Create-Read-Update-Delete jQM App
A sample CRUD app built with jQuery Mobile.
Important Note!
The option of creating new apps with the jQuery Mobile framework was removed but we still support the projects that were created with it earlier.
This app demonstrates how to work with objects stored in a database. The app shows how to perform the following actions:
- Create;
- Read;
- Update;
- Delete.
These actions are usually referred to as CRUD (taking the first letter).
Want to know more?
You might also like to check our Ionic Todo List Tutorial on creating an app for adding, editing, deleting, and updating items in a connected Appery.io database.
This app shows the following features:
- jQuery Mobile UI;
- Database;
- Server Code;
The sample app setup includes the following general steps:
- Creating a database to store data.
- Adding Server Code scripts. Scripts hold the app logic for accessing the database and retrieving data for the app (client).
- Adding app UI (as a plugin) built with jQuery Mobile.
- Connecting the app to the mobile backend.
- Testing the app.
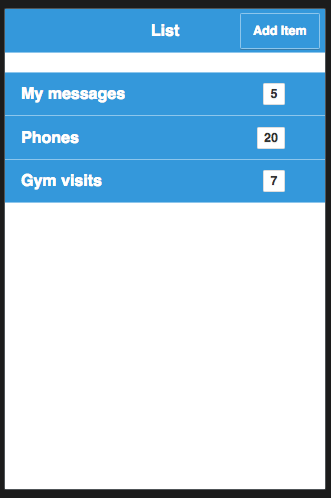
List page.
Creating Database
The database will hold the app data.
- From the Database page, click Create new database. Name it Crud_db.
- In the newly created database create a new collection. Name the collection: Items.
- Inside the collection, create two columns:
- name (String type);
- count (Number type).
- Enter one or two sample records into the collection by clicking the +row link. You can enter any random data.
That's all you need to do for the database. Your collection should look like this (with sample data):
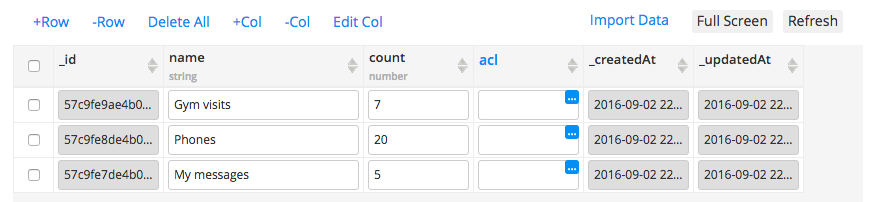
Collection with sample data.
The next step is to add Server Code scripts.
Server Code Scripts
Server Code scripts provide server-side app logic. The scripts will access the database and make data available for the app. You will create five scripts:
- List script;
- Create script;
- Read script;
- Update script;
- Delete script.
All the scripts are small and the setup is identical. Let's start with the script to retrieve all items from the database.
Creating Script to List all Items
To create the script:
- From the Server Code page, click the Create script button. The Server Code editor will open.
- For script name enter: list_script.
- Copy and paste the following code:
var dbApiKey = "database-api-key";
var result = Collection.query(dbApiKey, "Items");
Apperyio.response.success(result, "application/json");
You only need to set the database API key. The database API key can be found on the database you just created (to locate it, go to its Settings tab and copy the database ID (API key) to clipboard). After you set the API key you are ready to test the script.
Testing Script
To test the script, open the Run tab (right-hand side). As this script doesn't take any parameters, click the Save and run button to test the script. You should see the items you added to the database.
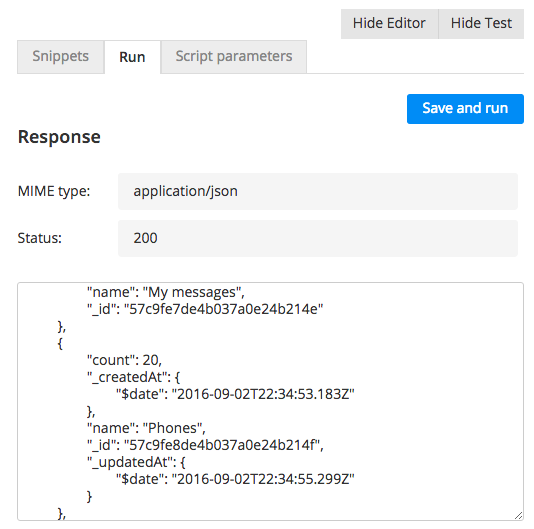
Testing the script.
Creating Script to Add New Item
This script will create a new item in the database.
- Create a new script and name it: create_script.
- Copy and paste the following code:
var dbApiKey = "database-api-key";
var name = request.get("name");
var count = request.get("count");
var result = Collection.createObject(dbApiKey, "Items", {
"name": name,
"count": count
});
var updated = ScriptCall.call("list_script_ID");
Apperyio.response.success(updated.body, "application/json");
There is one more step here and that's setting the ID of the list_script. Once a new item has been added, the script will query the database again and get the updated list to return to the app. With ScriptCall.call you can easily re-use/invoke an existing script.
To find the ID of the script, open list_script and then open the API information tab. There you will see a URL for the script with the ID. Copy that ID and set it into the script above.
Testing Script
To test this script, you first need to create two input parameters. To create the parameters:
- Switch to the Script parameters tab.
- There create two parameters: name and count and enter any sample data for them.
- Go back to the Run tab and click the Save and run button.
A new item will be added to the database and you will see an updated list as the script result.
Creating Script to Retrieve Item
This script will allow you to read (retrieve) a particular item. This is needed when editing or deleting an item.
- Create a new script and name it: read_script.
- Copy and paste the script code:
var dbApiKey = "database-api-key";
var itemId = request.get("itemId");
var result = Collection.retrieveObject(dbApiKey, "Items", itemId);
Apperyio.response.success(result, "application/json");
- In this script, you only need to set the database API key.
Testing Script
To test this script, you need to create one input parameter called: itemId. To set sample data, go to the database and copy and value under the _id column.
It's important to test the script and ensure it works before using it in the app.
Creating Script to Update Item
This script will allow you to update an item in the database.
- Create a new script and name: update_script.
- Copy and paste the script code:
var dbApiKey = "database-api-key";
var name = request.get("name");
var count = request.get("count");
var itemId = request.get("itemId");
var result = Collection.updateObject(dbApiKey, "Items", itemId, {"name":name, "count":count});
var updated = ScriptCall.call("list_script_ID");
Apperyio.response.success(updated.body, "application/json");
- In this script, you need to set the database API key and set the list_script ID.
Testing Script
To test this script, you need to create three input parameters:
- name;
- count;
- itemId.
Don't forget to test the script and ensure it works before using it in the app.
The last script is to delete an item.
Creating Script to Delete Item
This script will delete an item from the database.
- Create a new script and name it: delete_script.
- Copy and paste the script code:
var dbApiKey = "database-api-key";
var itemId = request.get("itemId");
Collection.deleteObject(dbApiKey, "Items", itemId);
var updated = ScriptCall.call("list_script_ID");
Apperyio.response.success(updated.body, "application/json");
- In this script, you need to set the database API key and set the list_script ID.
Testing Script
To test this script, you need to create one input parameter:
- itemId.
It's important to test the script and ensure it works before using it in the app.
You are done with the app logic. Next, you are going to work on the app.
Getting App
The app is available as a plugin which you can quickly import inside the App Builder.
- From the Apps page click the Create new app button.
- For the app name, enter CrudApp.
- Select the jQuery Mobile tab, then select Blank. Click the Create button.
- When the visual editor loads, click CREATE NEW > From plugin > Create-Read-Update-Delete App.
- Click the Import selected plugins button.
- On the next screen, select List as the start page and click Apply settings.
The app is now imported. The app has all the pages and services pre-configured. The only step that is left is to connect the app to the backend.
Connecting App with Backend
The app has five pre-configured services:
- create_script_service;
- delete_script_service;
- update_script_service;
- list_script_service;
- read_script_service.
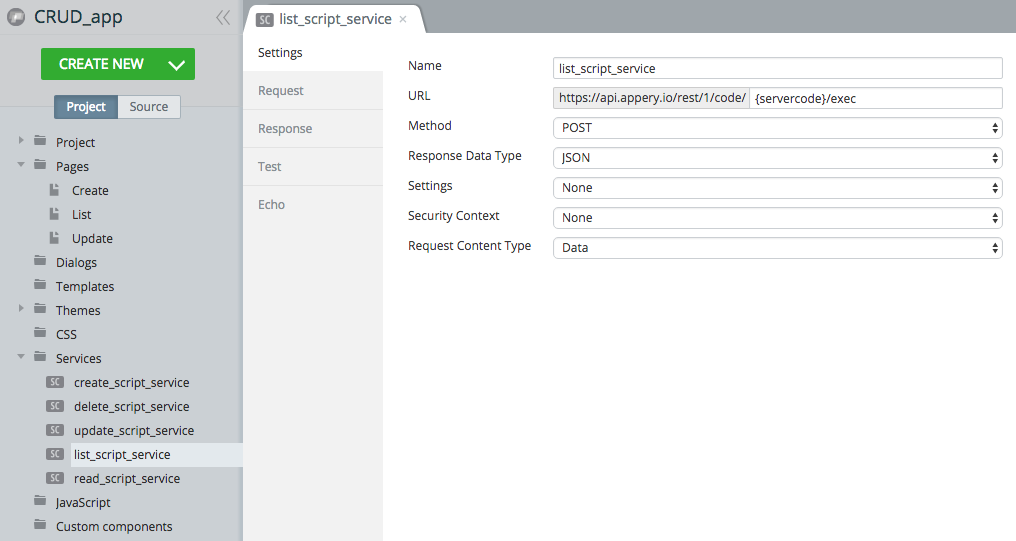
App services.
When you open a service you will see the the URL (the Settings tab) has the {server code}
placeholder. Replace this placeholder with Server Code script ID. The ID for each script can be found on the API information page.
After you have connected the app with the backend it's time to test the app.
Testing App
You are now ready to test the app. Click the Test button in the App Builder to launch the app in the browser.
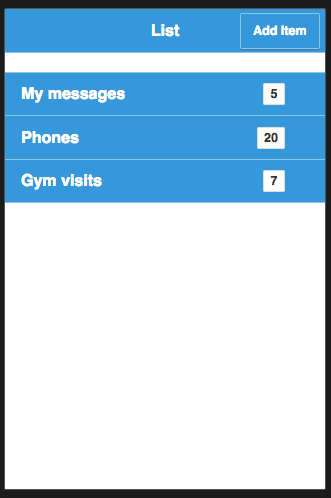
List page.
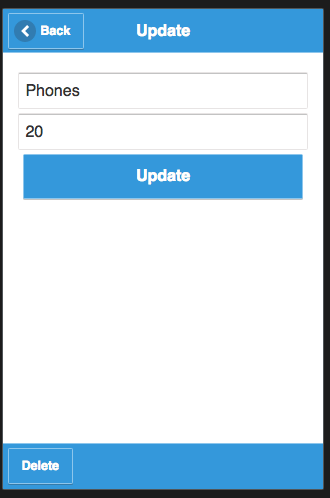
Update page.
Updated almost 2 years ago