Model and Storage
Model and Storage in a jQM app
Important Note!
The option of creating new apps with the jQuery Mobile framework was removed but we still support the projects that were created with it earlier.
Introduction
A model helps define the data structure for an app in order to simplify development. In general, a model allows you to mimic real life scenarios in your app. For instance, if you are building an app that has reviews about cars, you can define a Car object.
Here’s where the storage feature comes in. Let’s say your app shows reviews about five cars, and you need to keep (save) the data somewhere in the app. A single car or a collection of cars can be saved into storage.
Go to Project > Model and Storage to define models or storage variables.
Type a name for new entity (model) and click Add to create it. Create the data structure you need by clicking + and specifying the field names and types. When you are done defining the entity structure you can create a storage variable with a matching type. Switch to the Storage tab and create a new storage variable. You will see that there is a todo type in the Type drop-down list. That means that this storage variable will contain the same structure as the model.
Model
A model is a kind of a custom data structure that can be used to create new objects based on itself. Variables that are stored in certain storage can also use models as structure templates.
Go to Project > Model and Storage to create a new model and specify its structure.
Here is an example of an imaginary customer structure:
Supported Data Types
Model can be type of Array or Object.
When defining a model, you can use the following data types to specify the type for a specific model field:
- String.
- Boolean.
- Number.
- Array.
- Object.
Model API
View Model API in the Reference section.
Storage
Storage is a virtual client-side place where any data can be stored as a string. By using the models, data can be stored in specific storage based on the chosen model structure. Depending on the chosen storage, the stored data will be accessible for different amounts of time.
Storage Types
There are 3 storage types you can choose in Appery.io:
- localStorage – Data stored through localStorage does not expire and remains stored on the user’s computer until an app deletes it or the user removes it through the browser settings.
- sessionStorage – Data stored through the sessionStorage has the same lifetime as the window or browser tab, which means that when the window or tab is closed, any data stored will be deleted.
- Window – Variables in Window are the same as the simple variable of root window object. That means that data stored in the Window can be accessed the same way as the window variables. The lifetime of such variables are the same as the window lifetime (and sessionStorage variables lifetime).
Help
Read more about HTML5 Web Storage via the link.
Storage API
View Storage API in the Reference section.
Mapping Expression
The Mapping Expression is a feature provided by Appery.io that is similar to JSONPath. But, unlike JSONPath, the Mapping Expression makes it possible to read and write values by the specified path. Let’s say you have the following object:
{"users":{"FirstName":"Sam","LastName":"Winchester"}}
Using the Mapping Expression, you can retrieve only certain fields instead of the whole object:
Apperyio.storage.storageVariable.get("$['FirstName']"); //returns "Sam"
To retrieve a more complex structure as an array, use [i] identifier. In this case, an array will be returned. Let’s say you have the following object:
{"users":[{"Name":"Sam"},{"Name":"Billy"}]}
Apperyio.storage.storageVariable.get("$['users'][i]['Name']"); //returns ["Sam", "Billy"]
You can provide a specific array index instead of [i] identifier to return a specific array value. In that case, a plain result will be returned (not an array):
Apperyio.storage.storageVariable.get("$['users'][0]['Name']"); //returns "Sam"
Best Practices
Let’s say there is a customer collection in the database with the following structure:
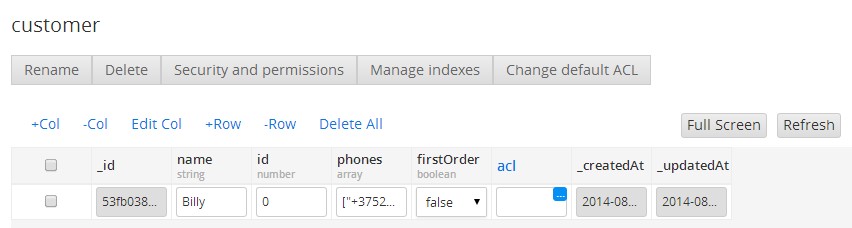
Database collection.
It’s easy to create a model with the same structure:
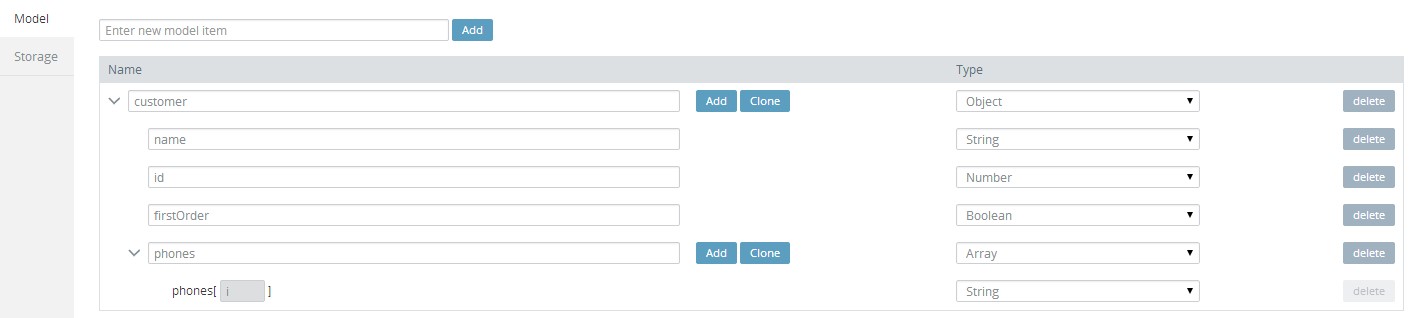
App model.
Then you can create a storage variable based on the model structure:

App storage.
Now, when you use a REST service to get customer collection data, you can map its response to your local storage variable, as in the following:
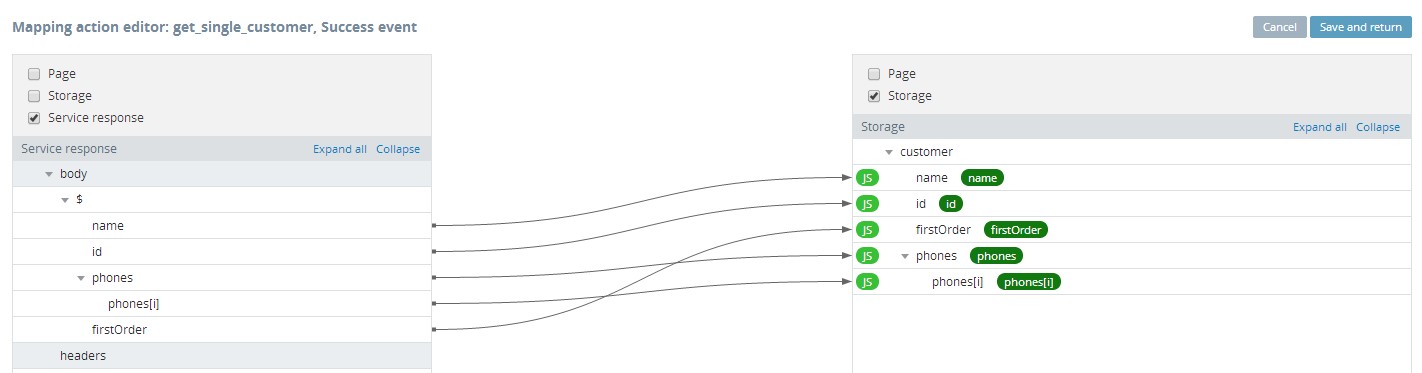
Mapping.
This approach will be effective only when you need to get a single item for the customer collection. To get the whole list of customers, you should create another model with type of Array and array items type as customer:
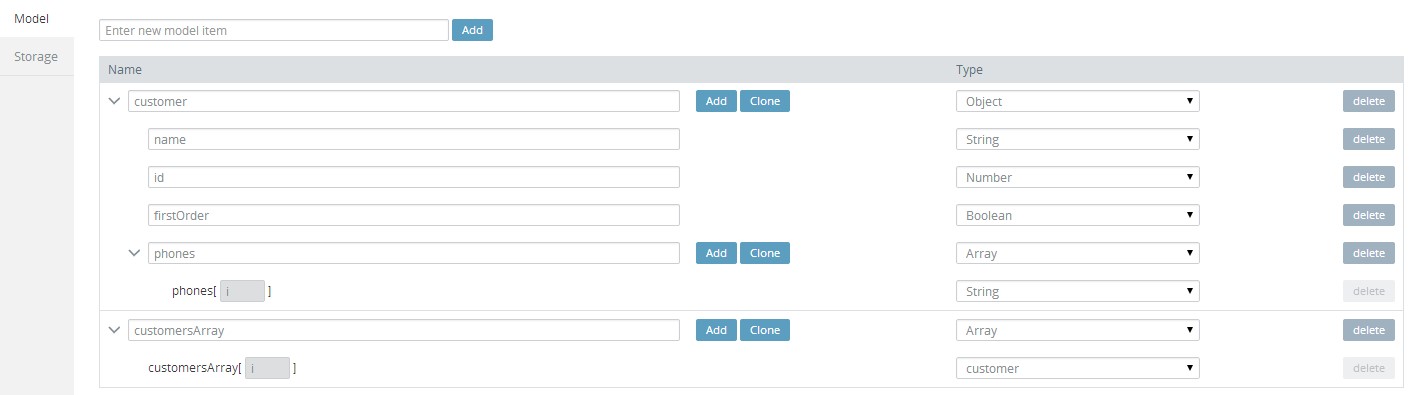
App model.
Note the second model with name customersArray. Now you can change your local storage variable type to customersArray.
After the following mapping, local storage variable customer will contain an array with customers:
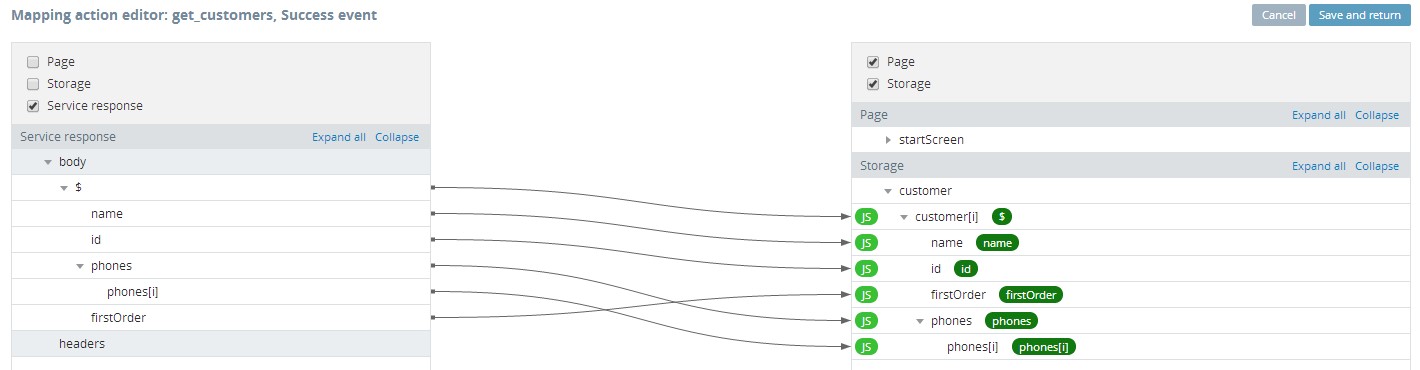
Mapping.
Updated almost 2 years ago