Getting started with Push Notifications for Ionic/Bootstrap/Cordova app.
This quickstart tutorial will show you how to send a Push Notification with an Ionic/AngularJS (and Bootstrap) app.
Important Note!
Note that the option of creating new apps with the Ionic and Bootstrap frameworks was removed but we still support the projects that were created with them earlier.
Apache Cordova (PhoneGap)
Apache Cordova (PhoneGap) is automatically included when you create a new app in Appery.io. The Push Notification component used in Appery.io app is the notification plug-in from Apache Cordova.
To learn more about the component, and any other settings or options, please see the notification documentation page.
Testing Push Notifications
To test sending and receiving Push Notification messages, install the app on the device.
Services in the Plug-in
The Appery.io Push plug-in contains the following services:
PushRegisterDevice Service
PushRegisterDevice is a generic service with a custom implementation. Its request contains parameters whose names correspond with the names of additional columns in the Devices collection.
This generic service response has a structure of the REST response from the Appery.io database.
JavaScript PushRegisterDeviceImpl registers a device with the Apple/Google Push Notification services and adds a registration to the Appery.io Database.
PushUpdateDevice Service
PushUpdateDevice is a generic service with a custom implementation in which the “request” contains additional columns from the Devices collection.
Its response has a structure of a response of the rest from the Appery.io Database.
PushRegisterDeviceImpl sends a REST request to the database to update the device information.
UnregisterDevice Service
PushUnregisterDevice JavaScript sends a REST request to Appery.io Database to delete the device from the database.
To invoke PushUnregisterDevice, use:
Apperyio.get("PushUnregisterDevice")();
PushSend Service
PushSend is a generic service with custom implementation whose request contains fields that will be sent in the body to an Appery.io Push Notification REST endpoint.
The response is a JSON from Appery.io Push REST endpoint.
JavaScript PushSendImpl sends instant Push Notifications to all registered devices. It is important to add the PushApiKey to the PushSettings service.
PushSchedule Service
PushSchedule is another generic service that adds a scheduled Push Notification to the Appery Push Notification scheduler.
It is important to add the PushApiKey to the PushSettings service.
PushSettings Service
PushSettings service is a settings service that stores application Push API Key. It is required for sending notifications but it isn’t required for registering, updating, and deleting devices.
Events
The following events are available in the Appery.io Push plug-in:
- push-notification - fires when the device receives a notification.
- pushinit - fires when the app initializes a connection with the server.
You can handle the events as followed:
document.addEventListener("push-notification", function (event) {
alert(event.detail.message);
});
Before You Start
The Appery.io Push plug-in is imported into an existing app. You can create a brand new Ionic (or Bootstrap) app or use an existing app.
If this is your first time working with Push Notifications, we recommend you create a new app so as not to impact anything else you are working on.
Enabling Push Notifications
Please follow the steps on the Configuration page to learn how to enable and configure Push Notifications.
Designing a Page for Push
Whether you use an existing or a new app, you need to create a very simple UI to handle the Push Notifications.
- When you open an app you will get two default pages: index and Screen1.
- Go to Screen1.
- Place the Button component with Text = Send Push, ng-click = sendPush ().
- This will allow you to send a Push Notification message from the device to yourself.
- When a new app is created all of the core Cordova plug-ins are checked. You may decide to only enable some of them and disable the rest, (in this app, for example, you’ll need only two: Device and PushPlugin) in order to decrease the app size. Only enabled (checked) plug-ins will be included in the binary application.
One more thing you need to do is create scope variables for this page.
- Switch to the SCOPE view of Screen1 and add four variables, all of String type:
- devID.
- timeZone.
- shift.
- message.
You are now ready to add the plug-in to your app.
Importing the Plugin
To add the Appery.io Push plug-in to your app:
- Select Create new > From Plugin.
- Check the Apperyio Push check box and click Import selected plugins. In the Project tree, a list of newly imported services and JavaScript files will be displayed.
Once the Push Notification service has been added to an app it can be invoked. Invoking a service is very similar to invoking a REST API service. Let's see how it's done.
Invoking PushRegister Service
The most important service in the Apperyio Push plug-in is the PushRegisterDevice service. By invoking it the device will be registered for notifications and registration will be added to the Device collection in the database.
- To call the service, in the app open Screen1 and go to the SCOPE tab, add a new function called regPush().
- In the pushReg editor click the arrow to filter snippets, select Invoke service, and click Insert Snippet. For the service_name select PushRegisterDevice.
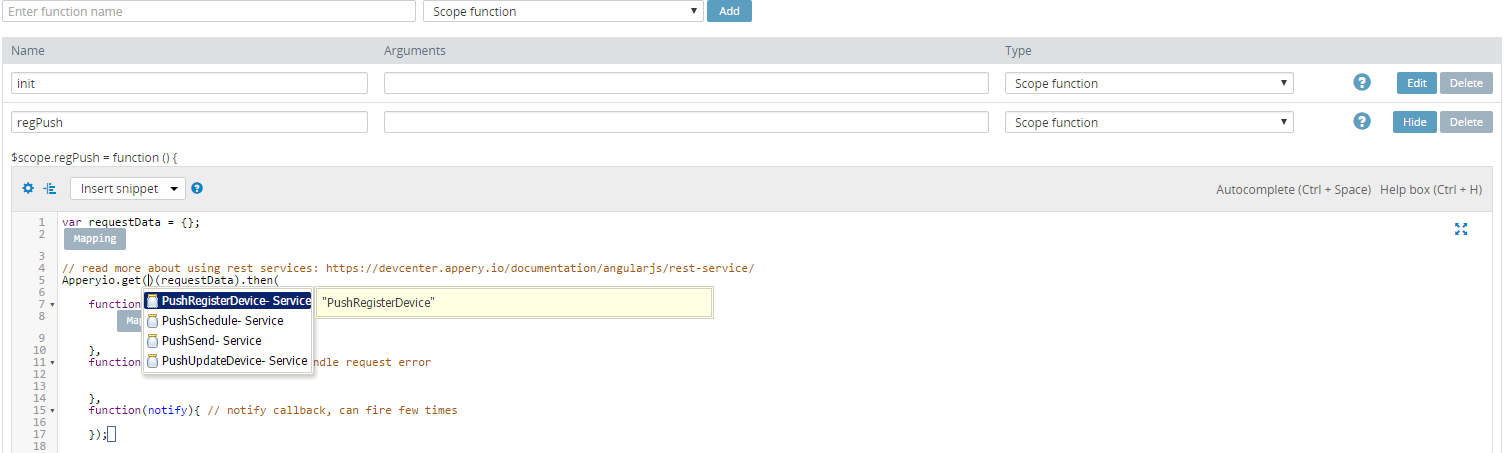
Service invocation.
- You can click Backspace and then Ctrl+Space to autocomplete the service name.
- Click Mapping for success event and create the following mapping: deviceID > devID.
- To show the results add the following code:
5. alert("Success. \n"+"Device ID: "+ $scope.devID);
- Then, add the following snippet into the error callback:
alert("ERROR:\n" + JSON.stringify(error));
- The resulting regPush() function should look like this:
var requestData = {};
Apperyio.get("PushRegisterDevice")(requestData).then(
function(success){
(function mapping2861(success, $scope){
var devID_scope = $scope.devID;
devID_scope = success.deviceID;
$scope.devID = devID_scope;
alert("Success. \n"+"Device ID: "+ $scope.devID);
})(success, $scope);
},
function(error){
alert("ERROR:\n" + JSON.stringify(error));
},
function(notify){
});
You are done setting up the PushRegister device invocation.
Invoking PushSend Service
Now you are going to work on setting up PushSend service.
- To call the PushSend service, in the SCOPE view of the Screen1 page add another function called sendPush().
- In the editor, click the arrow to filter snippets, select Invoke service, and click Insert snippet, then click Backspace. Use Ctrl+Space to get the drop-down with all the available services.
- Select the PushSend service from the list.
- Click Mapping for the request.
- Map scope.message to data.payload.message.
- Click Save & Replace.
- Next, paste the following code at the beginning of the function code:
$scope.message = prompt("Enter your push text: ", "Test push");
- Now, add the following into error callback:
alert("ERROR:\n" + JSON.stringify(error));
Your sendPush () function should look like this:
$scope.message = prompt("Enter your push text: ", "Test push");
var requestData = {};
requestData = (function mapping2733($scope){
var requestData = {};
requestData = Apperyio.EntityAPI('PushSend.request.data', undefined, true);
var message_scope = $scope.message;
requestData.payload.message = message_scope;
return requestData;
})($scope);
Apperyio.get("PushSend")(requestData).then(
function(success){
},
function(error){
},
function(notify){
});
- Now, under the Services folder in the project open the PushSettings service, and pass the app PushApiKey.
To locate the app go to Push Notifications > Settings.
Configuring Init Function
In this step, you are going to configure the app to handle the actual notification event.
- In Scope view, open the init() function of Screen1 and enter the following code:
document.addEventListener("push-notification", function(event) {alert(event.detail.message);});
$scope.regPush();
Your app is now ready to be tested.