Using the scope editor.
Introduction
Appery.io provides a visual representation of scope in AngularJS. By going to the SCOPE tab you can add new variables and functions. You can also manage their types.
Scope
There is three sub tabs underthe SCOPE tab: Variables, Split and Functions.
Variables
The variables tab allows to create new scope variables and define their types. Type can be primitive or based on the created Model (Project > Model)
Accessing Index Variables
You can access an index variable on different pages in the app. For example, if you have defined a variable on the index page selectedSpoolTag to the read/write scope variable from the page use $parent.$parent structure with the code below:
$scope.$parent.$parent.selectedSpoolTag = SpoolNumber;
Functions
In a newly created app, the init function is created by default (Type = Scope function).
To add a new function, specify its name and type by selecting from the dropdown:

To specify the function parameters, use the Arguments column. Separate them with a comma to provide multiple arguments.
Function Types
There are three function types to select from:
- Scope function is a simple scope method.
- Self executing functions are the ones invoked automatically as soon as they are defined. They are anonymous and are more useful when used with initialization logic.
- Function are functions that are not bound to the controller scope but exist only inside the controller function.
Dependency Injection
As Appery.io doesn’t provide access to the controller to its users, the Dependency Injection option is implemented with the function Apperyio.get("dependency").
In the Appery.io builder, there are only two objects injected into the controller by default: $scope and Apperyio.
The flow is as follows: in case you need to inject, for example, $timeout into the controller, use the following syntax:
var $timeout = Apperyio.get("$timeout")
Further Reading
Read more on Dependency Injection here.
Scope Variables in Ionic
In Ionic pages with content, if a user binds the variables with the UI components working via ng-model, the two-way data-binding won’t work properly in the screens SCOPE. This is due to the fact, that the screen Content has its own child scope, so when defining the screen UI components mentioned above, be sure to pass the scope values through the object.
e.g., If you want to bind the name variable with some component:
- Under Project > Model, create a model User (Type = Object) with a nesting name (Type = String) parameter.
- Switch to SCOPE and add a variable user with Type = User.
- Lastly, in the DESIGN view define the needed UI component with the ng-model = user.name.
As a result, now user.name is correctly binded.
Further Reading
To learn more about child scope, please check this link.
Autocomplete in Scope Editor
The intelligent autocomplete mechanism is available in code editor. To access the autocompletion window press Ctrl+Space or simply type . symbol after object’s name. Autocomplete provides completion for:
- Function names, JS keywords and code snippets.
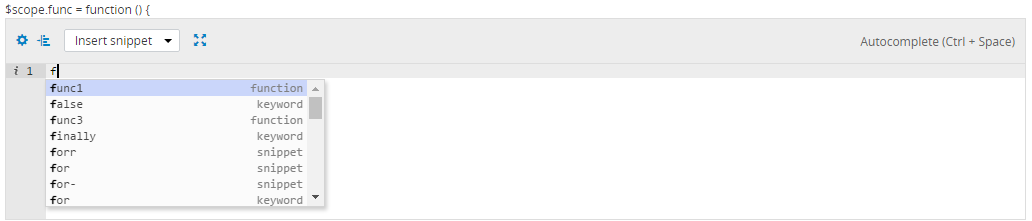
Function names, JS keywords and code snippets.
- Scope variables and functions.

Scope variables and functions.
- Scope variable’s properties.

Scope variable’s properties.
- Apperyio methods and properties.
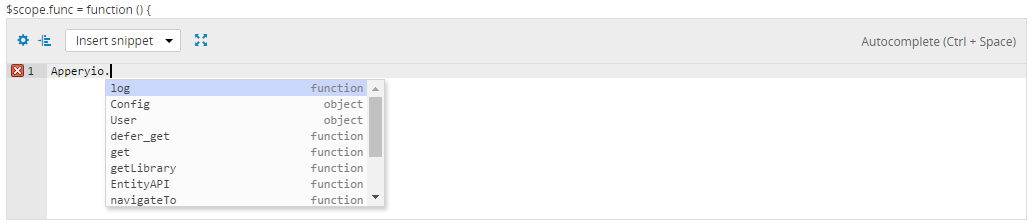
Apperyio methods and properties.
- Apperyio snippet shortcuts
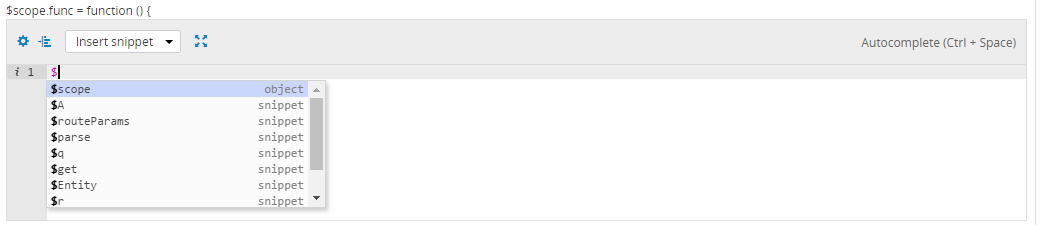
Apperyio snippet
Code Snippets
A snippet is a small section of reusable code that is inserted on an appropriate button click. To make using them easier most snippets have placeholders where you can input data to adjust them. These places are highlighted with a grey background. When the snippet is first added, the cursor will automatically move to the first placeholder. Press Tab to move between the snippet placeholders.
Invoke service
With this snippet, you can add an Appery.io service invocation to the function.
var requestData = {};
// onbeforesend mapping button allows to bind scope variable to service request data
Apperyio.get("service_name")(requestData).then(
function(success){
// onsuccess mapping button allows to bind service response data to scope variable in a case of successful result
},
function(error){
// In the case of error, result may be handled with custom code here
},
function(notify){
// In the case of service notification.
});
service_name – service name to execute. You can also use Ctrl + Space for autocomplete.
Tip
Drag and drop service from the project tree into the SCOPE function field
Navigate To Page
With this snippet, navigation to any page can be added to the scope function, but pages must have appropriate routes.
Apperyio.navigateTo("routeName", {});
routeName – route name to navigate.
{} - hash to be sent as parameters for route
Tip:
Drag and drop page from the project tree into the SCOPE function field
Invoke Scope Refresh
This snippet is used to execute an expression in AngularJS from outside of the AngularJS framework (for example, from browser DOM events, setTimeout, XHR or third party libraries).
$scope.$apply();
Open Modal Page
This snippet is used to adjust, open, and work with a modal. The snippets are different for Ionic and Bootstrap projects.
Bootstrap
$scope.type = newType;
Apperyio.get('Modals').loadModal(modal_name).then(
function(modal){
modal.scope.current_item = {
// Set input object for send to modal window
// Value: $ope.itemFromModal
};
modal.scope.modalInstance = modal.open({
// Optional settings:
size: '', /* 'lg' | 'sm' */
backdrop: true, /* true | false | 'static' */
keyboard: true, /* true | false */
backdropClass: '', /* backdropClass name */
windowClass: '', /* additional CSS class(es) to be added to a modal window template */
windowTemplateUrl: '' /* a path to a template overriding modal's window template */
});
modal.scope.modalInstance.result.then(
function(current_item){
// Custom implementation with modal response with current_item
},
function(error){
console.log(error);
}
);
});
Ionic
Read more information about Ionic modal here.
var modalOptions = { // About Ionic Modal: https://devcenter.appery.io/documentation/angularjs/screens/#Modal_screen
animation: 'slide-in-up', // The animation to show & hide with
focusFirstInput: false, // Whether to autofocus the first input of the modal when shown
backdropClickToClose: true, // Whether to close the modal on clicking the backdrop
hardwareBackButtonClose: true // Whether the modal can be closed using the hardware back button on Android and similar devices
};
Apperyio.get('Modals').loadModal(modal_name).then(
function(modalInstance) {
modalInstance.open(modalOptions).then(function(modal) {
modal.scope.modal = modal;
modal.show();
});
},
function(error){
console.log(error);
});
Tip
Drag & drop the modal page from the project tree into the SCOPE function field.
Init Push Handler
This snippet allows subscribing to a push notification init event.
Push Notification Handler
This snippet allows subscribing to a push notification event.
document.addEventListener("push-notification", function() {
});
Invoke REST API ($http)
The Invoke REST API ($http) is a wrapper for $http. All REST services created within the builder use this feature to create AJAX requests. Invoke the returned function without arguments to receive an object that creates AJAX requests.
The snippet has the following code:
Apperyio.get("REST")()
.setDefaults( options )
.execute( options ).then(
function(success){
},
function(error){
},
function(notify){
});
Options
Basic settings are the same as standard settings for the AngularJS $http service.
- URL (String) – Absolute or relative URL of the resource that is being requested.
- Method (String) – HTTP method (e.g. GET, POST, etc)
- Headers (Object) – Map of strings or functions which return strings representing HTTP headers to send to the server. If the return value of a function is null, the header will not be sent.
- Params (String or Object that contain Strings) – Map of strings or objects which will be turned to ?key1=value1&key2=value2 after the URL. If the value is not a string, it will be converted to JSON.
- Data (String or Object) – Data to be sent as the request message data.
The following additional settings are available:
- Echo (String) – If echo mode is on (property exists), then the service will return an echo value instead of the REST response.
- aio_config (Object that contains Strings) – Appery.io configuration object:
- requestType (String) – Request type (xml, x-www-form-urlencoded)
- responseType (String) – Response type (xml, json, jsonp)
Additional service settings may be needed for conversion from XML to JSON, objects to form data, etc.
The echo property allows you to emulate a service invocation without running the code.
Settings And Invoking
If this parameter exists in the settings dictionary the request doesn’t run.
This property must be deleted from the settings dictionary before publishing the app.
The function setDefaults( default_options ) sets default service settings, and returns a service instance that can be saved in a variable to use several times.
The function execute( /options_for_current_request/ ) can receive a dictionary with settings that can override default settings (described in setDefaults), but only for this request. Invoking a function without parameters will run a request with default settings. A Promise object will be returned.
Service Name
This service has a name REST, so it isn’t a template for some different name.
Shortcuts
Shortcuts are used to quickly add an expression to a function. To use shortcuts, type the appropriate characters and press Ctrl+Space (Cmd+Space) for autocomplete. Shortcuts will begin immediately after changed to the corresponding code.
$Cg to get a variable from config:
Apperyio.Config.get( expression/*, default*/ );
$Ca to add a variable to config:
Apperyio.Config.add( expression, value );
$u to generate a route URL. Use the options parameter to pass route settings:
Apperyio.url( route_name /*, options*/ );
If route_one in application routing is equal to http://somedomain.com/route/path/:id/:value/done/ then:
Apperyio.url( 'route_one', {id: 10, value: 4} );
Will return http://somedomain.com/route/path/10/4/done/
$Entity to reproduce the specified model structure in the object or part of the object.
Apperyio.EntityAPI( model /*, defaults*/ );
$get to get an AngularJS service or other AngularJS service used in the application:
Apperyio.get( service_name );
$q to get a deferred object via the $q service:
var deferred = Apperyio.get( '$q' ).defer();
$parse to convert an AngularJS expression into a function:
var getter = Apperyio.get( '$parse' )( 'expression' );
$routeParams to get route parameters:
var $routeParams = Apperyio.get( "$routeParams" );
More Help Resources
More helpful resources are links to our blog, and to our YouTube channel and other web sites.