Ionic: Working with Appery.io Database Binary Data
Sample Ionic app which shows how to work with binary data in the cloud database.
Introduction
In this document, you’ll learn how to build an Ionic mobile app that can get and send binary data from/to a cloud database.
We will demonstrate how to get the binary file from the Appery.io Database (check out here for its setup) and save it back, but you can use this approach working with third-party APIs.
Setting Up Cloud Database
First, we will need to setup the database.
Important Pre-condition!
First of all, create an Appery.io database (you are also free to use an existing database) and name it myFilesDB.
Please check below to learn how to create a new database.
Creating New Database
- Create a new database from the Databases tab, click Create new database:
- For the database name, enter myFilesDB and click Create.
When created, the new database will display the list of predefined collections. Also, you can create custom collections.
- Upload some file (here, it's
.gif
) to the default Files collection - it will be used as a data source.
Important note!
You are free to upload as many files as needed but note that the maximum upload file size is 20MB.
Getting Database Master API Key
The Master API Key of the created database can be found under the Databases > Settings tab:
API Key
A database API key is always used when making requests to REST API. It is added as X-Appery-Database-Id header (can also be found in the browser address bar).
Important Security Issue
Please be informed that using the database X-Appery-Master-Key can be insecure as it gives full access to the database, so it is recommended that you use X-Appery-Session-Token instead if you need to add extra protection to your data.
Creating New App
- To create a new Ionic app, under the Apps tab of the Appery.io platform, click the Create new app button.
- In the new window, provide the new app's name, for example, Ionic Binary Data App (or any other name you like), and confirm by clicking Create.
- After the App Builder loads, open Pages >Screen1 in the Project view tree, select Toolbar Title of the screen Header and change its Common > Text property to Binary Data App. You are also free to type right in the mobile frame:
Tip!
Using the OUTLINE view can be helpful in locating the needed component and/or arranging UI components on the screen.
- Now, select the Page component from the breadcrumbs (you can also use the OUTLINE view to locate the needed component) and set the page Footer property to False as we will not use it in the app (you will also be asked to confirm changing the property):
Now, when the app and database are created, you can move to creating the app UI.
Creating App UI
- Drag & drop three Button components from PALETTE to the Content area of the Screen1 page and modify their Common > Text properties correspondingly: Get file, Save as binary, and Save as blob:
Creating App Logic
- In your app, go to the Project > Model and storage > Storage tab and add a new variable,
data
of Any type:
For uploading files to the database, we will need to link this database by importing the corresponding service.
- To import the needed database services (here, Create, Upload, and Read) click CREATE NEW > Database Service, select the needed database from the list (here, myFilesDB) select the _files > Create, Upload and Read options and confirm by clicking Import selected services:
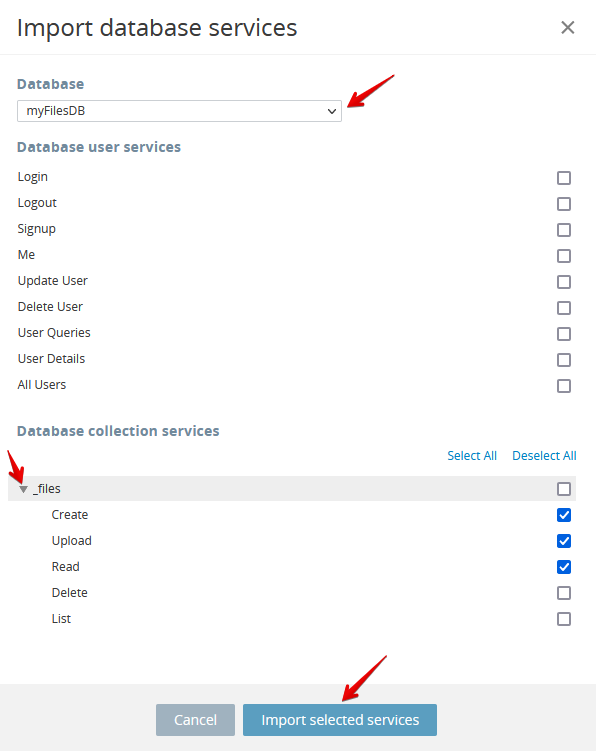
- Four database services will be instantly imported into the Services > MyFilesDB folder under the App Builder Project view tree: myFilesDB__files_create_service, myFilesDB__files_read_service, myFilesDB__files_upload_service and myFilesDB_settings:
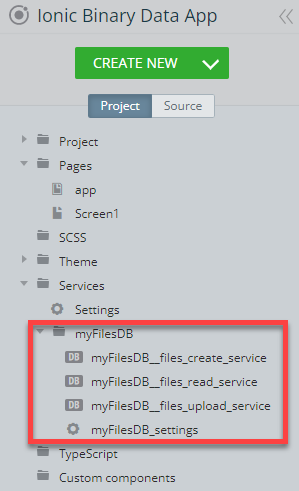
Since we are not planning to integrate any login functionality into this particular app, we will use the database X-Appery-Master-Key.
Important Security Issue
Please be informed that using the database X-Appery-Master-Key can be insecure as it gives full access to the database, so it is recommended that you use X-Appery-Session-Token instead if you need to add extra protection to your data.
- First, open the myFilesDB_settings file, add the X_Appery_Master_Key parameter (click the Add button) and define it with the X-Appery-Master-Key value of your database (can be found on the Databases > Settings page and you can check here for where to find it):
- Now, switch to the myFilesDB__files_create_service and edit its X-Appery-Session-Token request header name by replacing it with X-Appery-Master-Key.
- Now, provide the service X-Appery-Master-Key value as {myFilesDB_settings.X_Appery_Master_Key}:
- Repeat steps 5-6 for the myFilesDB__files_read_service and myFilesDB__files_upload_service services.
Reading Binary Data
You may need to read binary data when you want to download any binary file (image, archive, or whatever) from the Appery.io Database or any other third-party APIs. For example, you can read images from third-party services and save them in your DB, or send them to another third-party service.
- Switch to the Screen1 DATA panel.
- For the datasource, select myFilesDB__files_read_service, click Add and rename the service to readSrv:
- Now, go to the Files collection of myFilesDB database and copy the name of the file you want to read:
- Now, go back to the Screen1 DATA panel and for the readSrv service, click Mapping for its Before send event, paste the copied file name to the file_name field and save the mapping:
- Then click Mapping for the Success event and create the following mapping:
- Now, click the green gear button next to the Image > Src [str] property to open the Transformation editor:
- For transformation, select Custom TS and, in the Selected transformations section, paste the
return "data:;base64," + btoa(value);
,wherebtoa(value)
encodes received data to base64. Then click Save.
The green button should become blue when the change is saved. - Finally, click Save & Replace to exit the mapping editor:
- To add the toast indicating the successful file upload, click the arrow button next to the service Success event and select the Present toast action from the list. Then define the action like the following:
- Message = Done!;
- Color = success;
- Position = middle;
- Duration = 500.
Click Save and the event will be instantly added:
- Similarly, add the Present toast action to the Error event to show an error message about an unsuccessful upload defined like this:
- Message = Sorry, some error occurred;
- Color = danger;
- Position = middle;
- Duration = 500:
- Go back to the DESIGN panel and open the EVENTS tab.
- Select Button1 > Click > Invoke service > readSrv and click Save:
Testing Read Service
Now, when you have defined the service that can read files from your database and doesn't need authentication, you can test the service in preview: click the TEST button on the App Builder Toolbar, then click GET FILE:
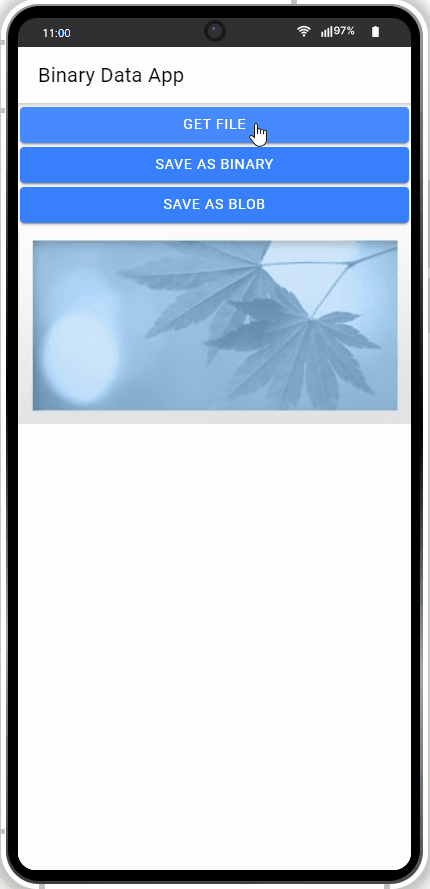
Sending Binary Data
Also, you can send file’s content as raw binary data (ArrayBuffer), or as a Blob inside multipart form data. It depends on the third-party API that used. Let’s try both variants.
Sending ArrayBuffer
- Open Screen1 DATA panel.
- For the datasource, select myFilesDB__files_create_service, click Add and rename the service to binaryArraySrv:
- Click Mapping for the Before send event and create the following mapping:
- Enter the MIME-type of the transmitted file in Content-Type property (in our case, it’s
image/gif
) for the file_name property, enter the file name, for examplebinary.gif
:
Note!
If working with other data types, for example
.jpeg
, you will need to adjust to the needed extension:
- Then, click the green gear button next to the data [any] property to open the Transformation editor and add the following code as Custom TS:
//transform string to ArrayBuffer
function str2ab(str) {
var buf = new ArrayBuffer(str.length);
var bufView = new Uint8Array(buf);
for (var i=0, strLen=str.length; i < strLen; i++) {
bufView[i] = str.charCodeAt(i);
}
return buf;
}
return str2ab(value);
The green gear button should become blue when the change is saved (don't forget to click the Save button to do it).
- Finally, click Save & Replace to exit the mapping editor.
- Delete the Success mapping button since it will not be used in the app but let's add some success/error toasts instead.
- To add the toast indicating the successful file upload, click the arrow button next to the service Success event and select the Present toast action from the list. Then define the action like the following:
- Message = Done!;
- Color = success;
- Position = middle;
- Duration = 500.
- Click Save and the event will be instantly added:
- Similarly, add the Present toast action to the Error event to show an error message about an unsuccessful upload defined like this:
- Message = Sorry, some error occurred;
- Color = danger;
- Position = middle;
- Duration = 500:
- Go back to the DESIGN panel and open the EVENTS tab.
- Select Button2 > Click > Invoke service > binaryArraySrv and click Save:
- Save the app by clicking the SAVE button on the App Builder Toolbar.
Testing Sending ArrayBuffer Service
The service is defined so let's restart the app: click the TEST button on the App Builder Toolbar, then click GET FILE and then hit the SAVE AS BINARY button:
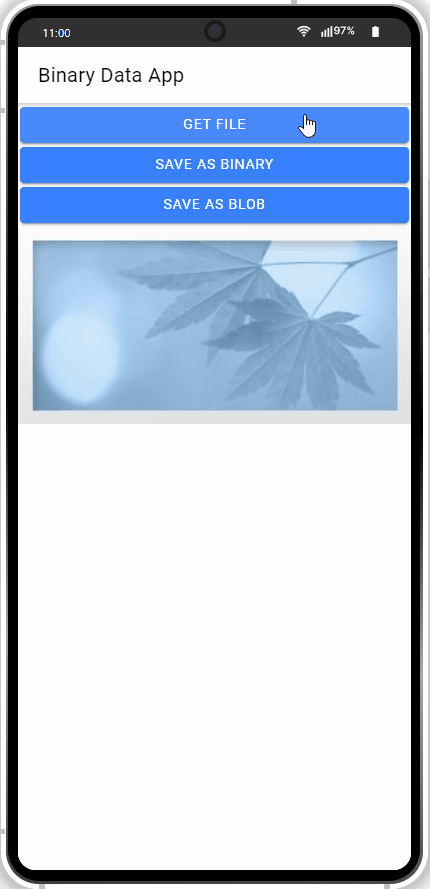
In a moment, you can open your database (here, it's myFilesDB) to check its Files collection and make sure that new file entry is already there (you may need to click the Refresh button to update the list):
Sending Blob
- Open Screen1 DATA panel.
- For the datasource, select myFilesDB__files_upload_service, click Add and rename the service to blobSrv:
- Click Mapping for the Before send event and create the following mapping:
- Then, click the green gear button next to the data [any] property to open the Transformation editor and add the following code as Custom TS:
function str2ab(str) {
var buf = new ArrayBuffer(str.length);
var bufView = new Uint8Array(buf);
for (var i=0, strLen=str.length; i < strLen; i++) {
bufView[i] = str.charCodeAt(i);
}
return buf;
}
var ab = str2ab(value);
var blob = new Blob([ab], {type: "image/jpeg"}); //here you can set file's mime type
var fd = new FormData();
fd.append('blob.jpg', blob); //here you can set file's name
return fd;
- Click Save. The green gear button should become blue when the change is saved.
- Finally, click Save & Replace to exit the mapping editor.
- Delete the Success mapping button since it will not be used in the app and add some success/error toasts instead.
- To add the toast indicating the successful file upload, click the arrow button next to the service Success event and select the Present toast action from the list. Then define the action like the following:
- Message = Done!;
- Color = success;
- Position = middle;
- Duration = 500.
- Click Save and the event will be instantly added:
- Similarly, add the Present toast action to the Error event to show an error message about an unsuccessful upload defined like this:
- Message = Sorry, some error occurred;
- Color = danger;
- Position = middle;
- Duration = 500:
- Go back to the DESIGN panel and open the EVENTS tab.
- Select Button3 > Click > Invoke service > blobSrv and click Save:
- Save the app by clicking the SAVE button on the App Builder Toolbar.
Testing Sending Blob Service
To test uploading blob file to the linked database, click the TEST button on the App Builder Toolbar, then click GET FILE and then press the SAVE AS BLOB button making sure the success toast is shown:
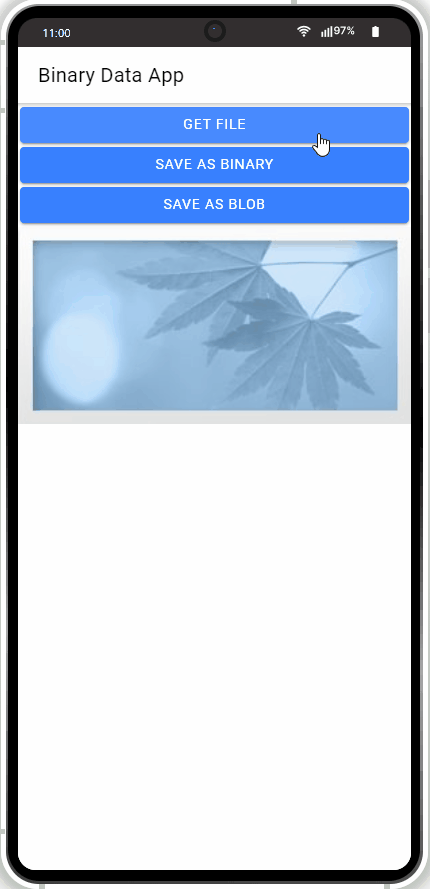
In a moment, check your database again to see that another new file has been uploaded to its Files collection (again, you may need to click the Refresh button to update the list):
Testing App on Device
Now, when you have defined all the services, you might also like to test the app on the device.
Appery.io Tester App
A great option to quickly test the app on the device is to use our Appery.io Tester app.
The app is free and available for both iOS and Android.
You can check out this page to learn more about using the Appery.io Tester app.
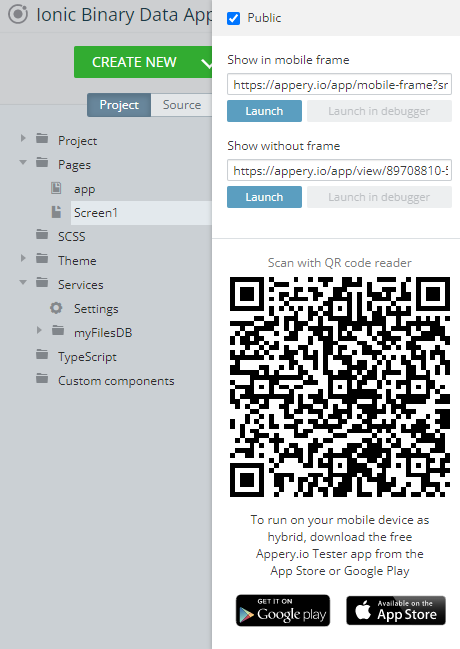
When the app loads, try clicking all the buttons:
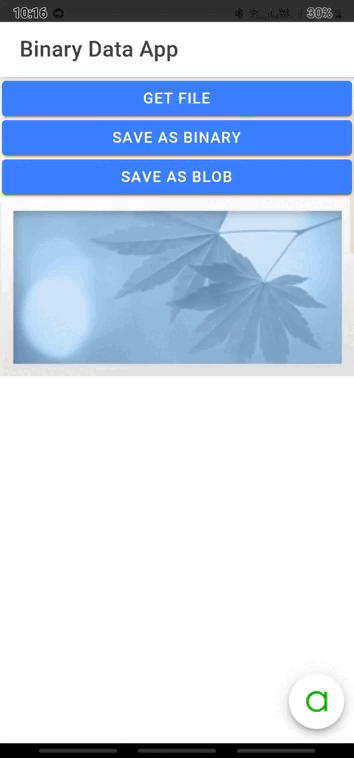
Note!
Note that this sample app is linked to the test database and you will only be able to see the success toasts informing that the files have been uploaded.
If you want to get access to the added records from under the Databases tab, you will need to set up your own database and link it to your app (like described here).
Updated 12 months ago