Using the Geolocation service in an Ionic app
Introduction
This tutorial shows how to create and use the Geolocation service in an AngularJS app.
Want to know more?
If you are interested in how to quickly get the Google Map plug-in up and running in an Appery.io Ionic 5 application capable of identifying and storing location data, check this document.
Important Note!
Note that the option of creating new apps with the Ionic framework was removed but we still support the projects that were created with it earlier.
Ionic 5 Google Maps Tutorial
You can also check our Ionic 5 Google Maps Tutorial, where we demonstrate how to use this component in an Ionic 5 app. As part of the app design, the Geolocation service is added to show current coordinates on the map.
Apache Cordova (PhoneGap)
Apache Cordova (PhoneGap) is automatically included when you create a new project in Appery.io. The Geolocation component used in Appery.io is the Geolocation component from Apache Cordova.
Note
To learn more about the component, and any other settings or options, please see the geolocation plugin documentation page.
Creating App
Design
- Open your Appery.io Bootstrap/AngularJS or Ionic/AngularJS project or create a new one. You will get two default pages: index and Screen1.
- Go to Screen1 and create the following UI consisting of:
- A Button component (configure its Block to True, Text to Get my position, and ng-click to geo());
- Text components: Text = Latitude, {{la}}, Longitude, and {{lo}} respectively.
This is how it should look like:
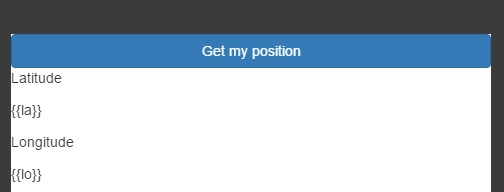
Now, let’s create services and variables and bind them with UI components.
Adding services
- In the Appery.io Visual Builder, go to Project > CREATE NEW > From Plugin.
- In the new window, select Apperyio Geolocation Service and click Import selected plugins.
- In the Project view, a list of related services and a JavaScript file appear.
- After a native service has been added to the app, it can be called. Invoking a native service is very similar to invoking a REST service.
Scope
- First, go to SCOPE and add two variables: la (for latitude) and lo (for longitude).
- To call the added service, on to the SCOPE view of the Screen1 page, add a new function – geo and click Add, opening the function editor.
- Next, in the editor, click the arrow to filter snippets, select Invoke service, and click Insert snippet.
- Delete the text "service_name" in the code and click CTRL+SPACE to get the drop-down with all the available services.
Note
Instead of invoking services in the code editor with subsequent auto completing, you can use a drag and drop feature to invoke services.
- Select Geolocation_currentPosition- Service. After auto-completing, the service is added to the function code and you can click Mapping to map the service to the page.
- By mapping the service response latitude and longitude to the la* *and lo* *variables respectively and click Save & Replace, you add the following code to the function:
var la_scope = $scope["la"];
var lo_scope = $scope["lo"];
la_scope = success.coords.latitude;
lo_scope = success.coords.longitude;
$scope["la"] = la_scope;
$scope["lo"] = lo_scope;
The code must look like:
var requestData = {};
Apperyio.get("Geolocation_currentPosition")(requestData).then(
function(success){
(function mapping5850(success, $scope){
var la_scope = $scope.la;
var lo_scope = $scope.lo;
la_scope = success.coords.latitude;
lo_scope = success.coords.longitude;
$scope.la = la_scope;
$scope.lo = lo_scope;
})(success, $scope);
},
function(error){
},
function(notify){
});
// Function code
Now, let’s modify the app by invoking Geolocation_watchPosition and Geolocation_clearWatch services.
Watching Position
Watching position is a great feature that tracks current location. Let’s add it to the app.
Note
The Geolocation_watchPosition method begins listening for updates to the current geographical location of the device running the client. It is an asynchronous function and returns the device’s current position when a change in position has been detected. When the device has retrieved a new location, the geolocationSuccess callback is invoked with a Position object as the parameter.
- On the Design tab of Screen1, add two buttons:
- The Button component (set Block to True, Text to Watch my position and ng-click to watch()).
- The Button component (set Block to True, Text to Stop watching and ng-click to stop()).
This is how it should look:
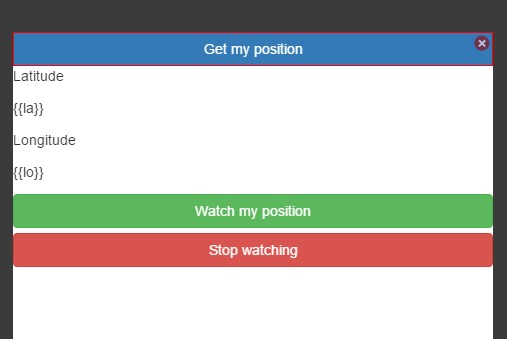
- Now, under SCOPE, create the watch() function that invokes Geolocation_watchPosition service and has the following code:
if ($scope.watchId) return;
var requestData = {};
var def = Apperyio.get("Geolocation_watchPosition")(requestData);
def.then(
function(success){
},
function(error){
},
function(notify){
var la_scope = $scope.la;
var lo_scope = $scope.lo;
la_scope = notify.result.coords.latitude;
lo_scope = notify.result.coords.longitude;
$scope.la = la_scope;
$scope.lo = lo_scope;
});
$scope.watchId = def.watchId;
Note
The notify event is used because you are going to receive coordinates many times. The received data is an object ({result: Geoposition, isSuccess: true} if successful and {result: PositionError, isSuccess: false} if unsuccessful). That is why you should check isSuccess in code.
- Then, create the stop() function that invokes Geolocation_clearWatch service and has the following code:
var requestData = {watchID: $scope.watchId};
Apperyio.get("Geolocation_clearWatch")(requestData);
$scope.watchId = undefined;
Note
The Geolocation_clearWatch method stops watching for updates to the current device’s geographical location by clearing the Geolocation_watchPosition referenced by watchID.
watchId is returned in the deffered object that was created when service invoked. You can obtain it and then kill this watcher. It’s possible to create several watchers in the app.
Call clearWatch() to kill them all.
You are ready to test the app.
Testing App
- Run a test to check how your app will work.
Note
The Location Service of your device must be enabled.
-
Click Get my position to obtain your current location.
-
Watch my position enables tracking your position in real-time. Stop watching button disables this mode.
Note
New coordinates must significantly differ from current ones to be catched. So don’t expect testing this feature when pacing the office.
Android
Testing on Android is relatively simple, since you can quickly install any app on your device.
To do it, build the Android binary and install it on your device. When the build is completed, you’ll see a QR code. Scanning the QR code will download the app to your phone. You can also email the app to your device.
iOS
Build the app and install it on your device.
Important Note
Please, note that to export the app for iOS, you will need to upload your distribution certificate and provisioning profile obtained from Apple under the App settings > iOS binary tab.
You can check this document in case you need help with exporting your application for iOS. Here, you will find the document that explains how to manage certificates in Appery.io.