Using the Contacts service in an Ionic app
Introduction
In this tutorial, you will learn how to use the Appery.io Contacts Service based on the Cordova API in an AngularJS app.
The app will look like:
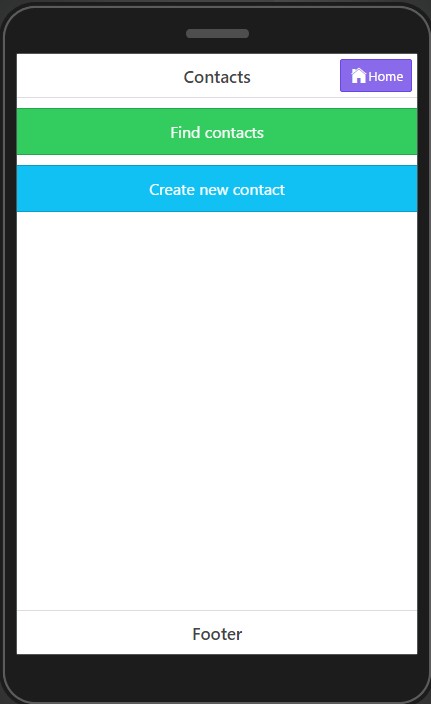
Important Note!
Note that the option of creating new apps with the Ionic framework was removed but we still support the projects that were created with it earlier.
Apache Cordova (PhoneGap)
Apache Cordova (PhoneGap) is automatically included when you create a new project in Appery.io. The Contacts Plugin component used in Appery.io is the cross-platform Cordova Contacts plugin.
Note
To learn more about the component, its objects and methods or any other settings and options, please visit the Apache Cordova plugin contacts page.
Cordova contact object vs Appery.io contact object
An Appery.io contact object is just a JSON object that describes contact. It can’t be used in save and remove services.
A Cordova contact object is a Javascript object with methods. It can be used in any of services. But it can only be created by Contact_create or Contact_clone services.
There is no difference in the structure of these objects.
If the documentation does not specify the object type, either of them can be used.
Services
Appery.io Contacts plugin contains the following services:
Contact_create
Creates a contact object, however, it doesn’t save it to the device’s contacts list.
Request params: contact object. All fields are optional.
Response params: Cordova contact object.
Contact_find
Finds contacts using a filter. Request params:
- options.filter (String) – The search string. (Default: "").
- options.multiple (Boolean) – Determines if the find operation returns multiple contacts. (Default: false).
- params.desiredFields (String) – Contact fields to be returned back. If specified, the resulting Contact object only features values for these fields. (Default: "*")
Response params: list of Cordova contact objects.
Contact_clone
Returns a new Cordova contact object that is a deep copy of the calling object, with the id property is set to null.
Request params: contact object. All fields are optional.
Response params: Cordova contact object.
Contact_save
Saves a new contact to the device’s contacts database, or updates an existing contact if contact with the same ID already exists.
Request params: Cordova contact object. All fields are optional.
Response params: Cordova contact object.
Contact_remove
Removes the contact from the device’s contacts database, otherwise executes an error callback with a ContactError object.
Request params: Cordova contact object. All fields are optional.
No response.
Contact_pick
Launches the Contact Picker to select a single contact.
No request params.
Response params: Cordova contact object.
Models
By default, the plug-in also contains the following models (Project > Model):
aioContact
- id: A globally unique identifier.
- displayName: The name of the contact, suitable for display to end users.
- name: An object containing all components of a persons name.
- nickname: A casual name by which to address the contact.
- phoneNumbers: An array of all the contact’s phone numbers.
- emails: An array of all the contact’s email addresses.
- addresses: An array of all the contact’s addresses.
- ims: An array of all the contact’s IM addresses.
- organizations: An array of all the contact’s organizations.
- birthday: The birthday of the contact.
- note: A note about the contact.
- photos: An array of the contact’s photos.
- categories: An array of all the user-defined categories associated with the contact.
- urls: An array of web pages associated with the contact.
aioContactField
- type: A string that indicates what type of field this is, home for example.
- value: The value of the field, such as a phone number or email address.
- pref: Should be set to true if this ContactField contains the user’s preferred value.
aioContactAddress
- pref: Set to true if this ContactAddress contains the user’s preferred value.
- type: A string indicating what type of field this is, home for example.
- formatted: The full address formatted for display.
- streetAddress: The full street address.
- locality: The city or locality.
- region: The state or region.
- postalCode: The zip code or postal code.
- country: The country name.
aioContactOrganization
- pref: Should be set to true if this ContactOrganization contains the user’s preferred value.
- type: A string that indicates what type of field this is, home for example.
- name: The name of the organization.
- department: The department the contract works for.
- title: The contact’s title at the organization.
aioContactName
- formatted: The complete name of the contact.
- familyName: The contact’s family name.
- givenName: The contact’s given name.
- middleName: The contact’s middle name.
- honorificPrefix: The contact’s prefix (example Mr. or Dr.).
- honorificSuffix: The contact’s suffix (example Esq.).
Creating App
Design
- Create a new blank Appery.io Bootstrap & AngularJS or Ionic & AngularJS project. You will get two default pages: index and Screen1.
- Define the Header for the index page: under the PROPERTIES tab, set its Text value to Contacts.
- Add a Button to the right Header Button: Text = Home, Width = Inline, for Attribute, set: navigate-to = main.
- Now, expand its Icon property and set its Position to left and Style to ion-ios-home:
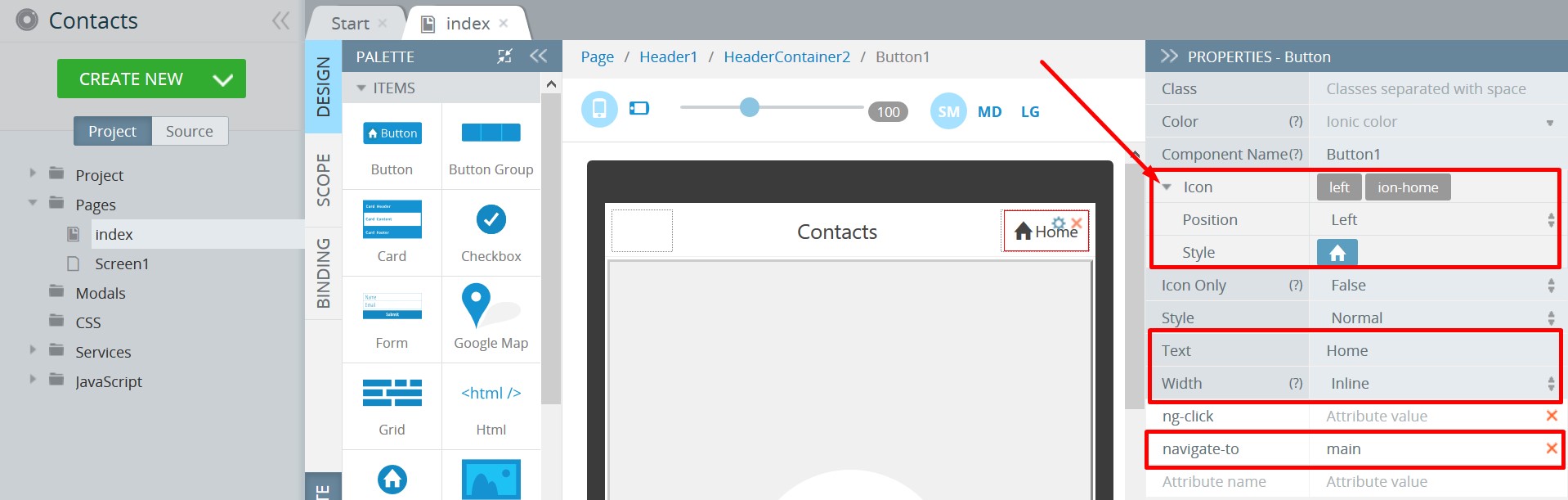
index page
- Now, under the Pages folder, select Screen1 and by clicking the cog icon, rename it to Main, then create the following UI consisting of two Button components.
Set the next properties for the first one: Text = Find contacts, Width = Full, for Attribute, set: navigate-to = find; for the second: Text = Create new contact, Width = Full, navigate-to = create respectively. - CREATE NEW > Page. Name it Create.
- Add the Grid with four Grid Row components having two Grid Cell components each.
- Drag and drop a Text component to each left Grid Cell of the Grid Rows and an Input to each Grid Cell on the right.
- Configure the Text components with the next Text properties: Name, Family Name, Display Name, and Phone.
- For the Inputs, define their ng-model fields as: $parent.name.givenName, $parent.name.familyName, $parent.displayName, and $parent.phoneNumber correspondingly.
- Lastly, place another Button: Text = Save, Width = Full, and ng-click = createContact().
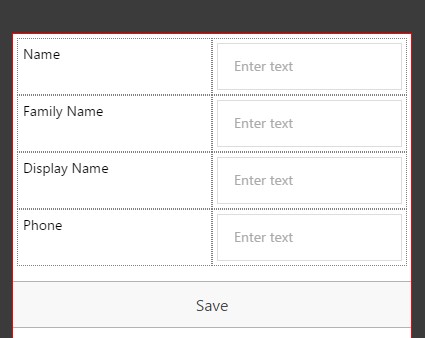
- Now, create another page: Find and define the following UI:
- Place a Grid component with two Grid Cells.
- Add an Input to the left Grid Cell and define: Placehoder = Filter... , ng-model = $parent.query.
- Drag & drop a Button to the Cell on the right: Text = cleared, Width = Inline, and ng-click = findContacts(). Add a search Icon: Position = left, Style = ion-ios-search-strong.
- Place another Grid with two Grid Rows having two Grid Cells each.
- Drag a Text component to each of the four Cells.
- Configure the Text components (from left to right) with the next Text properties: Name, Phone, {{contact.displayName}}, and {{phone.value}}.
- Define the lastText component: ng-repeat = phone in contact.phoneNumbers.
- Add the attribute to the second row: ng-repeat = contact in contacts.
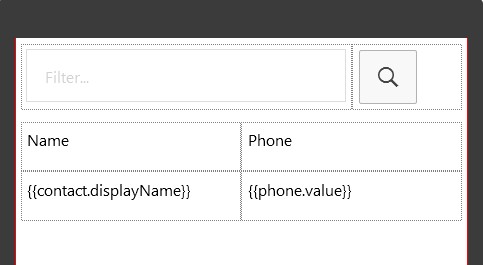
- Lastly, go to Project > Routing and set the following routing:

Now, let’s create services and variables and bind them with UI components.
Adding a Contacts plug-in
To add the Contacts services:
- From Projects view; CREATE NEW > From Plugin > Apperyio Contacts Service, and click Import selected plugins. New services will be listed under the Services folder.
- If you open any of the services, you’ll see that everything has been preconfigured:
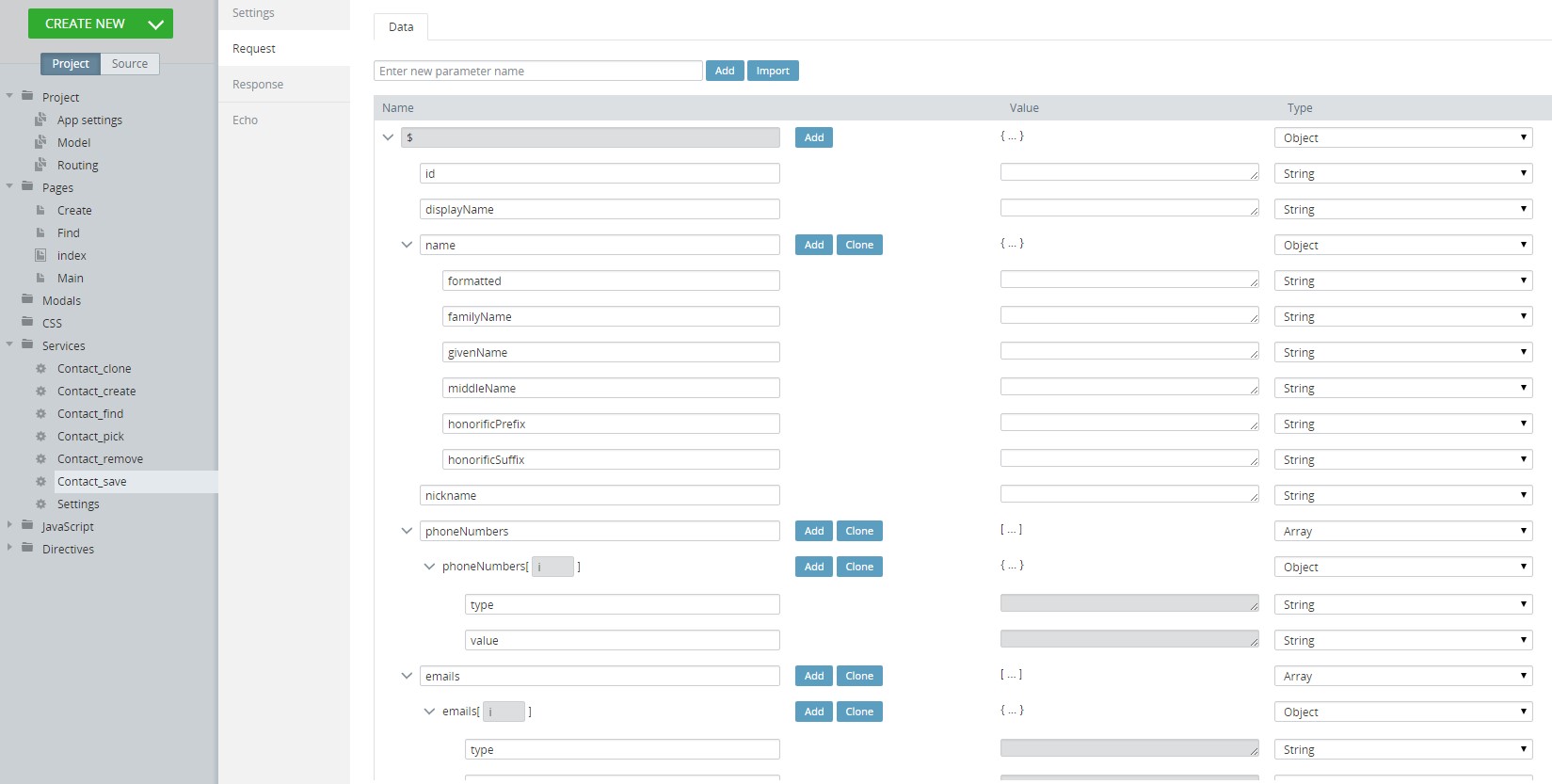
Scope
- To add the following variables, go to SCOPE:
- of theFind page: contacts (Type = aioContactsArray) and query (Type = *String***);
- of theCreate page: name (Type = aioContactName), displayName, phoneNumber (Type of both = String*), contact (Type = aioContact*), and phoneNumbers (Type = aioContactFieldsArray). After native services have been added to the app, they can be called. Invoking a native service is very similar to invoking a REST service.
- To call the added services, first, switch to the Find page, Functions view, click Edit for the init function and paste this code:
$scope.contacts = [];
$scope.query = '';
3.Now, add a new Scope function for the Find page: findContacts.
4. Next, click Edit for the findContacts function, opening the function editor.
5. In the editor, click the arrow to filter snippets, select Invoke service, and click Insert snippet.
6. Then, delete the text service_name in the code and click + to select Contact_find.
7. After auto completing, the service is added to the function code and you can click response Mapping to map the service to the page:

Note
Clicking on the upper Mapping button defines the service request, while clicking on the lower one defines the service response.
Add the next code after var requestData = {:
params: {
fields: ["displayName", "phoneNumbers"] //we'll search by these fields
},
options: {
filter: $scope.query,
multiple: true
}
The final result:
var requestData = {
params: {
fields: ["displayName", "phoneNumbers"] //we'll search by these fields
},
options: {
filter: $scope.query,
multiple: true
}};
Apperyio.get("Contact_find")(requestData).then(
function(success){
(function mapping8541(success, $scope){
var contacts_scope = $scope.contacts;
contacts_scope = success;
$scope.contacts = contacts_scope;
})(success, $scope);
},
function(error){
},
function(notify){
});
// Function code
- Switch to the Create page, then go to SCOPE and click Edit for the init function to paste this code:
$scope.clearVars();
- Now, create two more functions: createContact and clearVars.
- Click Edit for the createContact function and add the following code to the top:
$scope.phoneNumbers = [];
$scope.phoneNumbers.push(new ContactField('mobile', $scope.phoneNumber, true));
- Now, invoke the first service: Contact_create.
- After auto completing, the service is added to the function code and you can click Mapping to map the service to the page.
- Now, click the upper Mapping button and create this:
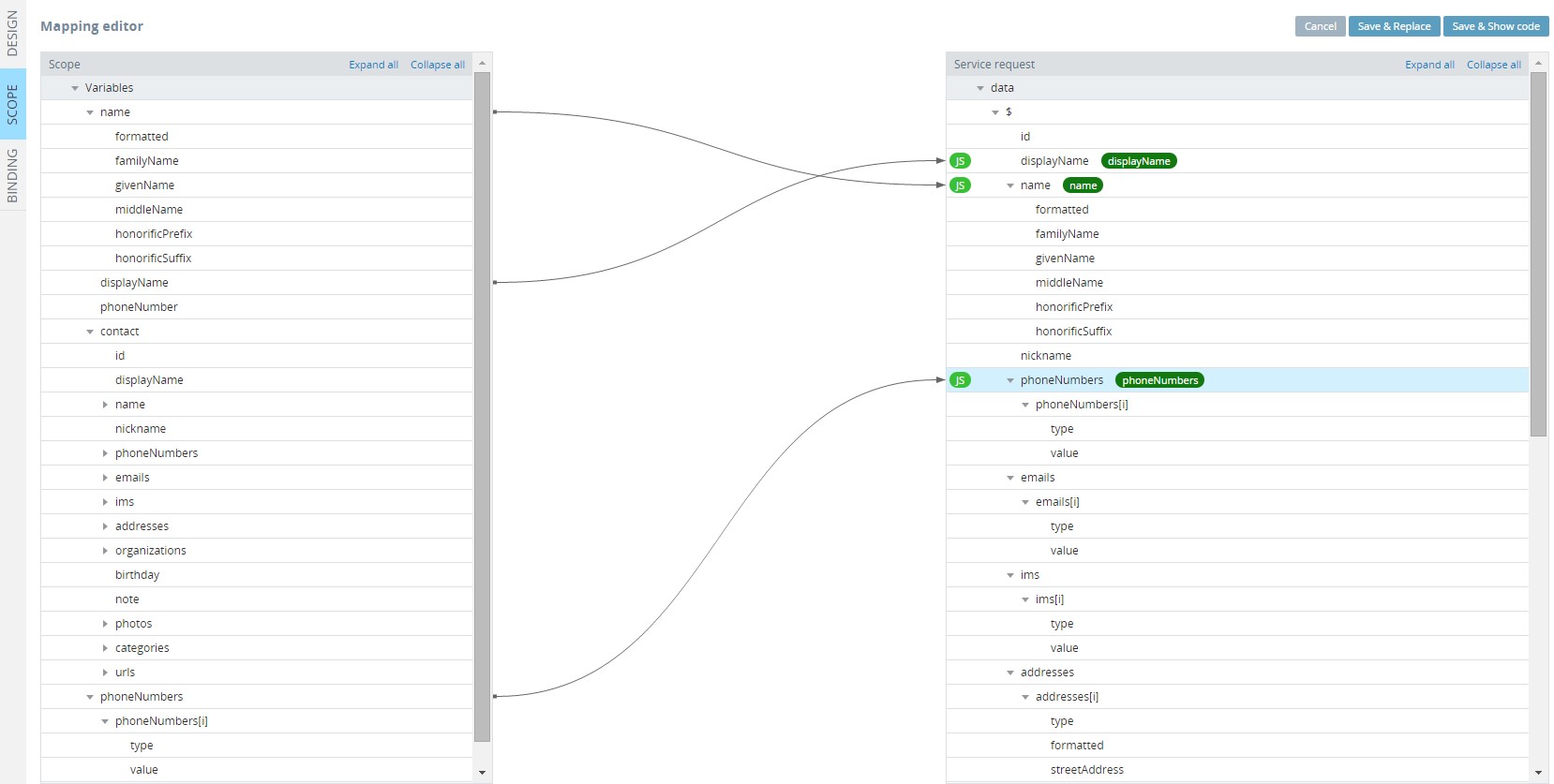
- Second, create the following mapping for the Contact_create service response:
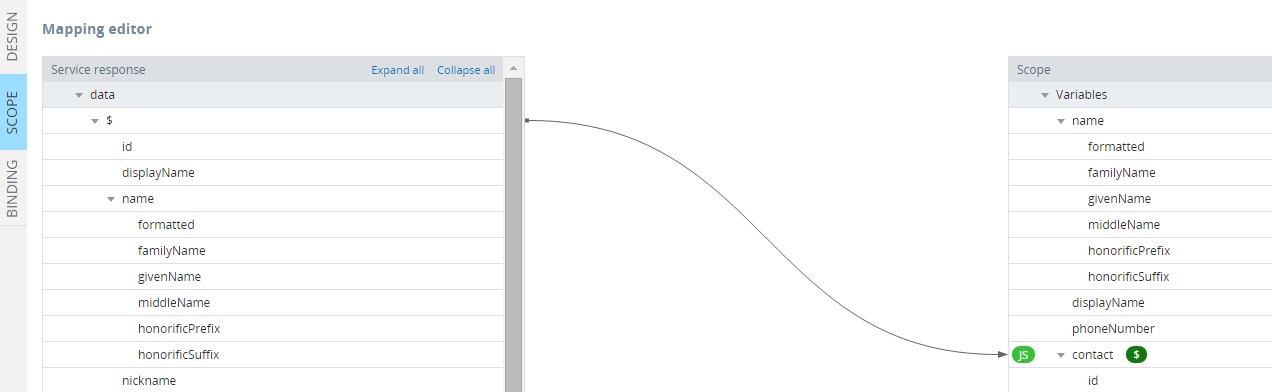
- Now, invoke another service: Contact_save and create the next mapping for the Contact_save service request:
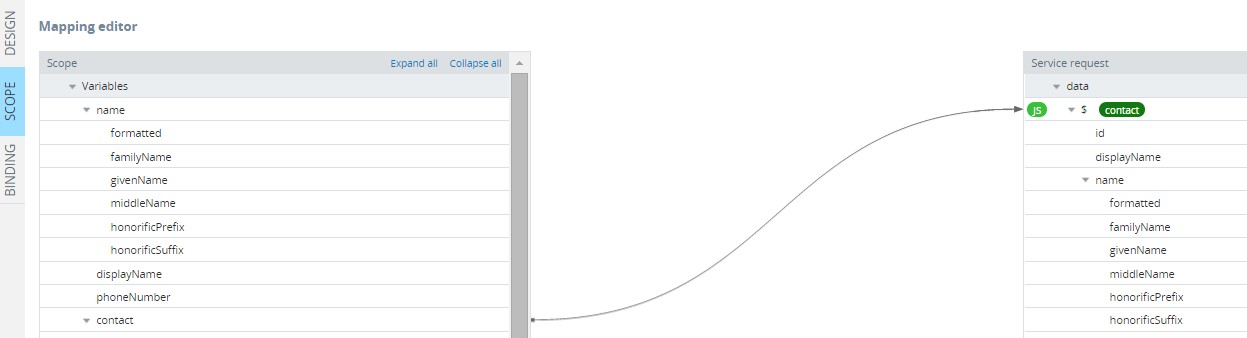
Note
Clicking on the upper service mapping button defines the service request while the lower one defines the service response.
Finally, replace the rest of the code starting from before the last mapping button with the following:
alert("Contact saved");
$scope.clearVars();
},
function(error){
alert("error: " + JSON.stringify(error));
},
function(notify){
});
},
function(error){
},
function(notify){
});
The resulting code should look similar to this:
$scope.phoneNumbers = [];
$scope.phoneNumbers.push(new ContactField('mobile', $scope.phoneNumber, true));
var requestData = {};
requestData = (function mapping8865($scope){
var requestData = {};
requestData = Apperyio.EntityAPI('Contact_create.request.data', undefined, true);
var displayName_scope = $scope.displayName;
var name_scope = $scope.name;
var phoneNumbers_scope = $scope.phoneNumbers;
requestData.displayName = displayName_scope;
requestData.name = name_scope;
requestData.phoneNumbers = phoneNumbers_scope;
return requestData;
})($scope);
Apperyio.get("Contact_create")(requestData).then(
function(success){
(function mapping8778(success, $scope){
var contact_scope = $scope.contact;
contact_scope = success;
$scope.contact = contact_scope;
})(success, $scope);
var requestData = {};
requestData = (function mapping2846($scope){
var requestData = {};
requestData = Apperyio.EntityAPI('Contact_save.request.data', undefined, true);
var contact_scope = $scope.contact;
requestData = contact_scope;
return requestData;
})($scope);
Apperyio.get("Contact_save")(requestData).then(
function(success){ alert("Contact saved");
$scope.clearVars();
},
function(error){
alert("error: " + JSON.stringify(error));
},
function(notify){
});
},
function(error){
},
function(notify){
});
- The last function to edit is clearVars. Add this code to it and save:
$scope.name.givenName = '';
$scope.name.familyName = '';
$scope.displayName = '';
$scope.phoneNumber = '';
Testing Options
Since we’re invoking a native component, this app needs to be tested as a hybrid app or installed on the device:
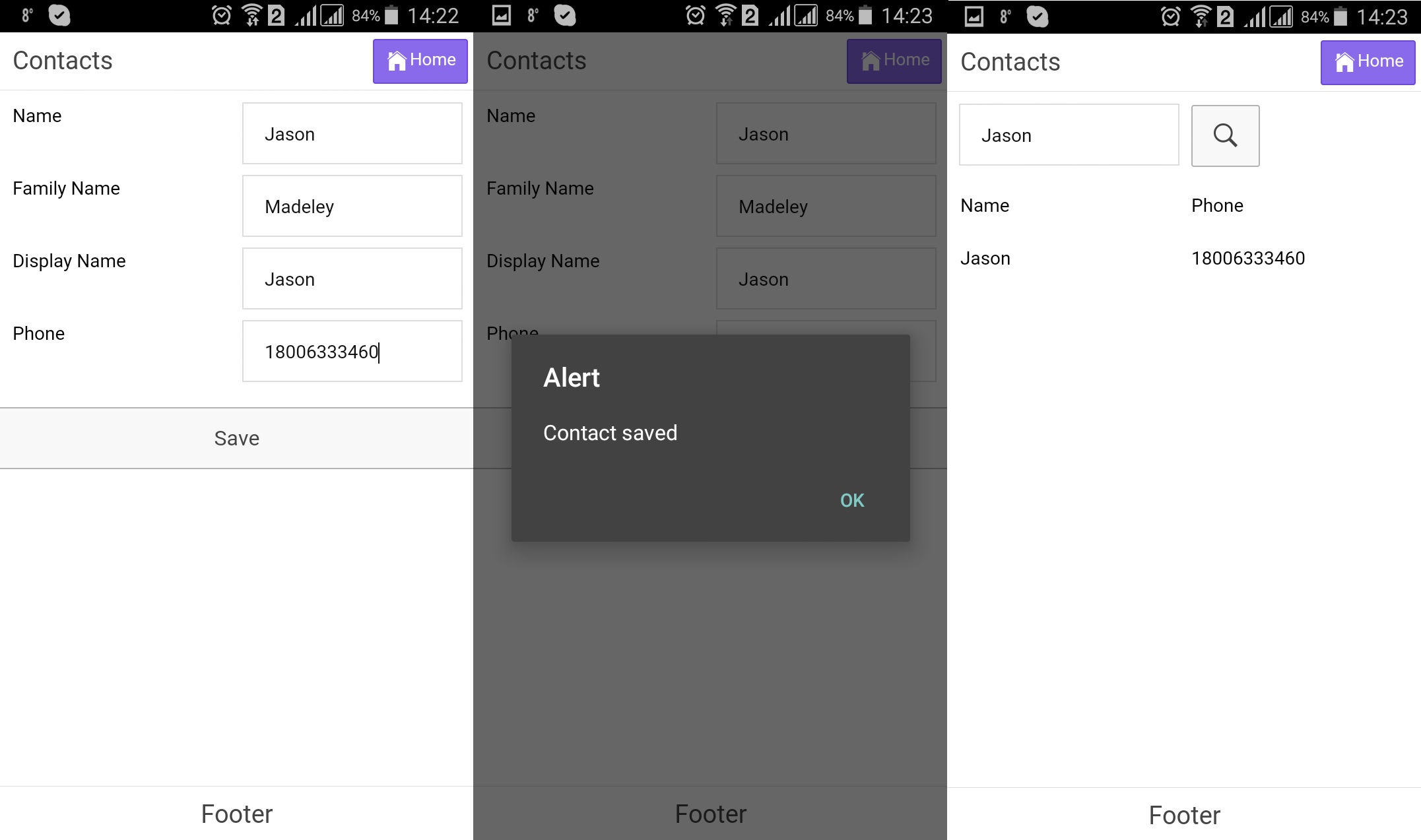
Android
Testing on Android is relatively simple, since you can quickly install any app on your device.
To do it, build the Android binary and install it on your device. When the build is completed, you’ll see a QR code. Scanning the QR code will download the app to your phone. You can also email the app to your device.
iOS
Build the app and install it on your device.
Important Note
Please, note that to export the app for iOS, you will need to upload your distribution certificate and provisioning profile obtained from Apple under the App settings > iOS binary tab.
You can check this document in case you need help with exporting your application for iOS. Here, you will find the document that explains how to manage certificates in Appery.io.