can be saved in variables that use this model as a template.
Go to Project > Model to define models.
Type a name for a new model and click Add to create it. Create the data structure you need by clicking the Add button and specifying field names and types. Here is an example of an imaginary customer structure:

To collapse or expand all model items, click the corresponding links at the top right corner.
Model API
Method summary
Method | Description |
---|---|
EntityAPI(modelName_or_Path, defaults) | Reproduces specified model structure in object or part of object. |
EntityAPI
Reproduces specified model structure in object or part of object.
Parameters:
- modelName – Name of the defined model to reproduce.
- Path – syntax construction for getting (core) part of the model.
defaults – Plain object that is used to extend object structure.
Examples
To create an object with a predefined structure based on a certain model, use the following method:
Apperyio.EntityAPI('modelName', defaults );
Here defaults is an optional defaults parameter. That is, if you need to use a structure, and can already set up some fields, then you can pass a part of the object (in compliance with the structure in depth) in this variable so it will be added to a result of generation from the model.
The following code will reproduce the customer model structure in customerObject:
var customerObject = Apperyio.EntityAPI('customer');
console.log(customerObject);//Object {id: 0, phones: Array[0], name: "", firstOrder: false}
By using the optional defaults parameter that receives a plain object, you can extend the final object structure:
var plainObject = {"parameter":"value", "firstOrder": true};
var customerObject = Apperyio.EntityAPI('customer', plainObject);
console.log(customerObject);//Object {id: 0, phones: Array[0], name: "", firstOrder: true, parameter: "value"}
In the case of a nonexistent model specified in the EntityAPI(...) function, the following error will occur:
TypeNotFoundError
You can use a model name as a method argument, separating model properties with dots.
Here is how to use dot-notation as a path inside the object.
Autocomplete
Apperyio.EntityAPI(
code assist is available here. Press Ctrl+Space)
This code will return as an Object (or simple type or Array) which exists in property3 in the full reproduced Model object:
Model.property1.property2.property3
The following will return as an Object (or simple type or Array) which exists in propertyX in any item inside the array in propertyN and sub-item property propertyM in full reproduced Model object (with at least one item in propertyN):
Model.propertyN.[i].propertyM.propertyX
Supported Data Types
A model can be the Array or Object type.
When defining a model, you can use the following data types to specify the type for a specific model field:
- String
- Boolean
- Number
- Array
- Object
Using Scope
Use SCOPE to create variables. An Object or Array variable created in SCOPE of an index screen will be available in the code of all the app pages. Please note it still cannot be accessed from mapping.
Example of using Model
Let’s say you need to create a simple application that fills a list with some service data using a model.
- Go to Project > Model to create a new model of Object type named Article.
- Add properties header (String), text (String) and rating (Number) to the model item.
- Create a new model of Array type named articlesList, select Article for its item type.
- Create a new model of Object type named ShoppingCart, and add an Object named Category to it.
- Add property Articles of articlesList type to to the model object item:
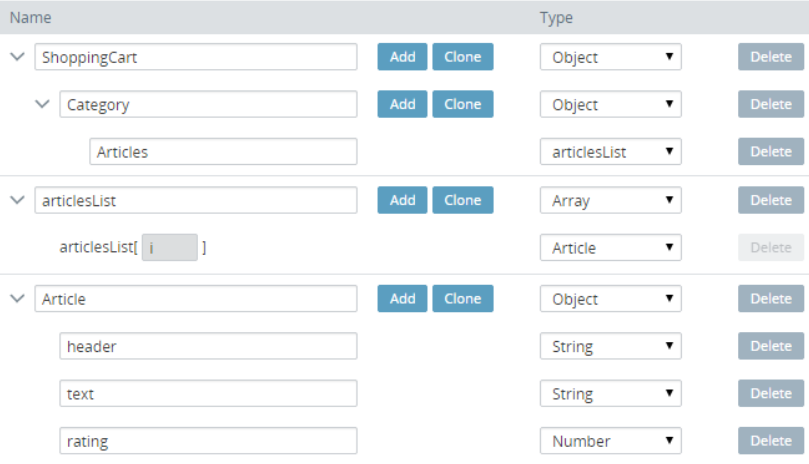
- Click CREATE NEW > Service to create a REST service called getArticles.
- Open the service Response tab and select Array type for a root item and Object type for the array item.
- Add properties header (String), content (String) and rating(Number) for the array item.
- Open the service Echo tab, enable echo, and paste the following echo code:
[{
"header": "Article1",
"content": "Lorem ipsum dolor sit amet",
"rating": 1
}, {
"header": "Article 2. The Cool",
"content": "Some text...",
"rating": 5
}, {
"header": "Another other headline...",
"content": "Important text",
"rating": 4
}]
- Go to the SCOPE tab of Screen1. Create a variable of articlesList type named articles. Create a variable of Article type named article. Create a variable of String type named header.
- Add add() function with the following code:
// We already have a variable named articles, but you could create it here using this code
//$scope.articles = Apperyio.EntityAPI( "ShopingCart.Category.Articles" );
// Now you can add an article object having not a full description. Other fields will be fulled with default values ("", 0 and false)
$scope.articles.push( Apperyio.EntityAPI( 'ShoppingCart.Category.Articles.[i]', { header: $scope.header } ) );
- Open init() function from the snippets drop-down list, select Invoke service to add an appropriate snippet. Press , then + to automatically input the service name.
- Click the Mapping button in Success function.
- Set the following mapping and click Save & Replace:
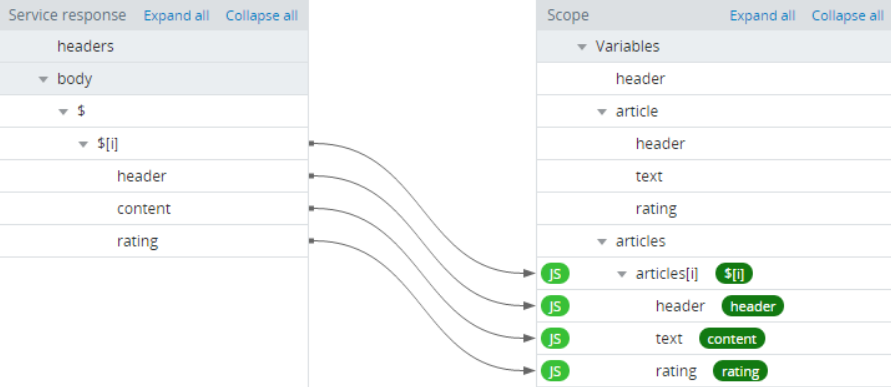
- Here we map content to text, as the objects differ. If an article object contained a field named content instead of text you could only map $[i] -> articles[i], and all the response items would have been copied automatically.
- Open the DESIGN tab. Drag an Input component to the page, and add property ng-model = header to it.
- Drag a Button to the page and set properties Text = Add item and ng-click = add() for this button.
- Add a List component and leave only a list item in it.
- Select a list item, add the attribute ng-repeat = article in articles
- Set the property Text = {{article.text}}
- Set the following properties values: Header = {{article.header}} and Badge = {{article.rating}} (please note, for Ionic you need to expand Heading.text and Item Badge.text first).
- Click TEST:
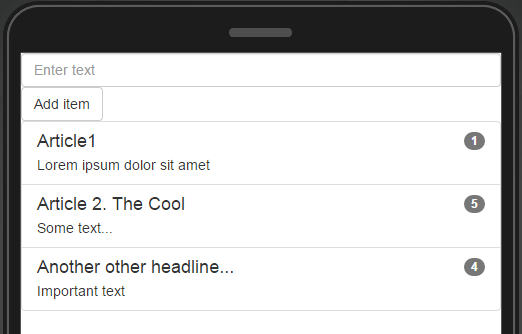
- Now you can add a new item.
- A new item is added, but not all the fields are filled (text is empty and rating = 0).