Step 1: Decide what you want your Token to be
In order to create an ERC20 token, you will need the following:
- The Token’s Name
- The Token’s Symbol
- The Token’s Decimal Places
- The Number of Tokens in Circulation
The decimal places is something where things can get tricky. Most tokens have 18 decimal places, meaning that you can have up to .0000000000000000001 tokens.
When creating a token, you will need to be aware of what decimal places you would like to define and how they would fit into the larger picture of your project.
To keep it simple, you can choose 0. But you could choose 1 if you would like people to have half a token, or 18 if you would like it to be ‘standard’.
Step 2: Code the Contract
Here is a contract that you can Copy/Paste to create your ERC20 token.
pragma solidity ^0.4.16;
contract Token {
/// @return total amount of tokens
function totalSupply() constant returns (uint256 supply) {}
/// @param _owner The address from which the balance will be retrieved
/// @return The balance
function balanceOf(address _owner) constant returns (uint256 balance) {}
/// @notice send `_value` token to `_to` from `msg.sender`
/// @param _to The address of the recipient
/// @param _value The amount of token to be transferred
/// @return Whether the transfer was successful or not
function transfer(address _to, uint256 _value) returns (bool success) {}
/// @notice send `_value` token to `_to` from `_from` on the condition it is approved by `_from`
/// @param _from The address of the sender
/// @param _to The address of the recipient
/// @param _value The amount of token to be transferred
/// @return Whether the transfer was successful or not
function transferFrom(address _from, address _to, uint256 _value) returns (bool success) {}
/// @notice `msg.sender` approves `_addr` to spend `_value` tokens
/// @param _spender The address of the account able to transfer the tokens
/// @param _value The amount of wei to be approved for transfer
/// @return Whether the approval was successful or not
function approve(address _spender, uint256 _value) returns (bool success) {}
/// @param _owner The address of the account owning tokens
/// @param _spender The address of the account able to transfer the tokens
/// @return Amount of remaining tokens allowed to spent
function allowance(address _owner, address _spender) constant returns (uint256 remaining) {}
event Transfer(address indexed _from, address indexed _to, uint256 _value);
event Approval(address indexed _owner, address indexed _spender, uint256 _value);
}
contract StandardToken is Token {
function transfer(address _to, uint256 _value) returns (bool success) {
//Default assumes totalSupply can't be over max (2^256 - 1).
//If your token leaves out totalSupply and can issue more tokens as time goes on, you need to check if it doesn't wrap.
//Replace the if with this one instead.
//if (balances[msg.sender] >= _value && balances[_to] + _value > balances[_to]) {
if (balances[msg.sender] >= _value && _value > 0) {
balances[msg.sender] -= _value;
balances[_to] += _value;
Transfer(msg.sender, _to, _value);
return true;
} else { return false; }
}
function transferFrom(address _from, address _to, uint256 _value) returns (bool success) {
//same as above. Replace this line with the following if you want to protect against wrapping uints.
//if (balances[_from] >= _value && allowed[_from][msg.sender] >= _value && balances[_to] + _value > balances[_to]) {
if (balances[_from] >= _value && allowed[_from][msg.sender] >= _value && _value > 0) {
balances[_to] += _value;
balances[_from] -= _value;
allowed[_from][msg.sender] -= _value;
Transfer(_from, _to, _value);
return true;
} else { return false; }
}
function balanceOf(address _owner) constant returns (uint256 balance) {
return balances[_owner];
}
function approve(address _spender, uint256 _value) returns (bool success) {
allowed[msg.sender][_spender] = _value;
Approval(msg.sender, _spender, _value);
return true;
}
function allowance(address _owner, address _spender) constant returns (uint256 remaining) {
return allowed[_owner][_spender];
}
mapping (address => uint256) balances;
mapping (address => mapping (address => uint256)) allowed;
uint256 public totalSupply;
}
//name this contract whatever you'd like
contract ERC20Token is StandardToken {
function () {
//if ether is sent to this address, send it back.
throw;
}
/* Public variables of the token */
/*
NOTE:
The following variables are OPTIONAL vanities. One does not have to include them.
They allow one to customise the token contract & in no way influences the core functionality.
Some wallets/interfaces might not even bother to look at this information.
*/
string public name; //fancy name: eg Simon Bucks
uint8 public decimals; //How many decimals to show. ie. There could 1000 base units with 3 decimals. Meaning 0.980 SBX = 980 base units. It's like comparing 1 wei to 1 ether.
string public symbol; //An identifier: eg SBX
string public version = 'H1.0'; //human 0.1 standard. Just an arbitrary versioning scheme.
//
// CHANGE THESE VALUES FOR YOUR TOKEN
//
//make sure this function name matches the contract name above. So if you're token is called TutorialToken, make sure the //contract name above is also TutorialToken instead of ERC20Token
function ERC20Token(
) {
balances[msg.sender] = NUMBER_OF_TOKENS_HERE; // Give the creator all initial tokens (100000 for example)
totalSupply = NUMBER_OF_TOKENS_HERE; // Update total supply (100000 for example)
name = "NAME OF YOUR TOKEN HERE"; // Set the name for display purposes
decimals = 0; // Amount of decimals for display purposes
symbol = "SYM"; // Set the symbol for display purposes
}
/* Approves and then calls the receiving contract */
function approveAndCall(address _spender, uint256 _value, bytes _extraData) returns (bool success) {
allowed[msg.sender][_spender] = _value;
Approval(msg.sender, _spender, _value);
//call the receiveApproval function on the contract you want to be notified. This crafts the function signature manually so one doesn't have to include a contract in here just for this.
//receiveApproval(address _from, uint256 _value, address _tokenContract, bytes _extraData)
//it is assumed that when does this that the call *should* succeed, otherwise one would use vanilla approve instead.
if(!_spender.call(bytes4(bytes32(sha3("receiveApproval(address,uint256,address,bytes)"))), msg.sender, _value, this, _extraData)) { throw; }
return true;
}
}
You are going to want to replace everything in the area where it says CHANGE THESE VARIABLES FOR YOUR TOKEN.
So that means, and you change:
- The token name
- The token symbol (usually not more than 4 characters)
- The token decimal places
- How much you as the owner want to start off with
- The number of tokens in circulation (to keep things basic, make this is the same amount as the owner supply)
Some things to keep in mind:
The supply that you set for the token is correlated to the number of decimal places defined.
For example, if you want a token that has 0 decimal places to have 100 tokens, then the supply would be 100.But if you have a token with 18 decimal places and you need 100 of them, then the supply would be 100000000000000000000 (18 zeros added to the amount).
Step 3: Test the Token on the TestNet
Next, we are going to deploy the contract to the Test Net to check if it works.
First, if you don’t have it already, download MetaMask. They have an easy-to-use interface to test this.
Once you have installed MetaMask, make sure that you are logged in and setup on the Ropsten test network. If you click on the top left where it says Main Ethereum Network you can change it to Ropsten.
To confirm, the top of your MetaMask window should look like this:
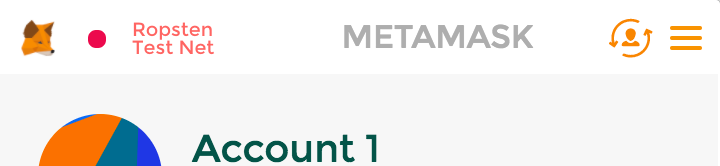
This Wallet is going to be the ‘Owner’ of the contract, so keep it safe, please! If you would not like it to be Metamask, you can use Mist or MyEtherWallet to create contracts as well.
Now, head to the Solidity Remix Compiler - it is an online compiler that allows publishing a Smart Contract straight to the blockchain.
Copy/Paste the source of the contract you have just modified into the main window. It will look something like this:
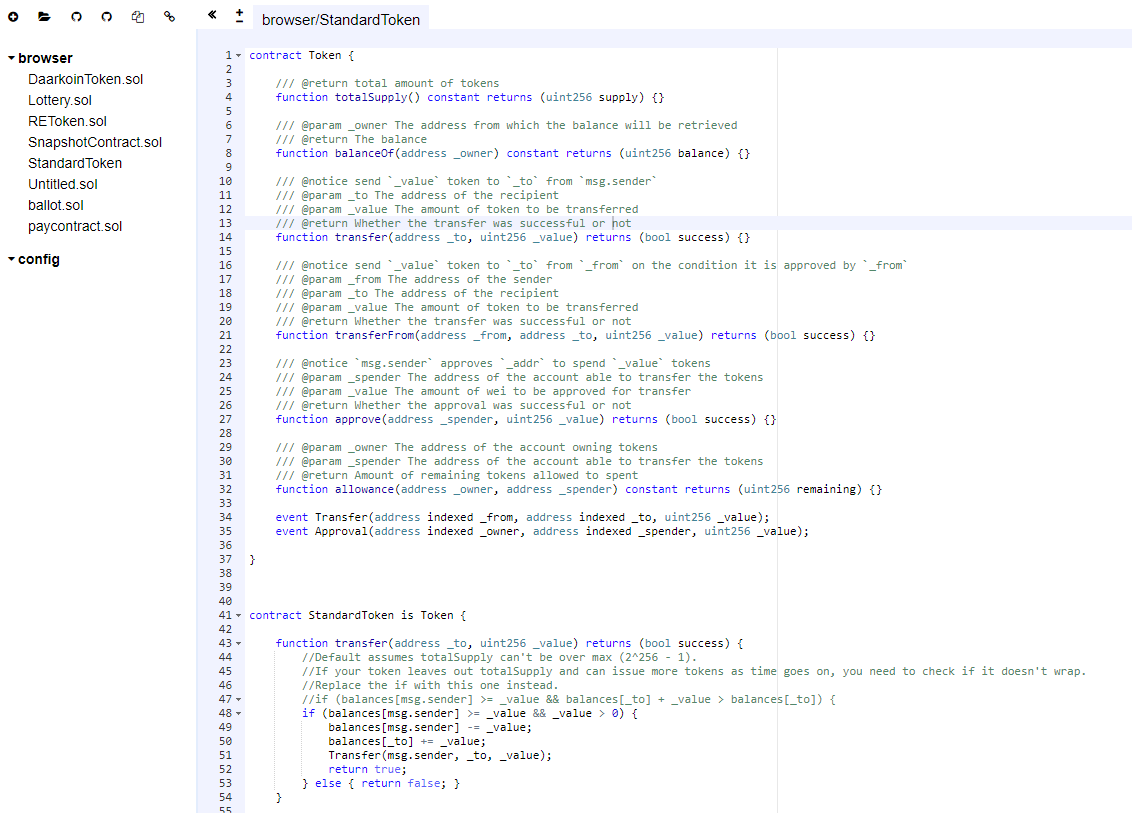
Click on Start to compile button for compile source code.
To deploy contract, press the deploy button on the Run tab.
MetaMask will pop up asking you to hit Submit to pay for the transaction.
Remember, this is the Ropsten test net, so it is not a real Ether. Just to be sure, you can double check to make sure you are on the test network in MetaMask before you submit.
When you hit Submit, it will say Contract Pending in MetaMask. When it is ready, click the Date and it will bring up the transaction in EtherScan. Like this
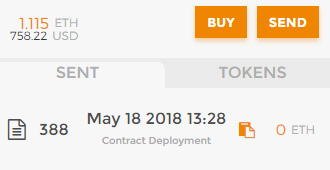
If you click the date, it will open the following screen:
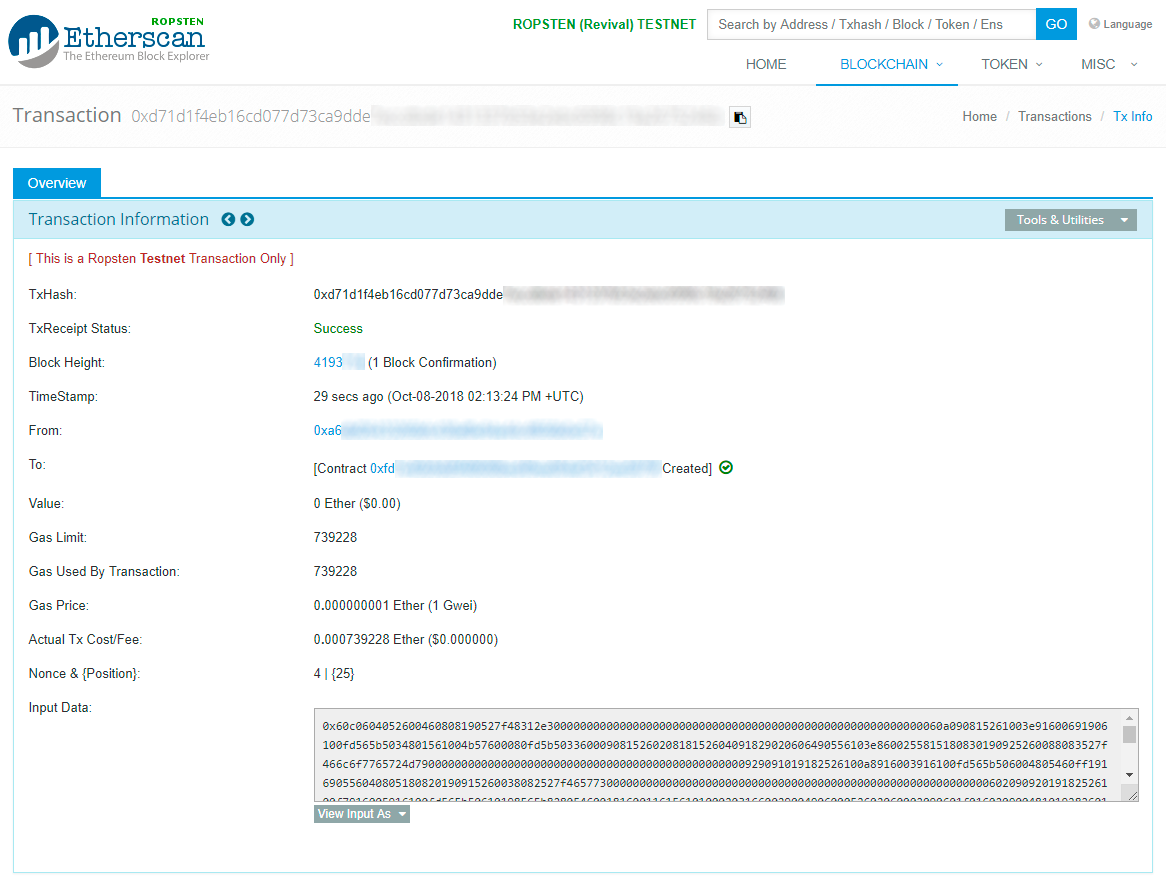
Step 4. Watch the Custom Token
Now, let’s see if it actually created the tokens and sent them.

Copy the Contract Address listed in the Transaction information part (see the screenshot above).
In this particular case, it is 0xfdxxxxxxxxxxxxxxxx.
Add that to the MetaMask Tokens tab:
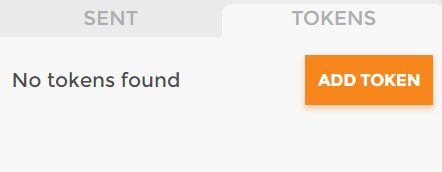
Click the ADD TOKEN button and paste it in there - it will automatically insert the information of the token, like below:
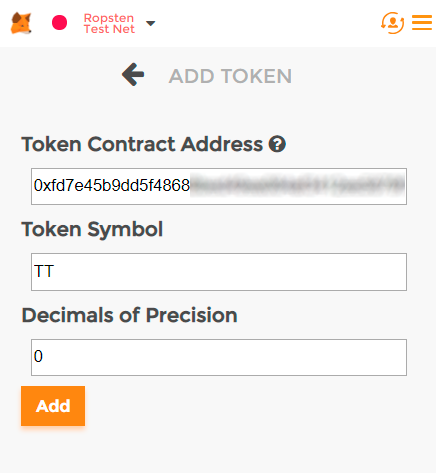
Step 5. Verify the Source Code
This is going to be important for various reasons, mainly because of verifying the validity of your token to the public.
It doesn’t technically matter, and your token will still be usable if you don’t do it, but it’s a good practice to verify it so that people know that you’re not doing anything shady.
When you’re on the Transaction screen from the previous step, click where it says Contract xxxxxx Created in the To: field. That is the Contract we have just published.
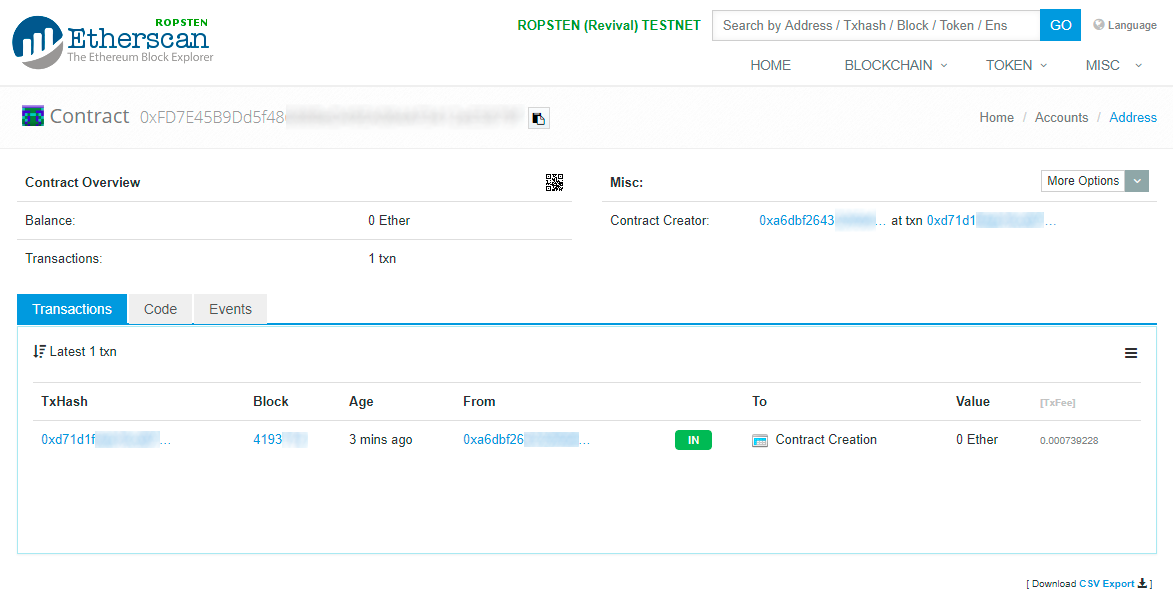
Then click the Code tab.
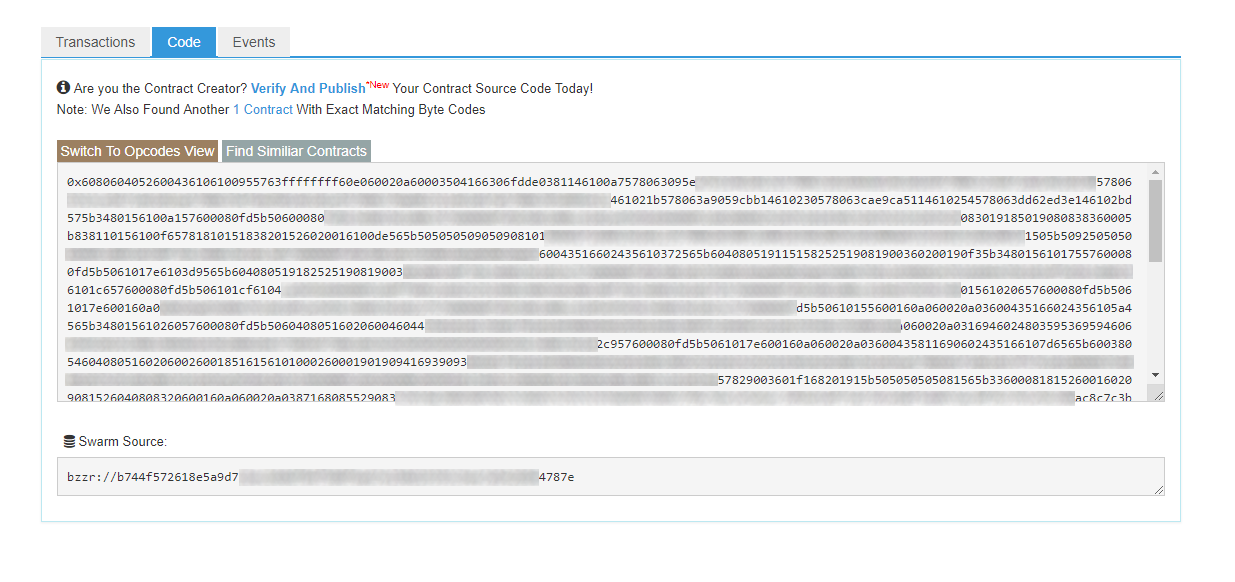
Hit Verify and Publish. That will bring you to this screen:
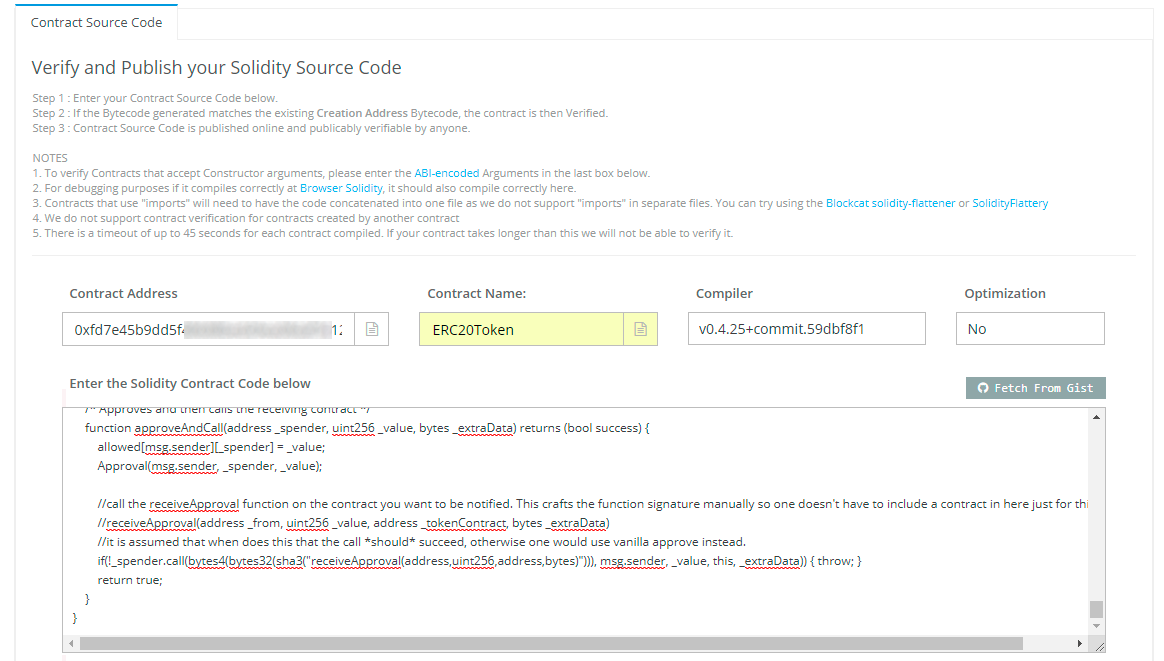
Here’s where you NEED to have the right settings.
The Contract Address will already be filled in.
For Contract Name, make sure to put the name of the function that you modified for your custom token. The default in the code provided above is ERC20Token, but if you rename it, make sure you have added the new name.
For Compiler, select the SAME compiler you used in the Solidity Compiler. Otherwise, you will not be able to verify your source code!
Select No for Optimization.
Then Copy/Paste the code from the compiler right into the Contract Code field (the big one).
Enter the captcha and hit Verify and Publish.
If you did the steps correctly, you will get something like this:

That means it has been verified.
If you go to the Contract address page, you’ll see that Contract Source says Yes and confirms that it is Verified. Like this:

If not, double check your steps, or make a comment in this thread and we’ll make research into what went wrong.
Now, we recommend that you follow the second part of this tutorial.