Form
Overview of the Form Component
Handling user input with forms is the cornerstone of many common applications. Applications use forms to enable users to log in, update a profile, enter sensitive information, or to perform many other data-entry tasks.
The Form component is based on Angular template-driven forms.
Composition
The Form is a container that can include Form Controls like Checkbox, Datetime, Input, Radiogroup, Range, Select, and Toggle).
To create a Form Control, drag & drop a needed component from the components PALETTE and place it inside the Form to see the Form Control section with more control properties added:
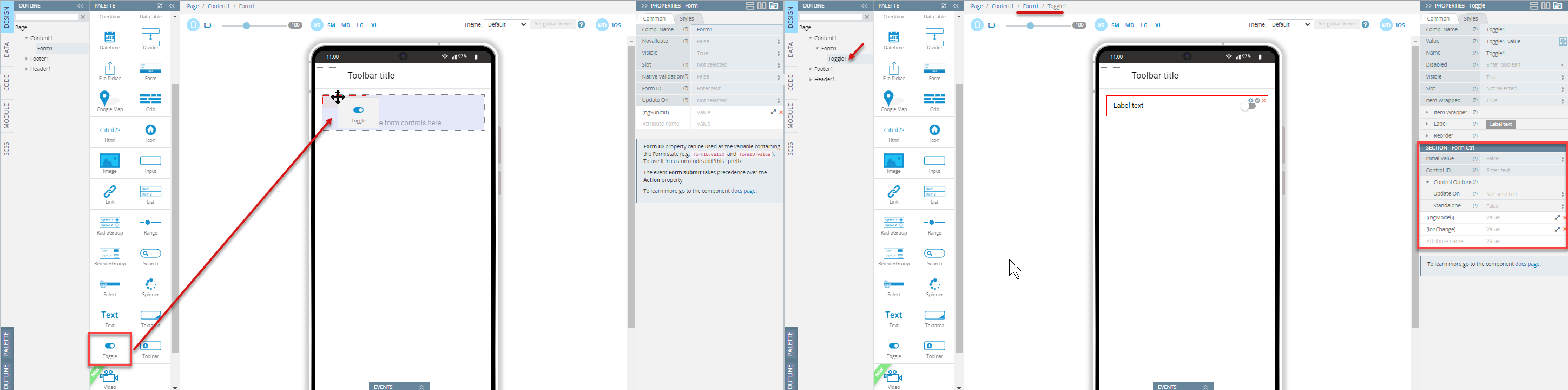
The added component will become a Form Control only when the property Name is specified which defines the control data key.
For example, we have an Input with definedName = userName
andvalue = Peter
. In this case, the control data will be available as the property of the form data object:{userName: 'Peter'}
.
Sending data
There are two ways to send form data:
- Including the special Submit control. The control has to be placed inside the form:
<form>
<ion-button type="submit">Submit</ion-button>
</form>
- Using the Form submit action on the EVENTS tab. The control can be placed everywhere on the page.
Actions at the form submitting can be specified in three ways:
- Using the Form submit event of the form component on the EVENTS tab (for beginners). In this case, a user can choose an action to be executed at the form submitting. For example, such action can be Invoke service (e.g. Login service) with specified mapping on the DATA panel.
- Using the (ngSubmit) event of the component (for advanced users). In this case, a user has to define a function - handler which will execute submitting from custom code.
- Using the Action property of the component (for advanced users). In this case, a user has to define the URL where form data will be sent.
Validation
By default, Ionic has its own validation rules for controls included in the form. If you want to use the validity of the form in general, specify the property Form ID.
The word passed as the value of the property can be used as a variable containing information about the form. Such usage is described in the PROPERTIES section:
The Form ID property can be used as the variable containing the Form state (e.g. formID.valid
and formID.value
). To use it in custom code, add 'this.'
prefix.
Validation ComponentTo define validation for the UI components that do not become the Validation property when included in the Form or are difficult to combine for validation purposes the Validation component can be used.
Form and Controls have different states when Ionic validation is used.
State | Description |
---|---|
valid | A control is considered valid if no validation errors exist with the current value. If the control is not present, null is returned. |
invalid | An error exists in the input value. If the control is not present, null is returned. |
pending | Async validation is occurring and errors are not yet available for the input value. If the control is not present, null is returned. |
disabled | The control is disabled in the UI and is exempt from validation checks and excluded from aggregate values of ancestor controls. If the control is not present, null is returned. |
enabled | The control is included in ancestor calculations of validity or value. If the control is not present, null is returned. |
pristine | The user has not yet changed the value in the UI. If the control is not present, null is returned. |
dirty | The user has changed the value in the UI. If the control is not present,null is returned. |
touched | The user has triggered a blur event on it. If the control is not present, null is returned. |
untouched | The user has not yet triggered a blur event on it. If the control is not present, null is returned. |
errors | Reports the control's validation errors. |
For example, check using the invalid input state to show an error message: <ion-text *ngIf="user.invalid" color="danger">Incorrect user name</ion-text>
. In this case, user is a Control ID defined for the Input component.
If you need to use validation to specific errors, use the errors state with specifying the type of validation error.
Let's see how to define validation for the UI components that do not become the Validation property when included in the Form component. how different error messages at the Input component validation are displayed.
Define the Control ID property of the Input with the passControl value and set its validation (to required
and pattern="[0-9]*"
, for example).
Add two components containing error messages and add the *ngIf directive as an advanced property with the value that is a condition for displaying the error component. The directive is used to show/hide components depending on a directive boolean value.
If the component is valid, the expression passControl.errors
will return null
(equal to false
).
If the component is invalid, the expression passControl.errors
will return the object containing all validation errors available by their names. The existence of a necessary error name in the returned errors object is used for the condition of displaying the error message.
<form #myForm="ngForm">
<ion-input required pattern="[0-9]*" #passControl="ngModel" [ngModel] name="pass"></ion-input>
<ion-text *ngIf="passControl.errors && passControl.errors.required" color="danger">Value has to be entered</ion-text>
<ion-text *ngIf="passControl.errors && passControl.errors.pattern" color="danger">Value should contain only numbers</ion-text>
</form>
Want to know more?More details with examples about the Validation property can be found [here](https://docs.appery.io/docs/ionic-5-property-validati
Properties
Important Note!This document lists the properties that are specific to this particular UI component.
To check for the properties common for most UI components, please check the General components document.
Common Properties
Property Name | Property Description |
---|---|
Novalidate | This boolean attribute indicates that the form shouldn't be validated when submitted. |
Native validation | If set to True, native HTML5 validation is used instead of Angular validation. The form will work as the default HTML <form>. |
Form ID | A unique word identifying the component (Template reference variable) that contains current form information. Used in component's properties as a variable containing the form state. |
Update On | Control when the value or the validity is updated: Change, Blur, Submit |
Features | Advanced options containing default HTML form attributes.
|
(ngSubmit) | The event emitted when the form is submitted. |
In the initial state of the component, the default HTML
<form>
features (Action, Target, etc.) are not supported because they are replaced with Angular functionality. To disable Angular functionality and enable default HTML behavior of the component, enable the Native validation property.
The Form ID property can be used in a template or code as a variable containing information about the form:
formID = {
value: boolean, // Reports the value of the form
valid: boolean, // Reports whether the form is valid. A form is considered valid if no validation errors exist with the current value
invalid: boolean, // Reports whether the form is invalid, meaning that an error exists in the value
dirty: boolean // Reports whether the form is dirty, meaning that the user has changed the value in the UI
}
In templates, the Form ID property is used as a variable. It can be also passed as a function argument:
<ion-button disabled={{formID.invalid}} type="submit">Submit</ion-button>
<ion-button (click)="send(formID)">Send</ion-button>
To be used in custom code, the variable is available as this.<Form ID>
:
if (this.formID.valid) this.submit();
Updated 15 days ago