ETHEREUM Component
Using the ETHEREUM component to work with the Ethereum platform
The ETHEREUM component allows you to work with the Ethereum platform without using 3rd party services.
Ethereum platform
Ethereum is a decentralized platform that runs smart contracts: applications that run exactly as programmed without any possibility of downtime, censorship, fraud or third-party interference.
These apps run on a custom built blockchain, an enormously powerful shared global infrastructure that can move value around and represent the property ownership.
Private Key
Generation of an Ethereum key is based on the elliptical curve ecp256k1. On EC ecp256k1, any number between 1 to 2^256-1 is a valid private key.
Example: afdfd9c3d2095ef696594f6cedcae59e72dcd697e2a7521b1578140422a4f890.
Address
Ethereum addresses are composed of the prefix 0x, a common identifier for hexadecimal, concatenated with the rightmost 20 bytes of the Keccak-256 hash (big endian) of the ECDSA public key. In hexadecimal, 2 digits represent a byte, meaning addresses contain 40 hexadecimal digits. Example: 0xb794f5ea0ba39494ce839613fffba74279579268.
Ethereum Call
A call is a local invocation of a contract function that does not broadcast or publish anything on the blockchain.
It is a read-only operation and will not consume any Ether. It simulates what would happen in a transaction, but discards all the state changes when it is done.
It is synchronous and the return value of the contract function is returned immediately. In Solidity, the function is marked constant.
Ethereum Transaction
A transaction is broadcast to the network, processed by miners, and if valid, is published on the blockchain.
It is a write-operation that will affect other accounts, update the state of the blockchain, and consume Ether (unless a miner accepts it with a gas price of zero).
It is asynchronous because it is possible that no miners will include the transaction in a block (for example, the gas price for the transaction may be too low). Since it is asynchronous, the immediate return value of a transaction is always the transaction hash. In Solidity, the function is not marked constant.
The algorithm for obtaining an account address from private key
1. Generating private key
A private key is 64 hexadecimal characters. Every single string of 64 hex is, hypothetically, an Ethereum private key (see link at the top for why this is not totally accurate) that will access an account. If you plan on generating a new account, you should be sure these are seeded with a proper RNG. Once you have that string.
2. Private Key -> Public Key
Your private key and the public key should be made according to the Elliptic Curve Digital Signature Algorithm (ECDSA) and, as a result, you will have a public key that is 64 bytes.
Principle of ECDSA
ECDSA stands for “Elliptic Curve Digital Signature Algorithm”, it is used to create a digital signature of data (a file for example) in order to allow verification of its authenticity without compromising its security. The ECDSA algorithm is basically all about mathematics. You have a mathematical equation which draws a curve on a graph, and you choose a random point on that curve and consider that your point of origin.
Then you generate a random number, this is your private key, you do some mathematical equation using that random number and that “point of origin” and you get a second point on the curve, that’s your public key. When you want to sign a file, you will use this private key (the random number) with a hash of the file (a unique number to represent the file) into an equation and that will give you your signature.
The signature itself is divided into two parts, called R and S. In order to verify that the signature is correct, you only need the public key (that point on the curve that was generated using the private key) and you put that into another equation with one part of the signature (S), and if signed correctly using the private key, it will give you the other part of the signature (R). So to make it short, a signature consists of two numbers, R and S, and you use a private key to generate R and S, and if a mathematical equation using the public key and S gives you R, then the signature is valid. There is no way to know the private key or to create a signature using the public key only.
3. Public key -> Address
Start with a public key (128 characters / 64 bytes).
Take the Keccak-256 hash of the public key. You should now have a string that is 64 characters / 32 bytes. (note: SHA3-256 eventually became the standard, but Ethereum uses Keccak).
Take the last 40 characters / 20 bytes of this public key (Keccak-256). Or, in other words, drop the first 24 characters / 12 bytes. These 40 characters / 20 bytes are the address. When prefixed with 0x, it becomes 42 characters long.
ETHEREUM Component
The easiest way to work with Ethereum is by using the ETHEREUM component.
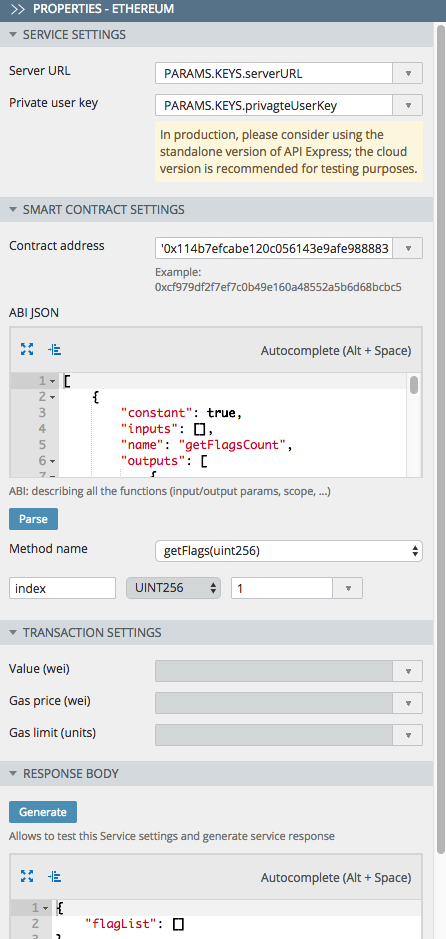
Server URL - url of the Ethereum client
Private user key - User private key
Contract address - Smart contract address
ABI JSON - Application Binary Interface determines such details as how functions are called and in which binary format information should be passed from one program component to the next
contractAddress - address of a smart contract
Method name - Invoking method name
Value (wei) - Amount of transferred ethers
Gas price (wei) - Gas price
Gas limit (units) - Gas limit
Ethereum Properties
{"status": "success"}
If the invocation of the ETHEREUM component fails, the response is:
{
"status": "failed",
"failedDetailsMessage": "Invalid amount of gas"
}
What is Gas?
Gas is a unit of measuring computational works of running transactions or smart contracts in the Ethereum network. This system is similar to the use of kilowatts (kW) for measuring electricity in your house; the electricity you use is not measured in dollars and cents but instead in kWH or Kilowatts per hour.
It is important to understand that different kinds of transaction require different numbers of gas to complete. For instance, a simple transaction of sending ETH from one place to another makes 21,000 Gas while sending ICO tokens from your MyEtherWallet (MEW) wallet costs much more due to higher levels of computation ended.
Gas Limit
Gas limit refers to the maximum number of gas you are willing to spend on a particular transaction. Higher gas limits mean that more computational work must be done to execute the smart contract. A standard ETH transfer requires a gas limit of 21,000 units of gas.
Gas Price
Gas price refers to the number of Ethers you are willing to pay for; every unit of gas and is usually measured in “Gwei”. An analogy for the gas price – relating to the previous analogy for gas limits – is that it is similar to the cost of each litre of fuel that you are paying for filling up your car tank.
Unit | Wei |
---|---|
Wei | 1 |
Kwei | 1,000 |
Mwei | 1,000,000 |
Gwei | 1,000,000,000 |
Szabo | 1,000,000,000,000 |
Finney | 1,000,000,000,000,000 |
Ether | 1,000,000,000,000,000,000 |
Wei is the smallest unit of Ether, and a Gwei consists of a billion Weis.
Samples
Let's consider the "HelloWorld" example. The Smart contract greeter which can store and return the string value:
pragma solidity >=0.4.22 <0.6.0;
contract greeter {
/* define variable greeting of the type string */
string greeting;
/* this runs when the contract is executed */
constructor() public {
greeting = "Hi";
}
function setGreeting(string memory _greeting) public {
greeting = _greeting;
}
function getGreeting() public view returns (string memory) {
return greeting;
}
}
The Application Binary Interface of the greeter smart contract:
[
{
"constant": false,
"inputs": [
{
"name": "_greeting",
"type": "string"
}
],
"name": "setGreeting",
"outputs": [],
"payable": false,
"stateMutability": "nonpayable",
"type": "function"
},
{
"inputs": [],
"payable": false,
"stateMutability": "nonpayable",
"type": "constructor"
},
{
"constant": true,
"inputs": [],
"name": "getGreeting",
"outputs": [
{
"name": "",
"type": "string"
}
],
"payable": false,
"stateMutability": "view",
"type": "function"
}
]
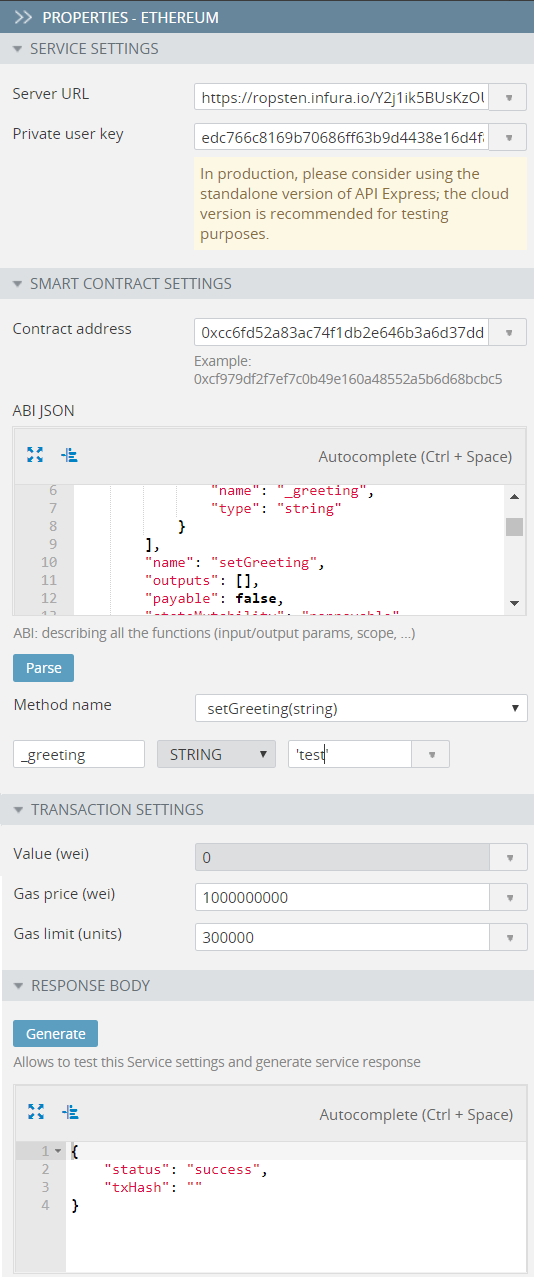
AppClient
Appery.io provides an opportunity to work with Ethereum directly using the AppClient library of API Express.
The Ethereum Folder contains the following generic services:
AppClientETHSettings - settings to work with Ethereum
host - url of the Ethereum client
abi - Application Binary Interface determines such details as how functions are called and in which binary format information should be passed from one program component to the next
contractAddress - address of the smart contract
gasPrice
gasLimit
AppClientETHCall - invokes the Ethereum method
methodName - name of the smart contract method
parameters - a list of parameters can be passed as an array [param1, param2, ...] or as an object {"param1" : param1, "param2": param2}
AppClientETHTransaction - creates the Ethereum transaction
methodName - name of the smart contract method
parameters - a list of parameters can be passed as an array [param1, param2, ...] or as an object {"param1" : param1, "param2": param2}
value - number of ethers which will be sent on a contract
gasPrice - gas Price
gasLimit- gas Limit
password - password for getting access to private keys
Response
txHash - transaction hash code (for example 0x73d419e3a9a63aab7ee4f3be43c0df0175f4395615945d395c827bffb3bbfecc)
AppClientETHCreateAccount - creates a new Ethereum account
password - password for encrypting private keys
Response
account - account address
AppClientETHGetAccount - returns the current account
Response
account - account address
AppClientETHGetBalance - returns the number of ethers of the Ethereum account
account - account address
Response
balance - number of the Ethereum account ethers
AppClientETHTransferEther - transfers ethers from one account to another
to - destination account address
value - number of the transferred ethers
gasPrice - gas Price
gasLimit - gas Limit
password - password for getting access to a sender private key
Response
txHash - transaction hash code
AppClientETHSetPrivateKey - setting an account private key
privateKey - Ethereum account private key
password - password for encrypting a private key
Response
AppClientETHExportPrivateKey - exports the Ethereum account private key
*password - password for getting access to a sender private key
Response
privateKey - Ethereum account private key
AppClientETHGetTransaction - returns information about a just created transaction
txHash - transaction hash code
Response
{"jsonrpc":"2.0","id":1,"result":{"blockHash":"0x49d03f4c027264d247a01ce00f455e829a058c0c505e1fe4afa54e091122374c","blockNumber":"0x3055f2","from":"0xfc0d335cf35800334add0e1bd32fd2b86e61900d","gas":"0x1806f9","gasPrice":"0x12a05f200","hash":"0x7b228690a9e54999b88e6d6dac61e22ebf09fece1d215a7085d7b7b993127cac","input":"0x60806040526103e860005534801561001657600080fd5b5064e8d4a51000600160003373ffffffffffffffffffffffffffffffffffffffff1673ffffffffffffffffffffffffffffffffffffffff16815260200190815260200160002081.....","nonce":"0x6","to":null,"transactionIndex":"0x1","value":"0x0","v":"0x29","r":"0xfccbadba6441e8b383a10506aa801d188e4e03874f52f1f5e3989cff1388a64e","s":"0x5132e6bee7c3dbc3ca0b3f5598092e5bc256ea2ed8fbd78b86ff69d963c80af6"}}
AppClientETHGetTransactionReceipt - returns information about the transaction already added into the ledger
txHash - transaction hash code
Response
{"jsonrpc":"2.0","id":2,"result":{"blockHash":"0x49d03f4c027264d247a01ce00f455e829a058c0c505e1fe4afa54e091122374c","blockNumber":"0x3055f2","contractAddress":"0xd7fcef447e0ec3127581b0e3f1bfc794b5b42f91","cumulativeGasUsed":"0x3981cc","from":"0xfc0d335cf35800334add0e1bd32fd2b86e61900d","gasUsed":"0x1806f9","logs":[],"logsBloom":"0x00000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000000","status":"0x1","to":null,"transactionHash":"0x7b228690a9e54999b88e6d6dac61e22ebf09fece1d215a7085d7b7b993127cac","transactionIndex":"0x1"}}
Updated over 4 years ago