WebSocket
WebSocket Example plugin
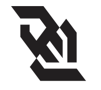
The WebSocket Example plugin is designed to facilitate real-time, bidirectional communication between a client and a server over a persistent connection. It leverages the WebSocket protocol, which allows data to be exchanged as "packets" without the need for additional HTTP requests. This makes it particularly useful for services that require continuous data exchange, such as online games or real-time trading systems.
Note!
Please make sure to install the express and socket.io npm modules.
Sample server node.js
code.
const express = require("express");
const http = require("http");
const { Server } = require("socket.io");
const app = express();
const server = http.createServer(app).listen(8080);
app.get("/", (req, res) => {
return res.json({status: "ok"});
});
const io = new Server(server, {
cors: {
origin: "*",
methods: ["GET", "POST"],
},
});
const clients = {};
let clientIndex = 0;
io.on("connection", (socket) => {
const id = clientIndex++;
console.log("connected", id);
clients[id] = { socket: socket };
socket.on("disconnect", () => {
console.log("disconnected", id);
delete clients[id];
});
socket.on("message", (data) => {
console.log("message " + id, data);
for (let clientId in clients) {
if (id != clientId) clients[clientId].socket.emit("message", data);
}
});
});
We also offer a detailed video tutorial explaining how you can to add websocket support to mobile and web applications:
Updated 3 months ago