PayPal Sample jQM App
Sample JQM app that uses PayPal API.
Important Note!
The option of creating new apps with the jQuery Mobile framework was removed but we still support the projects that were created with it earlier.
Introduction
Important PayPal API Change - November 27, 2016
If your PayPal API authorization script has stopped working, it can be fixed by updating the Server Code script. The updated script code with description is here.
In this tutorial, you will learn how to import and use the PayPal API plugin. This plugin makes it simple to add secure payments to your Appery.io mobile app and trigger any PayPal REST API.
By using PayPal, the world-famous payment system, you can build your app to accept payments.
This tutorial will also show how to create a Server Code script to implement server-side authentication which is totally secure – your app will not contain any sensitive data such as client secret or token.
PayPal Account
To use the PayPal API, you will need to sign up for an account: http://developer.paypal.com/. Then you should create your app on the PayPal side and you will get an API key to use. All code in this tutorial will be used for PayPal sandbox (https://www.sandbox.paypal.com/) for production purposes use url without sandbox (https://www.paypal.com/).
App Parts
The complete app consists of:
- jQuery Mobile App (client).
- Backend.
The backend consists of the following:
- Database - for storing session token.
- API Express - for invoking PayPal API.
- Server Code - for authentication logic.
In the next sections, you will get and put together the various parts of the app.
Getting App
In this step, you are going to import a plugin which will be the app (client).
- From the App page, select Create new app. Enter PayPalApp for the app name.
- Select the jQuery Mobile app Blank template.
- Click the Create button.
- When the App Builder loads, in the CREATE NEW dropdown, select From Plugin, check PayPal API plugin, and click the Import selected plugins button.
- A pop-up dialog should appear. Set start page to Paypal_Home and confirm.
That's it. In the next steps, you are going to work on setting up the app backend.
Setting up Database
In this step, you will create the app backend. The first step is to create a database to store session token information.
- Under the Databases tab, create a new database called PayPalDB.
- Inside the database, create a collection called Credentials.
- Inside the Credentials collection, create two columns called: key and keyValue (both String type).
- Your database should be secure, so open the Credentials collection, click Security and permissions, and check the Secure collection option.
- Copy the created database ID and paste it into Services > PayPal_Settings > database_id inside the App Builder.
- Open the database Settings page and copy Master API key. It will be used by API Express and Server Code.
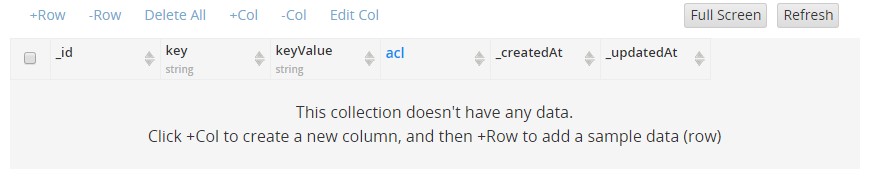
Database collection: Credentials.
Setting up API Express Project
API Express will hold services that connect to PayPal API.
- Download the API Express project backup.
- Go to the API Express page.
- Click Create new project, upload the backup, set any project name (e.g. PayPal API) and click Create.
- Open Service keys tab of API Express project and paste your Master key (from database) directly to masterKey value.
- Copy your API Express project API key from the address bar or the Settings tab. Paste into the API_EXPRESS_API_KEY field of the PayPal_Settings service in the app.
Next, you are going to get the Server Code script for authentication.
Adding Server Code Script
This app uses Server Code script to authenticate with PayPal.
- From the Server Code page, create a new script. Name the script PayPal_Authentication and click the Save button.
- Copy the script code below and paste it over the default new script you have just created.
var database_id = request.get('database_id');
var paypal_url_api = request.get('paypal_url_api');
var tokenID;
var tokenUpdatedTime;
var COLLECTION_NAME = 'Credentials';
var CLIENT_SECRET_ENCODED = '{{PUT YOUR ENCODED SECRET HERE}}';
var masterKey = '{{PUT YOUR DB MASTER-KEY HERE}}';
//Retrieving collection with token and client secret
var collection = Collection.query(database_id, COLLECTION_NAME, {
"criteria": {
"key": {
"$eq": "token"
}
}
}, masterKey);
//Filtered collection should contain only one object according to criteria
if (collection.length) {
var tokenID = collection[0]._id;
var tokenUpdatedTime = collection[0]._updatedAt;
}
//If token already exists, then check if it's expired
if (tokenID) {
var updatedTime = new Date(tokenUpdatedTime);
var now = new Date();
console.log("Token exists. It's alive for " + (Math.round((now - updatedTime)) / 1000).toFixed(0) + ' sec');
//Check if token is expired
if ((Math.round((now - updatedTime)) / 1000).toFixed(0) >= 28800) {
console.log('Token expired, issuing new one');
//Sending XHR for new token
sendXHR();
} else {
//Token exists and it's not expired so just send a success
console.log("Token exists and it's not expired. ");
response.success({
'Message': 'Authorization successful. Token still alive.'
}, 'application/json');
}
} else {
console.log('Token does not exist. Creating new one');
//If there is no token, let's issue one
sendXHR();
}
function sendXHR() {
var XHRResponse = XHR2.send("POST", "https://api.sandbox.paypal.com/v1/oauth2/token", {
"headers": {
'Authorization': 'Basic ' + CLIENT_SECRET_ENCODED,
'Content-Type': 'application/x-www-form-urlencoded',
'Accept': 'application/json'
},
'body': 'grant_type=client_credentials'
});
var status = XHRResponse.status + '';
//If XHR response status doesn't start from 2 digits
if (status.charAt(0) != '2') {
response.error({'Message':'Error during authorization. ' + 'Status: ' + status});
} else {
try { //If we're here and tokenID exists, we should update it
if (tokenID) {
Collection.updateObject(database_id, COLLECTION_NAME, tokenID, {
"key": "token",
"keyValue": XHRResponse.body.access_token
}, masterKey);
response.success({
'Message': 'Authorization successful. Token updated.'
}, 'application/json');
} else {
//If we're here, token doesn't exist so let's create it
Collection.createObject(database_id, COLLECTION_NAME, {
"key": "token",
"keyValue": XHRResponse.body.access_token
}, masterKey);
response.success({'Message': 'Authorization successful. Token stored in the database.'}, 'application/json');
}
} catch (e) {
response.error({'Message':'Error during saving / updating token. ' + e}, 'application/json')
}
}
}
- Inside the script, replace {{PUT YOUR DB MASTER-KEY HERE}} template with your database Master key.
- Replace {{PUT YOUR ENCODED SECRET HERE}} template with your PayPal app client ID and secret separated by a colon and encoded as base64. For example, you should combine the following string:
ATQ8cxB02m5DRQ7xDOFbazqISjUUhCsXVjkMlmYkpOqw1oxg4uCqkvhJEruF:EBYoDSDQa_gGBADGWLdooA3hd5qBVk7xvfKZWio4Bosajr4p8JZwdi_PJleD
and then encode it as base64.
- Copy the Server Code script API Key from the address bar or from the API Information tab and fill auth_script_id parameter of PayPal_Settings
Setting Return and Cancel URLs
The last step of the setup is to fill up the return_url and cancel_url parameters in PayPal_Settings. This plugin doesn’t handle payment canceling in any special way, so the URLs will be the same. But if you want to handle payment cancels you can use a unique URL for cancel_url. Change <YOUR_APP_ID> to your Appery.io app ID (copy it from the address bar) and paste the following string to return_url and cancel_url parameters.
For a jQuery Mobile app:
https://appery.io/app/view/<YOUR_APP_ID>/app/PayPal_Payment_Details.html
For an Ionic app:
https://appery.io/app/view/<YOUR_APP_ID>/#/PayPal_Payment_Details.html
If you’re using library version 3.0 or lower, the URL will look like this:
http://appery.io/app/view/<YOUR_APP_ID>/PayPal_Payment_Details.html
Running App
That’s all you need to do. You can now run the app by clicking the Test button inside the App Builder. Due to security, you need to launch the app without the mobile frame.
Once the app is loaded, the Server Code script will be triggered.
The home page contains the predefined amount of money (6.99) and a select component to change the currency (USD or EUR).
Click Make Payment to create a payment. This is the same if the user would click a buy button in your app.
Once the payment is created, you (or the buyer) will be redirected to the PayPal page. On this page, the buyer credentials should be entered. You can use one of the PayPal test accounts to test the app.
Once the payment is approved on behalf of the buyer, the PayPal_Payment_Details page will be opened. The payment details will be shown there.
When you are done testing the app, don't forget to change paypal_url_api from sendbox to the PayPal production servers before going live.
Updated about 2 years ago