Setting up routing (navigation) for an Ionic app.
Introduction
One of the core AngularJS features is Routing, which is used to build a single page app with multiple views. Using Routing to load different parts of the app helps logically divide the app and make it more manageable.
Routing is needed to show different content depending on what route is chosen. It sets the page content to be displayed in the ng-view component of the index page.
Using Routing
When creating a new page you are suggested to create a navigation route for it:
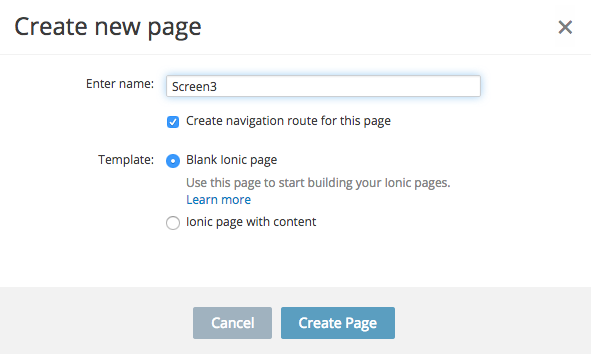
New page with navigation.
Once a page is created, go to Project > Routing to see the newly created routing:
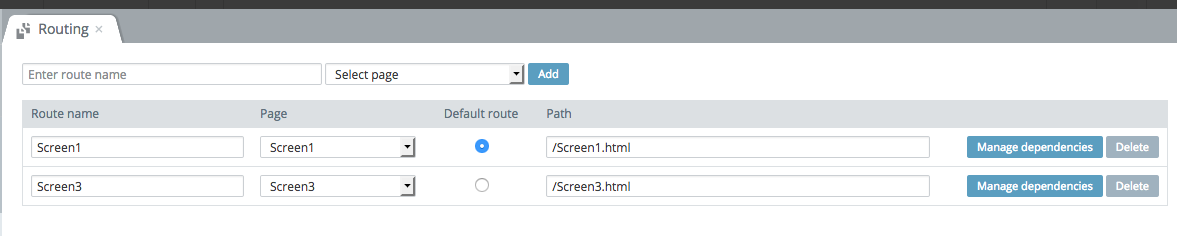
Ionic Routing.
It's also simple to add a route manually. On the Routing view:
- Enter the route name.
- Select the page (where to navigate).
- Click Add to add this routing rule.
Make sure a Default route is set. The Default route shows the first page that will be shown when the app loads.
Navigating to a non-existing route will also redirect you to a default screen. Several routes can lead to the same screen but paths must be different.
Duplicate Paths
Routing should not contain duplicated paths. Unique paths are recommended to ensure your app works correctly.
When renaming a page, you can choose whether to change paths of the corresponding routes.
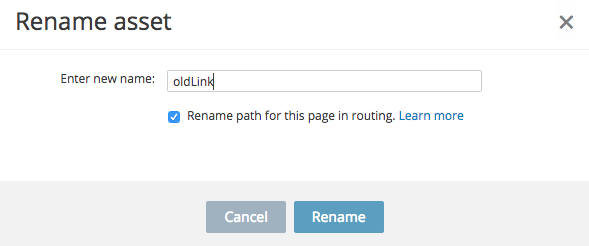
Renaming screen and route.
You can add a dependency for each route by clicking the Manage dependencies button. Note, that you should enable this dependency in the Dependency Manager beforehand.
How Routing Works
To build an app that has multiple views, an AngularJS module called ngRoute is used.
AngularJS uses $routeProvider to handle all of the magic required to get a new file and inject it into layout.
ng-view is an AngularJS directive that will include the template of the current route (/home, /about, or /contact) in the main layout file. In plain words, it takes the file you want based on the route and injects it into your main layout (index.html). In Routing, you set where to place the rendered pages.
Further Information
Read more about passing parameters in URL here.
Using a Directive to Navigate
Navigating to a page is as simple as using the built-in navigate-to directive and setting its value to an existing route:
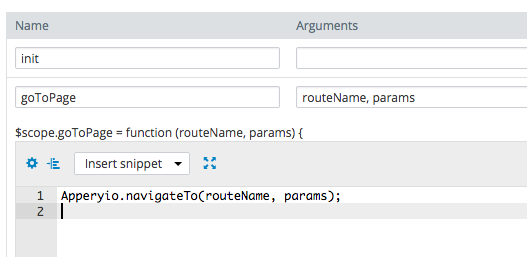
Using navigate-to directive.
Using JavaScript API to Navigate
To navigate using JavaScript API, create a Scope function called goToPage() with the following code:
Apperyio.navigateTo("Screen2");
Inserting Navigation Code With Code Assist
You can insert Apperyio.navigateTo code using a snippet. Select Navigate to Page snippet from the snippets list.
Navigate to Route with Drag & Drop
Drag the needed page from Project tree and drop it in Scope's function.
For the component which is to invoke the navigation, use the ng-click directive and set it to goToPage() function (also available via code assist).
Passing Parameters
In Routing, set the parameters to pass. The following examples show passing two parameters: userId and productId:
/Screen2.html/:userId/:productId
This is how it looks in the Routing view:
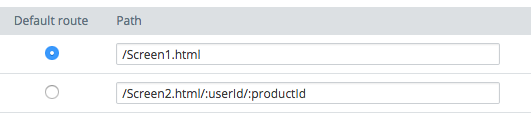
Passing parameters in the URL.
Using Directive
Passing parameters using the special navigate-to[name] directive:
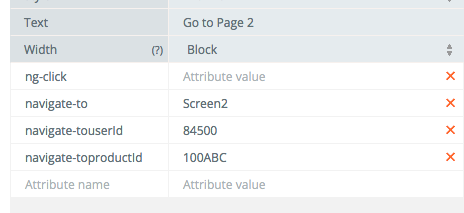
Passing parameters using the built-in directive.
Using JavaScript API
Passing parameters using the Javascript API, update the goToPage function in Scope:
Apperyio.navigateTo("Screen2", {userId: "84500", productId: "100ABC"});
After navigation, you should see the parameters and the updated URL:
http://appery.io/app/view/APP_ID/#/Screen2.html/84500/100ABC
Changing the URL
To see the changed URL click Remove frame button.
Passing in Runtime
To pass navigation parameters in runtime, you can set ng-click to:
goToPage('Screen2', {'userId':'84500', 'productId':'100ABC'});
The goToPage function in Scope is updated with two parameters (routeName, params) in definition and updated code:
Apperyio.navigateTo(routeName, params);
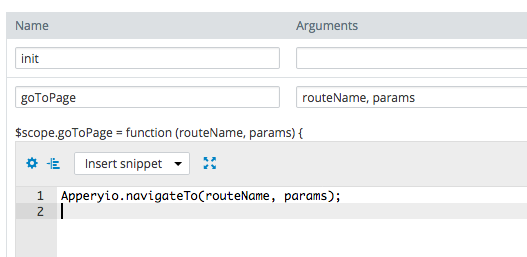
Passing navigation parameters in runtime.
Accessing Parameters
On the page where you navigate to, add this code anywhere you need to access the passed parameters:
var $routeParams = Apperyio.get( "$routeParams" );
$scope.userId = $routeParams.userId;
$scope.productId = $routeParams.productId;
Now userId and productId can be displayed on a page.
Converting Route Name to URL
The routeName2Url() function that returns a route path generated from a route name and dynamic parameters:
Apperyio.routeName2Url( route_name /*, options*/ );
The first parameter is a route name. The second parameter is an object with path variables.
For example, there is a route named route_one that equals to http://somedomain.com/route/path/:id/:value/done/.
The following code will return http://somedomain.com/route/path/10/4/done/:
Apperyio.routeName2Url('route_one', {id:10, value:4});
Using Route Path Template
By default route path template looks like /screen_name.html. You can easily change path by clicking the dropdown list and selecting a new screen. The default path may be changed this way when needed.
You can manually change a path value, including the default part (for example, you change /Screen1.html to /Screen1). From now, automatically inserting a new path when selecting from list is disabled. This is to prevent you from changing your custom path occasionally.
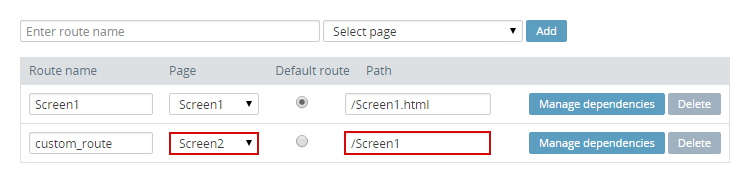
However, if you leave the default part (for example, /screen1.html or /screen1.html/some/path/here) it can be changed as usual.