Using the Barcode scanner service in an Ionic app
Introduction
This quick tutorial shows how to create an AngularJS app that uses the Cordova Barcode Scanner.
Want to know more?
Check here to learn how to create your Ionic 5 mobile application with a barcode scan feature by adding the Barcode Scanner plug-in.
Important Note!
Note that the option of creating new apps with the Ionic framework was removed but we still support the projects that were created with it earlier.
Ionic 5 Barcode Scanner Tutorial
You can also check our step-by-step Ionic 5 Barcode Scanner Plug-in Sample App tutorial that explains how to build an app that launches a barcode scanner on a button click. After the scan is completed, the scan information will be displayed.
Apache Cordova (PhoneGap)
Apache Cordova (PhoneGap) is automatically included when you create a new app in Appery.io.
Cordova Barcode Scanner Plugin
To learn more about the component, any other settings or options, please see the PhoneGap barcodescanner-plugin page.
Creating App
The first step is to create a new app. You can also use an existing app.
Design
- Open your Appery.io Bootstrap & AngularJS app or create a new one. You will get two default pages: index and Screen1.
- Go to Screen1 and create the following UI consisting of:
- A Button component. Set its Text to scan .
- A Text component.
The UI should look like this:
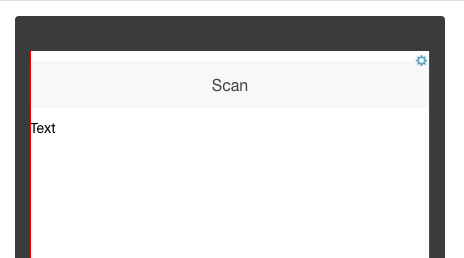
Ionic Barcode Scanner app .
Now, let’s create services and variables and bind them with UI components.
Scope
- Go to SCOPE and add a variable called scannedValue of type String.
- Then, to add the BarcodeScanner plugin, go to Project > Create new > From Plug-in.
- In the new window, select Apperyio Barcode Service and click Import selected plugins.
- Click Apply settings on the next page and the plug-in is imported. You should see Barcode_scan service and Barcode JavaScript file.
- To invoke the service you just imported, in SCOPE view of the Screen1 page, add a new function: scan, and click the Add button.
- Using drag-and-drop, select the Barcode_scan service and drag it inside the scan function editor. The service invocation code will be automatically inserted.
- Inside the success callback, click CLICK TO EDIT MAPPING to open the visual binding editor.
- Inside the visual binding editor, click Expand all on both sides.
- Using drag and connect, connect text (from left) to scannedValue on the right. Once the connection line is drawn, click Save & Replace button to generate the mapping code.
Binding Scope to Page
The last step before testing is to bind the scope to the page.
- Open the DESIGN view.
- Select the button. In PROPERTIES view, for ng-click, place the cursor inside the value field and press Ctrl+Space. From the list, select the scan function.
- Select the Text component. For its Text property, set it to {{scannedValue}}. You can type {{}} and the invoke code assist.
Testing App
As the Barcode scanner is a native API, it has to be tested on the device.
Android
Testing on Android is relatively simple, since you can quickly install any app on your device.
To do it, build the Android binary and install it on your device. When the build is completed, you’ll see a QR code. Scanning the QR code will download the app to your phone. You can also email the app to your device.
iOS
Build the app and install it on your device.
Important Note
Please, note that to export the app for iOS, you will need to upload your distribution certificate and provisioning profile obtained from Apple under the App settings > iOS binary tab.
You can check this document in case you need help with exporting your application for iOS. Here, you will find the document that explains how to manage certificates in Appery.io.
API Changes (November 2015)
BarcodeScanner services created before these changes may not work properly. To solve this problem, the Barcode.js custom JavaScript should be replaced by the new code shown below.
define(['require', 'lodash'],
function (require, _) {
return [{
type: 'service',
name: 'Barcode_scan',
deps: ['Apperyio', scan]
}];
function scan(Apperyio) {
var $q = Apperyio.get('$q');
return function (config) {
var deferred = $q.defer(),
serviceSettings = Apperyio.EntityAPI("Barcode_scan");
if (_.isUndefined(serviceSettings.echo)) {
if (!window.cordova == undefined || window.cordova.plugins == undefined || window.cordova.plugins.barcodeScanner == undefined) {
deferred.reject('BarcodeScanner not found');
} else {
cordova.plugins.barcodeScanner.scan(deferred.resolve, deferred.reject);
}
} else {
deferred.resolve(JSON.parse(serviceSettings.echo));
}
return deferred.promise;
};
}
});