Using the Push Notifications in an Ionic App
API Changes
March 20, 2016
If the push notifications feature is used in your app and you decide to switch libraries version from 1.0 to 1.1, you would need to reimport the Appery.io Push Plugin.
November 15, 2015
Push notifications services may not work properly for pushes that are read with event.notification.aps.alert. To solve this problem, in the code, locate this:
event.notification.aps.alert
and replace it with the next string:
event.detail.message
Introduction
This tutorial shows how to create and use the Push notification service in an AngularJS app.
Important Note!
Note that the option of creating new apps with the Ionic framework was removed but we still support the projects that were created with it earlier.
Apache Cordova (PhoneGap)
Apache Cordova (PhoneGap) is automatically included when you create a new project in Appery.io. The push notification component used in Appery.io is the Notification component from Apache Cordova.
Note
To learn more about the component, and any other settings or options, please see the notification documentation page.
Services Description
Note
See also Push notifications overview and Push notifications REST API.
The Appery.io Push plug-in contains the following services:
PushRegisterDevice service
PushRegisterDevice is a generic service with a custom implementation. Its “request” contains the parameters whose names correspond with the names of additional columns in the Devices collection.
This generic service “response” has a structure of the REST response to the Appery.io database.
JavaScript PushRegisterDeviceImpl registers a device with the Apple/Google push services and adds it to the Appery.io database.
PushUpdateDevice service
PushUpdateDevice is a generic service with custom implementation whose “request” contains additional columns in the Devices collection.
Its “response” has a structure of response of the rest to the Appery.io database.
JavaScript PushRegisterDeviceImpl sends REST request to the Appery.io database to update the device information.
UnregisterDevice service
PushUnregisterDevice JavaScript sends REST request to the Appery.io database to delete the device from the database.
To invoke PushUnregisterDevice, use:
Apperyio.get("PushUnregisterDevice")();
PushSend service
PushSend is a REST service. It sends instant push notification to registered devices.
It’s important to add the Push API key to the X-Appery-Push-API-Key header under the Request tab.
Also, to send push notifications, a user has to compose the “message”.
#PushSchedule service
PushSchedule is another REST service. It adds a scheduled push notification to the Appery Push scheduler.
It’s important to add Push API key to X-Appery-Push-API-Key header, see above.
Also, to send push notifications, a user has to set the message, scheduledTime and timeZone data.
Events
The following events are available in Appery.io Push plug-in:
- push-notification – fires when the device receives a notification.
- pushinit – fires when the app initializes a connection with the server.
You can handle this event as follows:
document.addEventListener("push-notification", function (event) {
alert(event.detail.message);
});
Creating App
Design
- Open your Appery.io Ionic/AngularJS project or create a new one. You will get two default pages: index and Screen1.
- Go to Screen1 and place the Button component (to enable sending pushes from device) with Text = Send Push, ng-click = sendPush().
- When a new app is created, all the core Cordova plugins are checked. You may decide to enable only some of them and disable the rest (in this project, for example, you’ll need only two: Device and PushPlugin) in order to decrease the app size. Only enabled (checked) plugins will be included in the binary application and be used for any target device type in events, services, etc.: Project > App settings > Cordova plugins.
Importing Plug-in
- First, in the app, go to SCOPE of Screen 1 and add three variables: devID, timeZone, and mess:
- Then, the Apperyio Push plugin should be imported: CREATE NEW > From Plugin.
- In the new window, check the Apperyio Push checkbox and click Import selected plugins.
- In the Project tree, a list of newly imported services and JavaScripts will appear.
After native services have been added to the app, they can be called. Invoking a native service is very similar to invoking a REST service.
Invoking PushRegister Service
The most important service in the Apperyio Push plug-in is PushRegisterDevice service. By invoking it, the device will be registered (added) to the database.
- To call the service, in the project, open Screen 1, go to the SCOPE tab, add a new function – regPush(), and click Add, opening the function editor.
- In the editor, click the arrow to filter snippets, select Invoke service, and click Insert snippet and, for service_name, select PushRegisterDevice:
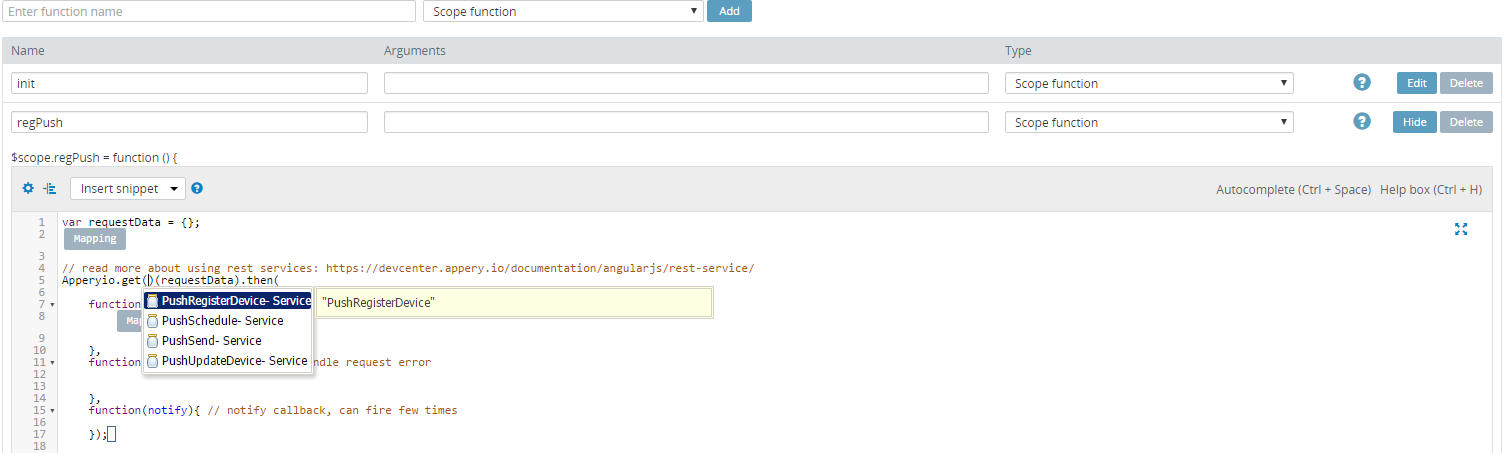
Note
You can click Backspace and then CTRL+SPACE to autocomplete the service name.
- Click Mapping for success event and create the following mapping.
- To show the result, add the following to the code:
alert("Success. /n"+"Device ID: "+ $scope.devID);
- Then, add the following snippet from the event handler to the end of the code:
alert("ERROR:/n" + JSON.stringify(error));
- The resulting regPush() function should look like:
var requestData = {};
Apperyio.get("PushRegisterDevice")(requestData).then(
function(success) {
(function mapping8634(success, $scope) {
var devID_scope = $scope.devID;
devID_scope = success.deviceID;
$scope.devID = devID_scope;
alert("Success. /n" + "Device ID: " + $scope.devID);
})(success, $scope);
},
function(error) {
alert("ERROR:/n" + JSON.stringify(error));
},
function(notify) {});
Invoking PushSend Service
- To call the PushSend service, in the SCOPE view of the Screen1 page, add another new function – sendPush() and, by clicking Add, open the function editor.
- In the editor, click the arrow to filter snippets, select Invoke service, and click Insert snippet, then click Backspace and then – CTRL+SPACE to get the drop-down with all the available services.
- Select the PushSend sevice from the list.
- Click Mapping for request, create the following, then click Save & Replace.
- Next, paste the next code to the beginning of the code:
$scope.mess = prompt("Enter your push text: ", "Test push");
- Your sendPush() function should look like:
$scope.mess = prompt("Enter your push text: ", "Test push");
var requestData = {};
requestData = (function mapping4743($scope) {
var requestData = {};
requestData.data = Apperyio.EntityAPI('PushSend.request.body', undefined, true);
var mess_scope = $scope.mess;
requestData.data.payload.message = mess_scope;
return requestData;
})($scope);
Apperyio.get("PushSend")(requestData).then(
function(success) {},
function(error) {
alert("ERROR:n" + JSON.stringify(error));
},
function(notify) {});
- Now, under the Services folder in the project, open the PushSend service, and pass the app X-Appery-Push-API-Key under Request > Headers:
Note
Similarly, the app X-Appery-Push-API-Key must be passed under Request > Headers for PushSchedule service. To locate the app X-Appery-Push-API-Key, go to: Push Notifications > Settings.
Configuring init
Function
init
FunctionNow, open the code editor of the init() function of Screen1 and pass the following code:
$scope.timeZone = "GMT+01:00";
document.addEventListener("push-notification", function(event) {alert(event.detail.message);});
$scope.regPush();
It allows scheduling pushes and monitoring the changes of some predefined variables and responding with predefined actions.
Your app is ready, but, before you can start testing it, go to Push > Push Notifications > Settings and make sure you’ve enabled and configured your push settings, linked the app with the database, and passed the device credentials.
When testing the app, you’ll get:
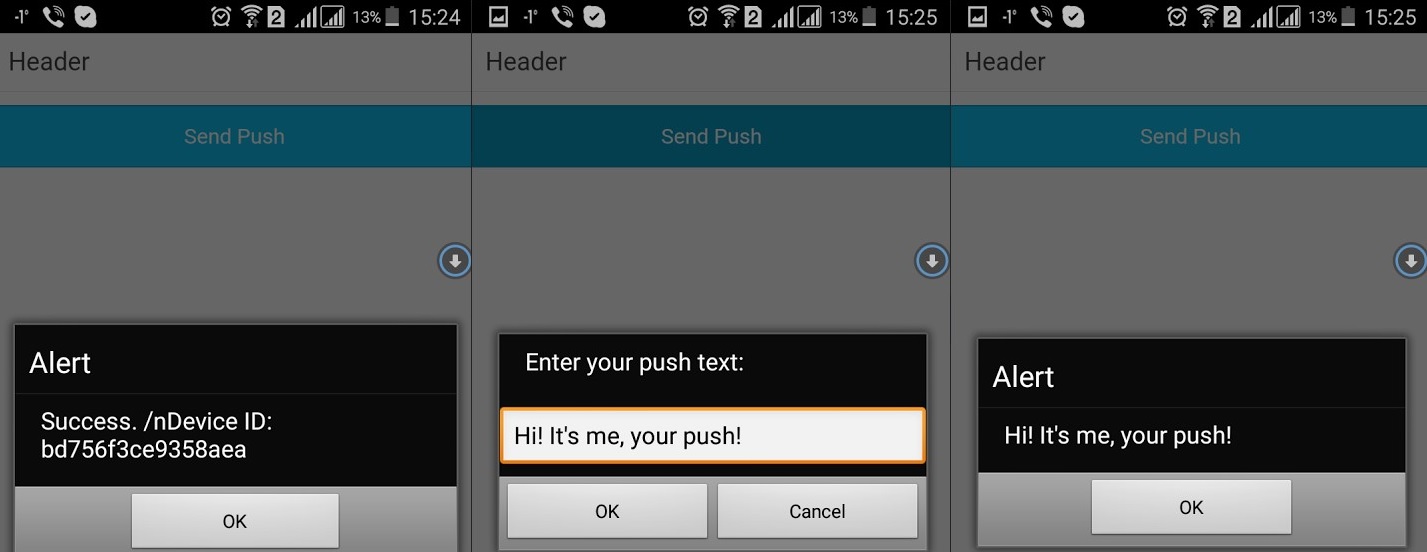
Testing Options
Android
Testing on Android is relatively simple, since you can quickly install any app on your device.
To do it, build the Android binary and install it on your device. When the build is completed, you’ll see a QR code. Scanning the QR code will download the app to your phone. You can also email the app to your device.
iOS
Build the iOS binary and install it on your device.
Important Note
Please, note that to export the app for iOS, you will need to upload your distribution certificate and provisioning profile obtained from Apple under the App settings > iOS binary tab.
You can check this document in case you need help with exporting your application for iOS. Here, you will find the document that explains how to manage certificates in Appery.io.