The tutorial demonstrates how to use ngx-translate third-party internationalization (i18n) library to build a multilingual Ionic 4 app.
For more details on using ngx-translate library, visit this documentation page.
Creating New App
In this tutorial, we will build a simple multilingual app using ngx-translate library.
- From the Apps tab, click Create new app.
- Select Ionic 4 application type and Blank template, enter an app name and click Create. You will see a Start page.
Setting up Internationalization
- On the left side, unfold the Project folder and go to App settings > Components.
- Enable the Internationalization checkbox.
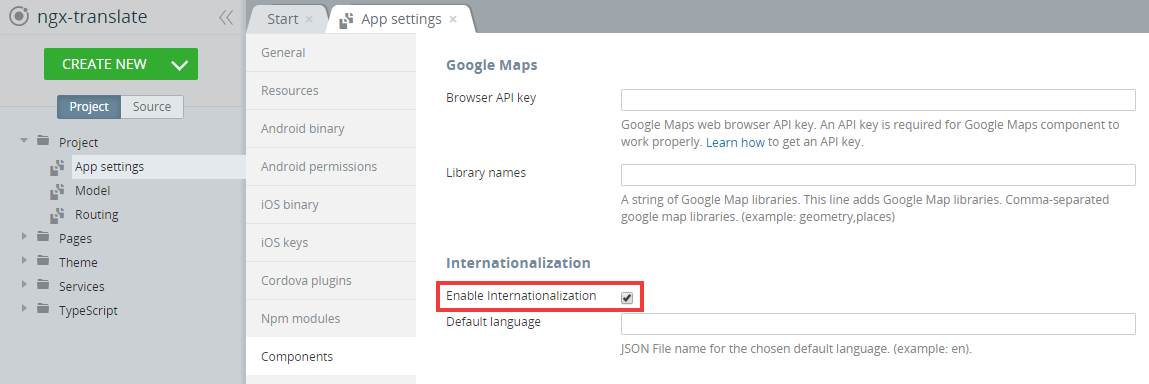
Internationalization enabled
- Then, create the first translation file. Select Create new > TypeScript, from the drop-down, select the Angular i18n language type and enter its name, e.g. en. To confirm, click Create Script.
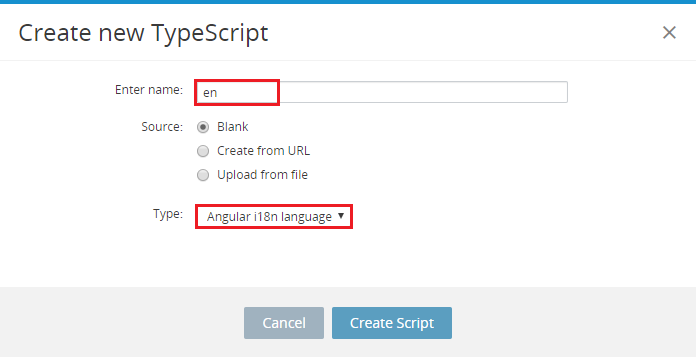
New language TypeScript
- Go back to App settings > Components.
- Set the default language of your app, for example, English. Enter the appropriate JSON File name in the Default Language box. Be sure that your default language and the typescript file have the same names.
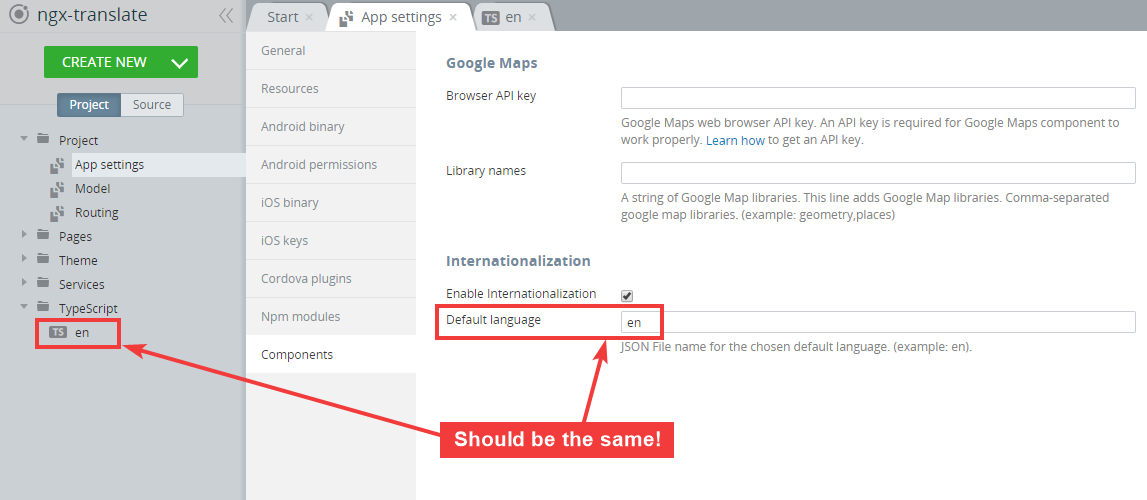
Default language
JSON Translation Files
The only limitation of using the ngx-translate library is that translation cannot be dynamically generated. Therefore, before you can use the library, you need to define translations for all the app texts.
Each language is stored in a separate JSON file. It is represented as a set of key-value pairs, where the key is an identifier used by ngx-translate to find the translation strings, and the value is the actual text in the specified language.
- First, let's create a dictionary for the English translation. On the left, open the previously created en TypeScript and add some JSON dictionary, e.g:
{
"TITLE": "Hello!",
"FOOTER": "made by: Appery",
"Title_2": "Hello Appery.io with ngx-translate!",
"change": "Change language",
"description": "Ooohh... did you just translate this text?",
"data": {
"name": "My name is {{value}} ",
"name2": "My username is <q><i>{{value2}}</i></q>"
},
"select": "select",
"enter_name": "Please enter your name",
"placeholder": "enter name",
"button": "button",
"listItem": "listItem",
"search101": "search",
"radiobutton": "radiobutton"
}
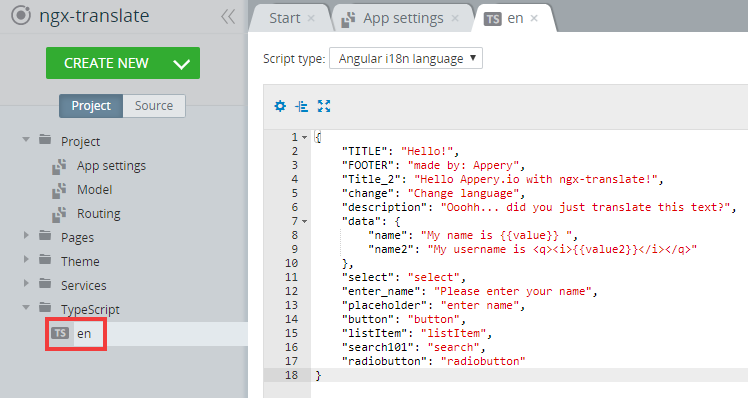
English translation TypeScript
- Similarly, we need to create dictionaries for other languages. For example, let's create es and de JSON files, for Spanish and Deutch translations, respectively:
{
"TITLE": "Hola!",
"FOOTER": "hecho por: Appery",
"Title_2": "Hola Appery.io con ngx-translate!",
"change": "Cambiar de idioma",
"description": "Ooohhh.... ¿acabas de traducir este texto?",
"data": {
"name": "Mi nombre es {{value}} ",
"name2": "Mi nombre de usuario es <q><i>{{value2}}</i></q>"
},
"select": "seleccione",
"enter_name": "Por favor, introduzca su nombre",
"placeholder": "introducir nombre",
"button": "pulsador",
"listItem": "listItem",
"search101": "búsqueda",
"radiobutton": "radiobutton"
}
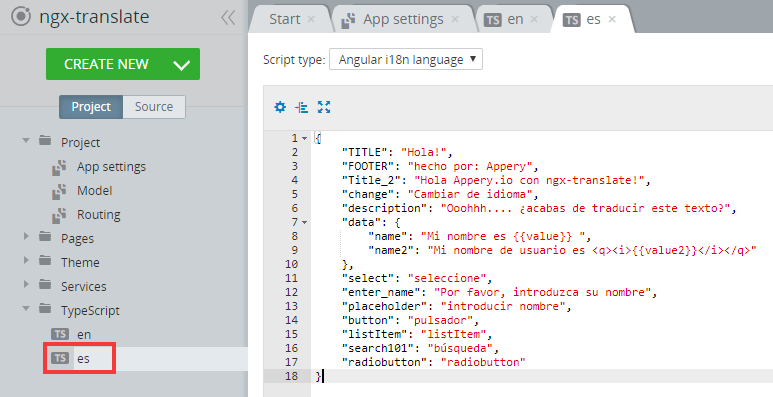
Spanish translation TypeScript
{
"TITLE": "Hallo!",
"FOOTER": "hergestellt von: Appery",
"Title_2": "Hallo Appery.io mit ngx-translate!",
"change": "Sprache ändern",
"description": "Ooohhh.... hast du gerade diesen Text übersetzt?",
"data": {
"name": "Mein Name ist {{value}} ",
"name2": "Mein Benutzername ist <q><i>{{value2}}</i></q>"
},
"select": "auswählen",
"enter_name": "Bitte geben Sie Ihren Namen ein",
"placeholder": "Name eingeben",
"button": "button",
"listItem": "listItem",
"search101": "Suche",
"radiobutton": "radiobutton"
}
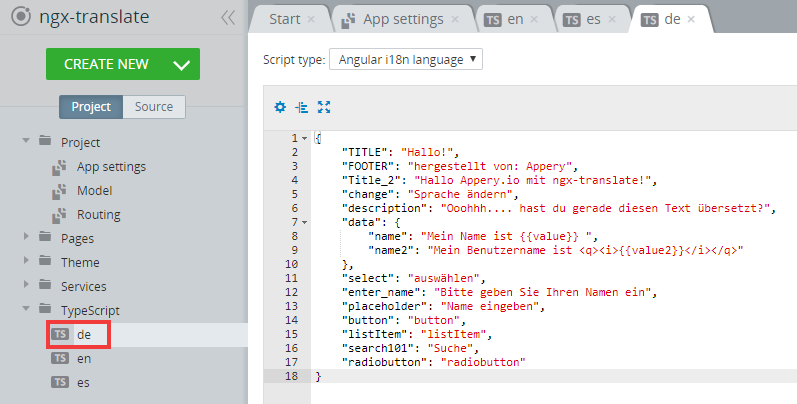
German translation TypeScript
Building UI
Now, we are ready to build the app UI.
- Under the Pages folder, go to Screen1 and open its DESIGN panel.
- Click on the Toolbar title in the app screen and change it to ngx-translate app by editing the Text property on the right menu.
- Drag & drop the Text component to the screen, change its Text property to {{'Title_2' | translate}} and its Container property to h2.
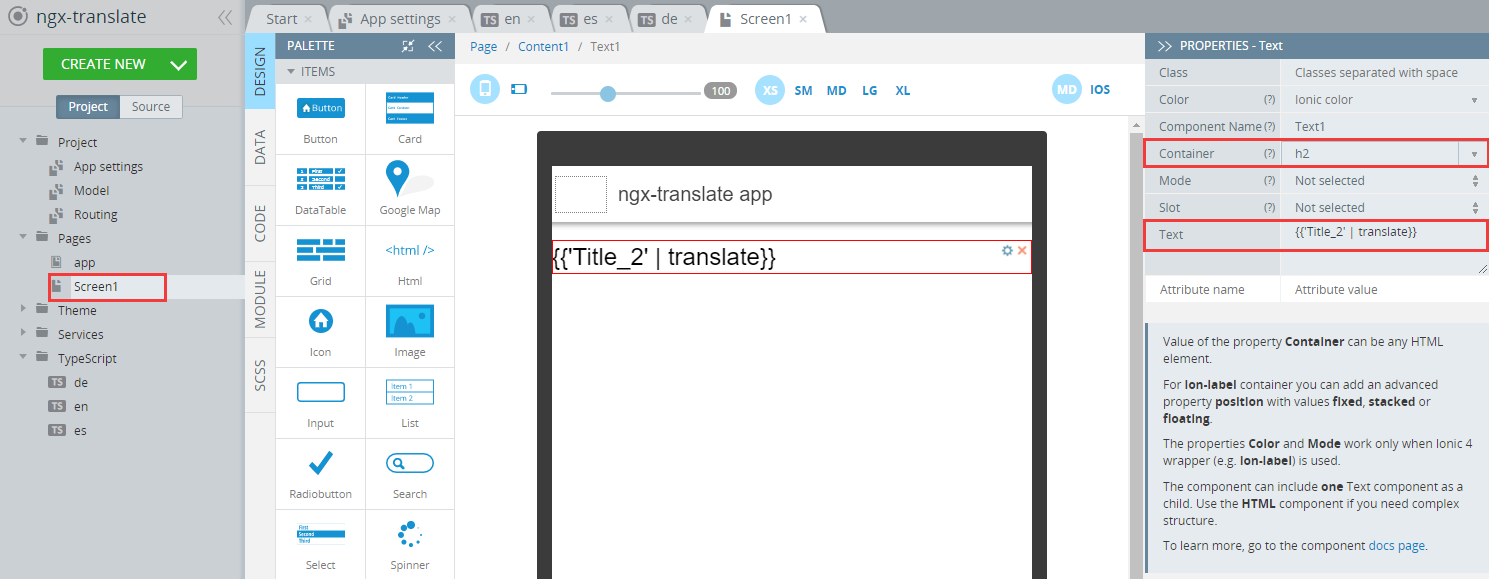
Toolbar Title component
- Drag & drop the Button component to the app toolbar. Change its Text property to EN. Also, change its Slot property to Primary to move it to the right of the toolbar.
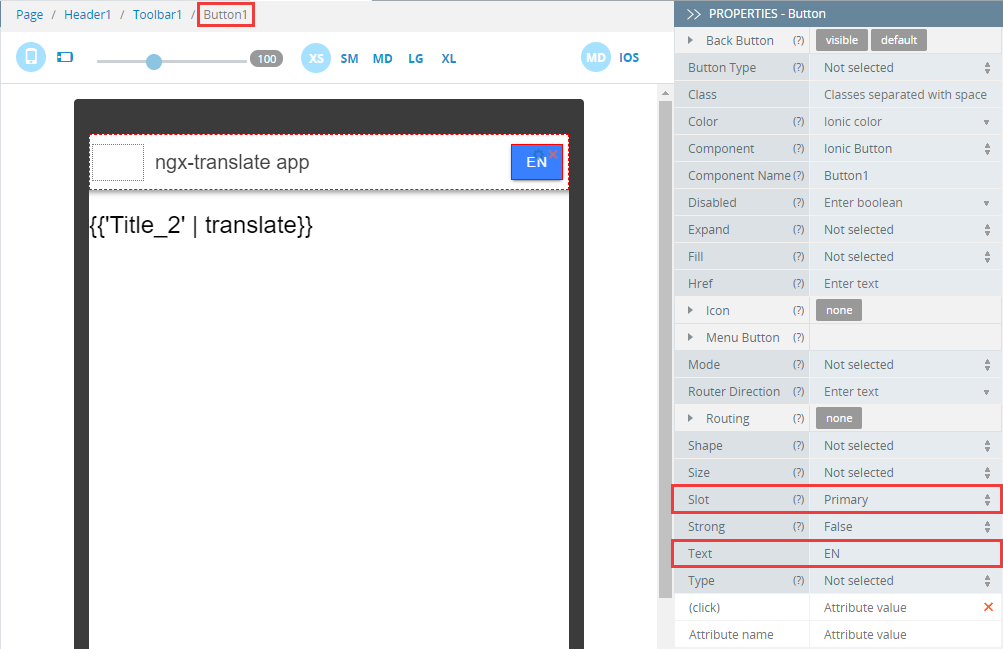
Defining the EN button
- Create two more similar language switching buttons with their Text properties ES and DE, respectively.
The resulting app UI:
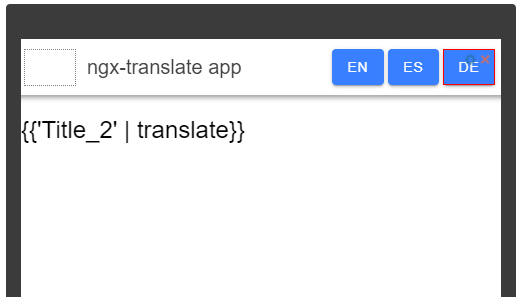
App UI view
Defining Application Logic
- Click the EN button (Button1) and expand the EVENTS tab from the bottom.
- There, for the Button1 component Click event, select Run TypeScript for action.
- In the editor, click Insert snippet and select the i18n set language snippet.
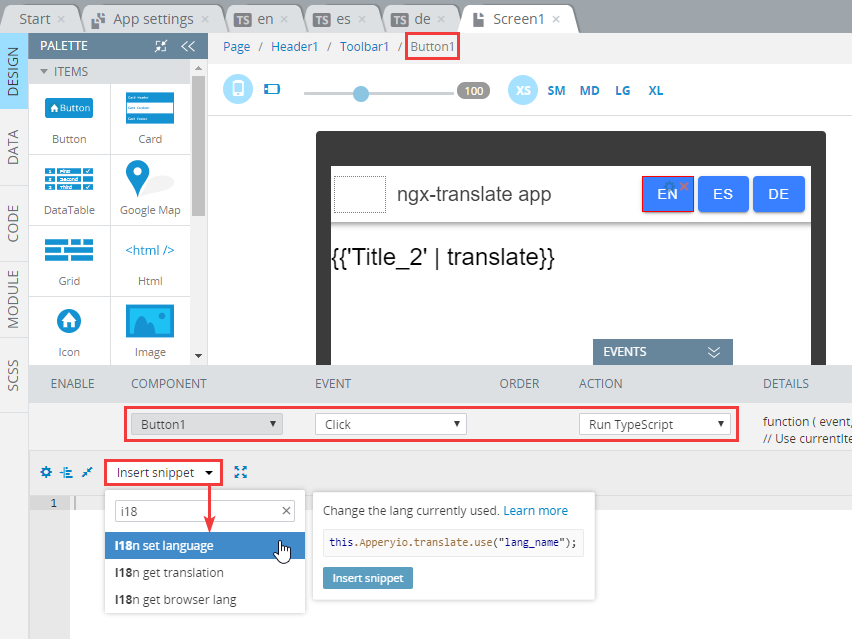
Inserting the i18n set language snippet
- In the code, change the text "lang_name" to "en" and click Save.
this.Apperyio.translate.use("en");
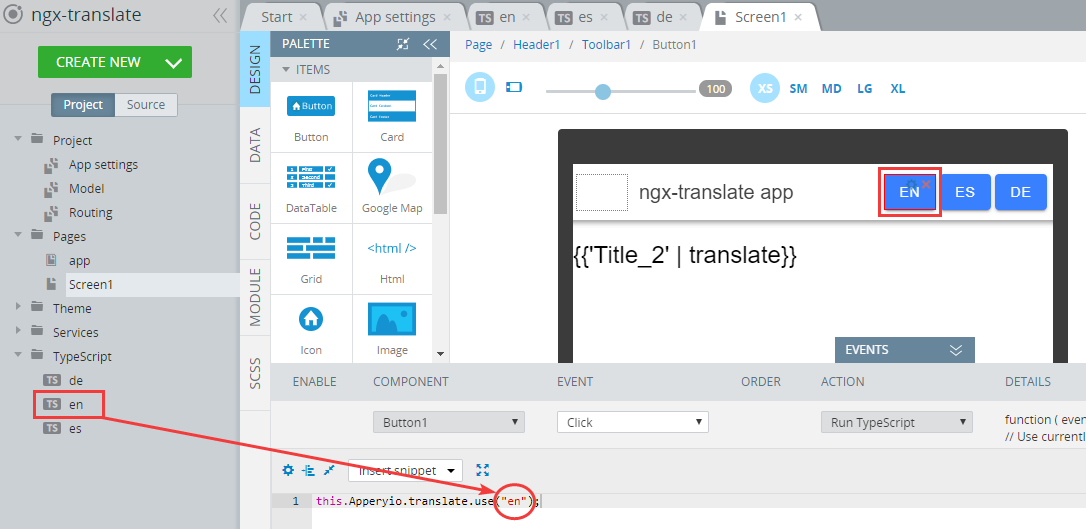
Setting language name in TypeScript
- Repeat steps 1 through 4 to create the similar events for the ES and DE language switching buttons. Use the appropriate translation name for each language:
this.Apperyio.translate.use("de");
this.Apperyio.translate.use("es");
- Click the Save button to save all the changes.
App Testing
Now, you are ready to test your app. Сlick the TEST button in the App Builder to launch your app in the browser.
When the app starts, click the language buttons in the toolbar. Make sure that the app language switches on click:
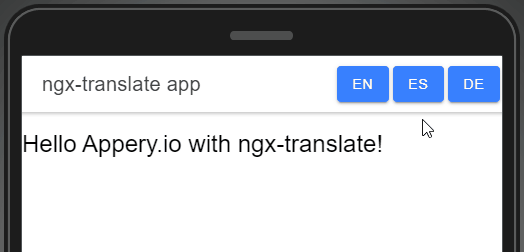
App in action
More Details
We can also use the Translate service with parameters. They are passed as an object, and the keys can be used in the translation strings. Let's give it a try.
- Open the Screen1 CODE panel.
- Here, create three variables:
- variable name of String type with '' (empty string) default value
- variable params of Any type with { value2: 'myusername' } default value
- variable params1 of Any type with { value: 'John' } default value
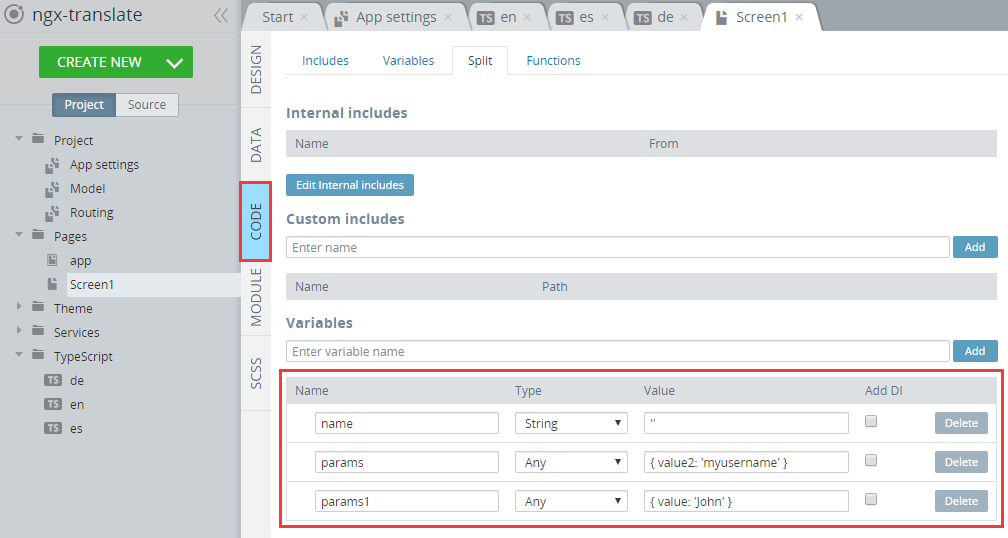
App variables
- Switch to the DESIGN panel.
- Drag & drop the Text component to the screen, change its Container property to h3, and its Text property to the following value:
{{ 'data.name' | translate:{value:'Andreas'} }}
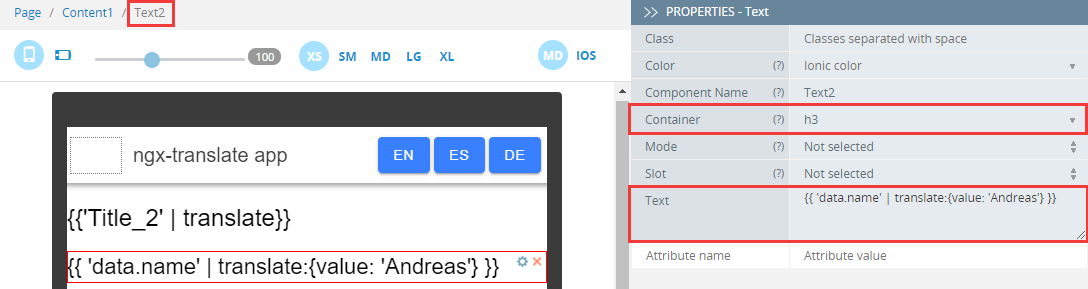
Defining Text2 component
- Drag & drop another Text component to the screen, change its Container property to p, and its Text property to the following value:
{{ 'data.name' | translate:{value: this.name} }}
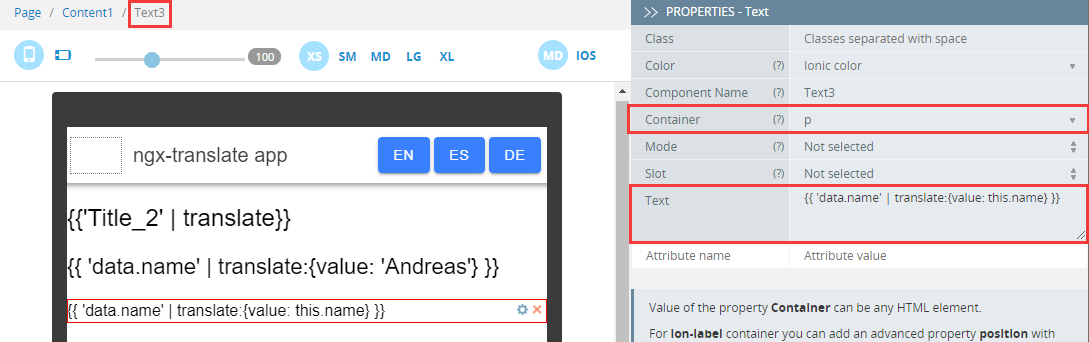
Text3 component parameters
- Under the Text component that we've just created, drag & drop the Input component. Set its properties to the following values:
- [(ngModel)] property to name
- Placeholder property to {{ 'placeholder' | translate }}
- Text property (unfold Label) to {{ 'enter_name' | translate }}:
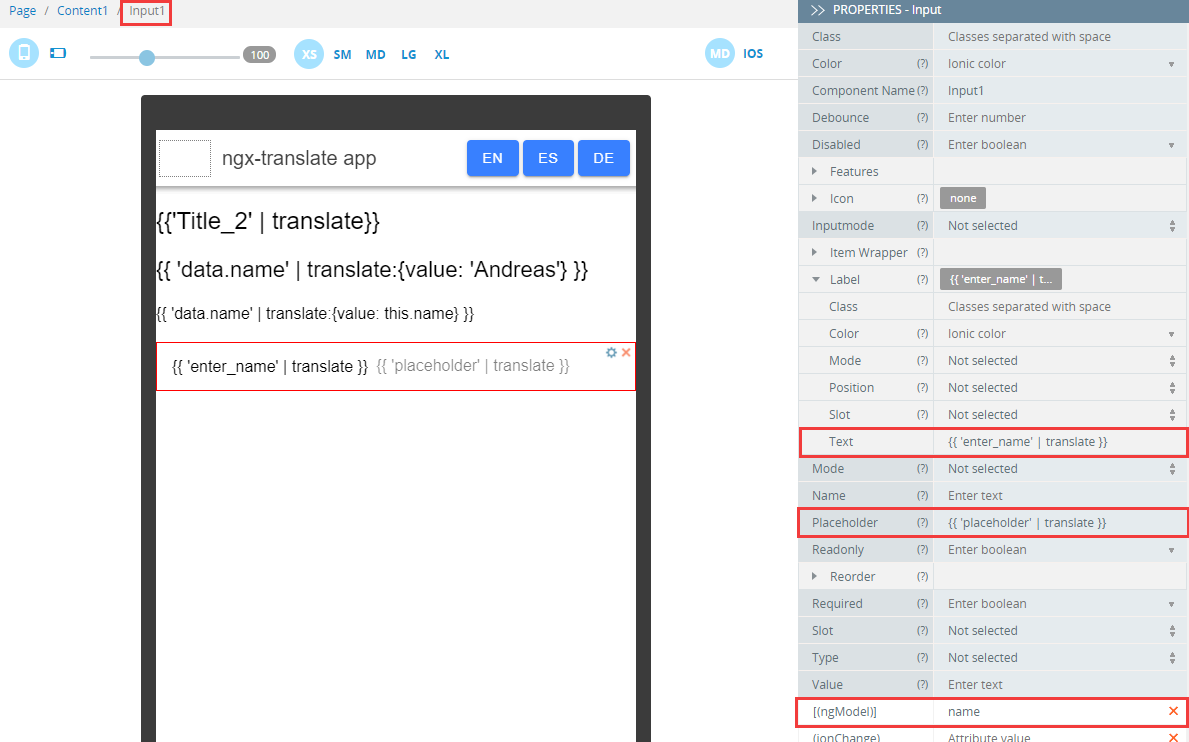
Input component parameters
- Finally, drag & drop the last one Text component to the screen. Change its Container property to p, and the Text property to the following value:
{{ 'data.name' | translate: params1 }}
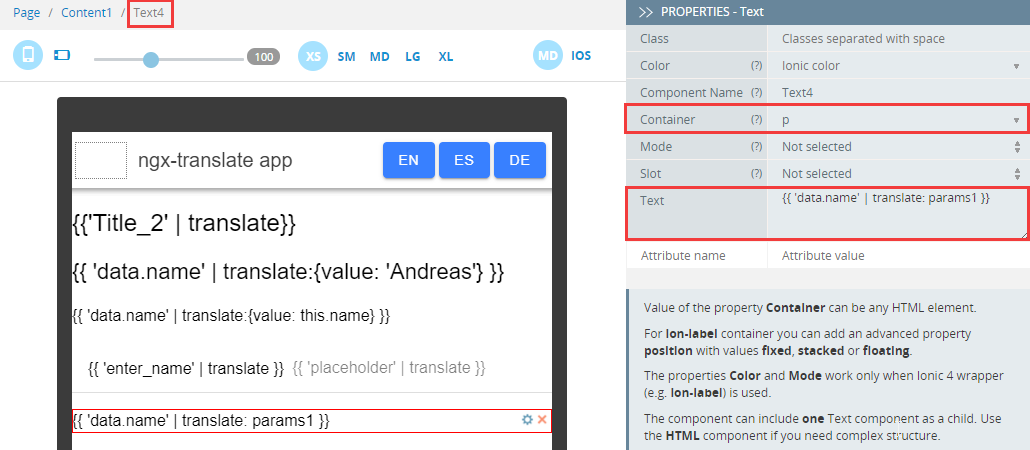
Text4 component parameters
Advanced Attributes
You can also use the advanced attributes [translate] and [translateParams] for translations.
For example, let's add the Card component to Screen1. Inside the Card, choose the CardItemTitle component (for your convenience, you can use the OUTLINE panel to select it).
For the CardItemTitle component, add the property [translate] with the value 'data.name', and the property [translateParams] with the value {value: 'test value'}.
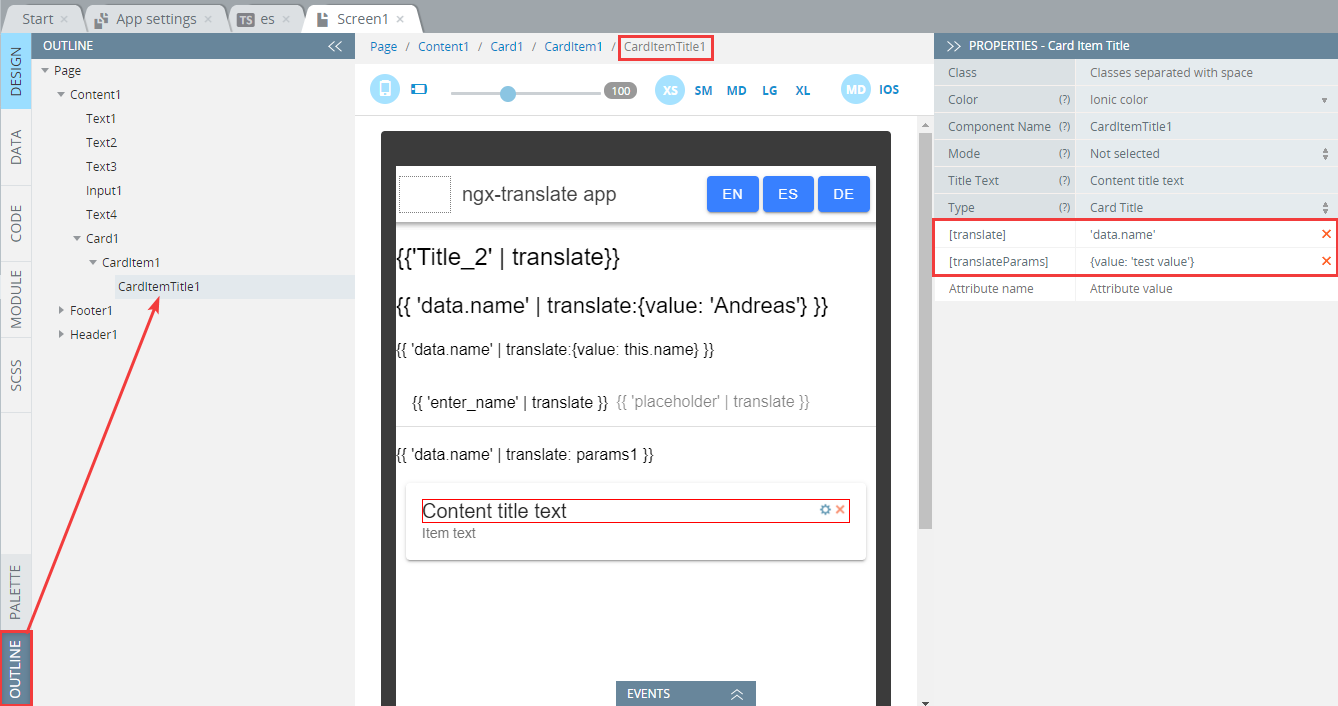
Defining CardItemTitle component
Finally, we are ready to test the app. In the top menu, click the TEST button to test the app. When the app starts, it should work as follows:
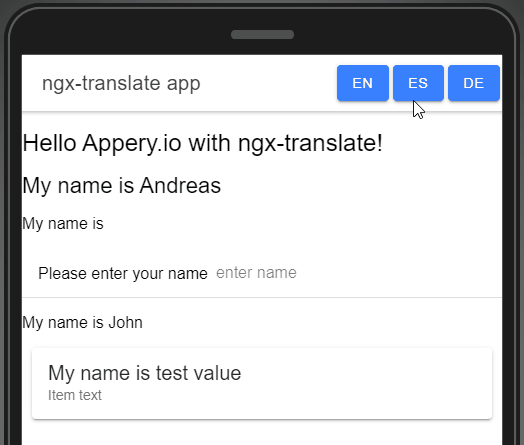
Advanced app performance
Advanced
If the translation text contains some HTML code, you should perform translation via binding to the [innerHTML] property.
In our previously created translation files, the data.name2 value contains HTML code:
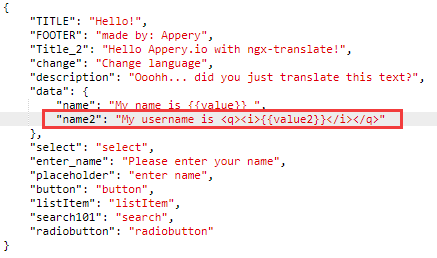
data.name2 value in code
So, let's add one more component to our app.
- Go to the Screen1 DESIGN panel.
- Drag & drop the Text component to the screen and change its Container property to h4, and set its [innerHTML] property to the following value:
'data.name2' | translate: params
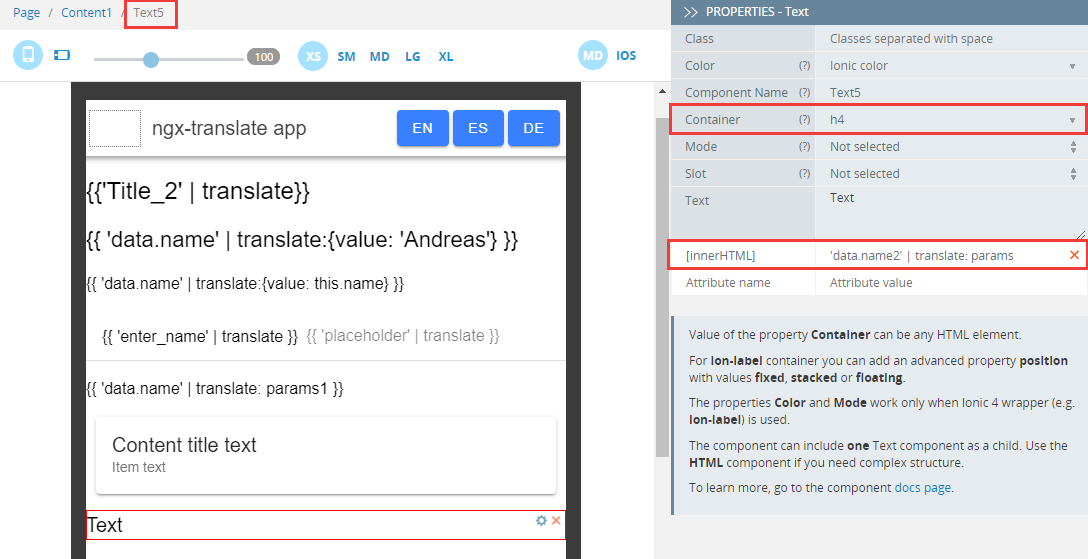
Text5 innerHTML property
- Click the TEST button to test the app. When started, the app should show the formatted text (in our case, the text is "quoted" and has italics style):
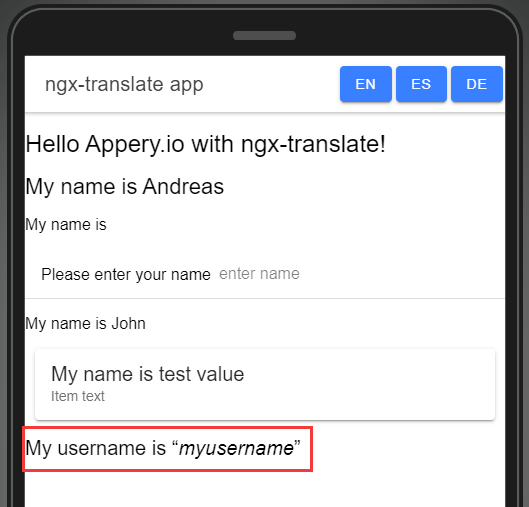
Testing the app
You might also like this Appery.io Community video showing how to create an Ionic 4 application with multilanguage support: