Google Analytics (deprecated needs a new revision)
Using the Google Analytics API
What are we going to build?
In this tutorial, you’ll learn how to build an app that tracks data or user actions via the PhoneGap Google Analytics plugin.
The app will consist of two pages, and they will look like:
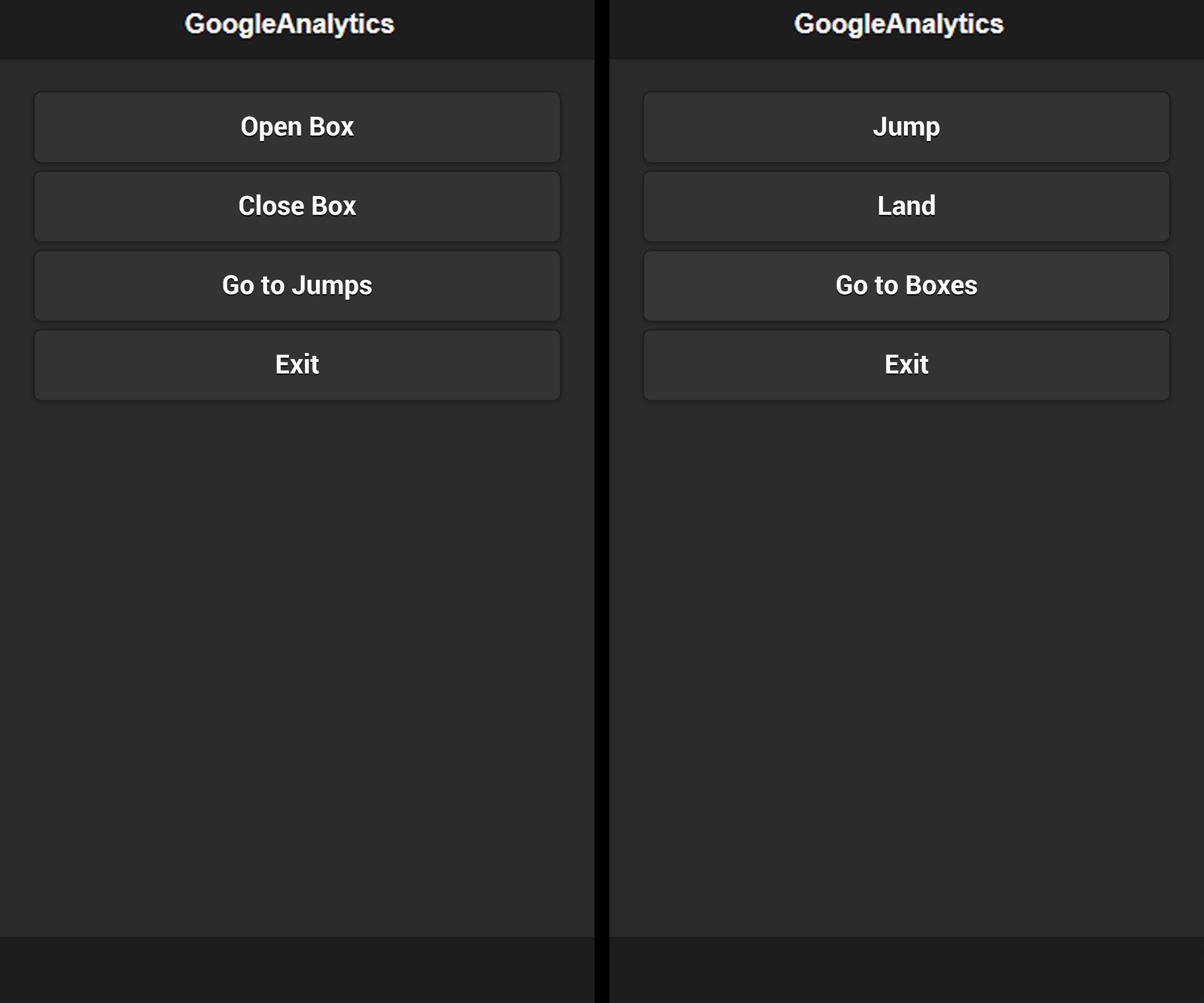
Apache Cordova (PhoneGap)
Apache Cordova (PhoneGap) is automatically included when you create a new project in Appery.io.
Creating a new app
Create a new app in the builder. From the apps page, enter an app name (use any name) and click Create.
Creating the UI
Lets start with the first page:
- Open the Pages folder. Click the gear icon to the right of startScreen, and select Rename. Rename it to boxesScreen.
- Place 4 buttons on the page.
- Select the first button, and set its Text property to Open Box. Set it’s Name property to open_box.
- Select second button and set its Text property to Close Box. Set its Name property to close_box.
- Select third button and set its Text property to Go to Jumps. Set its Name property to go_to_jumps.
- Select the fourth button and set its Text property to Exit. Set its Name property to exit.
That’s it for first page. Now, create the second page the same way:
- In the right of the screen, click Create New > Page, name it jumpsScreen.
- Place 4 buttons on the page.
- Select the first button and set its Text property to Jump. Set its Name property to jump.
- Select the second button and set its Text property to Land. Set its Name property to land.
- Select the third button and set its Text property to Go to Boxes. Set its Name property to go_to_boxes.
- Select the the fourth button and set its Text property to Exit. Set its Name property to exit.
That’s it for the UI. Now you need to add some JavaScript.
Adding JavaScript code
It’s useful to keep all the needed functions in a separate JavaScript file:
- From the Projects view, click Create New > JavaScript, enter its name: ga, and click Create JavaScript. The new JavaScript file will be listed under the JavaScript folder.
- Open the ga.js file, and add the following code:
var gaSuccessHandler = function(result) {
alert('GA initialized: ' + result);
};
var gaErrorHandler = function(error) {
alert('GA initialization failed: ' + error);
};
var gaNativePluginResultHandler = function(result) {
alert('Event tracked: ' + result);
};
var gaNativePluginErrorHandler = function(error) {
alert('Event tracking failed: ' + error);
};
var getGAPlugin = function() {
if (GAPlugin) {
console.log('GA Plugin found');
return GAPlugin;
}
console.log('GA Plugin NOT found');
};
var initGA = function() {
console.log('Initialize GA');
if (getGAPlugin()) {
getGAPlugin().init(gaSuccessHandler, gaErrorHandler, "ENTER_YOUR_GOOGLE_ANALYTICS_ID_HERE", 10);
}
};
var trackPage = function(pageName) {
console.log("trackPage: " + pageName);
if (getGAPlugin()) {
getGAPlugin().trackPage(gaNativePluginResultHandler, gaNativePluginErrorHandler, pageName);
}
};
var trackEvent = function(category, eventAction, eventLabel, eventValue) {
console.log("trackEvent: " + category);
if (getGAPlugin()) {
getGAPlugin().trackEvent(gaNativePluginResultHandler, gaNativePluginErrorHandler, category, eventAction, eventLabel, eventValue);
}
};
- Note the string 28. You should use your Google Analytics ID. It can be found on the Google Analytics website, to the right of the created application:
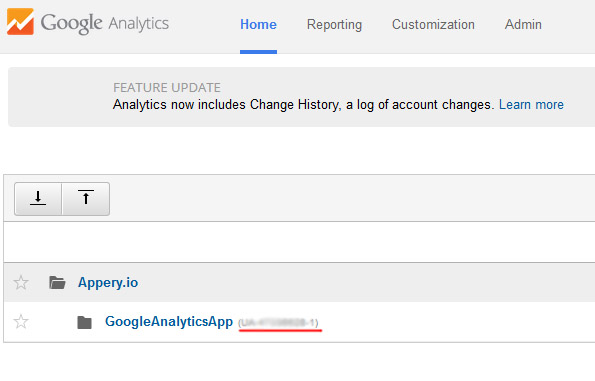
Adding the events
Now you need to add initialize and tracking events to the components:
- Select boxesScreen. Open the EVENTS tab, and add the following event:
boxesScreen > Device ready > Run JavaScript. Add the following code:
initGA();
trackPage('boxes');
This code will initialize the plugin and track that the boxes page was opened.
- Click Save.
- Add one more event directly to the boxesScreen:
boxesScreen > Device ready > Run JavaScript. Add the following code and save:
trackPage('boxes');
This code will track that the boxes screen was opened when a transition was performed from another page.
- Select the open_box button. Add the following event:
open_box> Click > Run JavaScript. Add the following code and save:
trackEvent("Box", "Open", "times", 1);
This code will track the Open event in the Box category.
- Select the close_box button. Add the following event: close_box> Click > Run JavaScript. Add the following code:
trackEvent("Box", "Close", "times", 1);
This code will track the Close event in the Box category.
7. Click Save.
8. Select the go_to_jumps button. Add the following event: go_to_jumps> Navigate to page> jumpsScreen.
9. Save.
10. Select the exit button. Add the following event: exit > Click > Run JavaScript, and add the following code:
navigator.app.exitApp();
Now, let's add events to the second page. Under the Pages folder, select the jumpsScreen page:
- Open the EVENTS tab, and add the following event:
jumpsScreen > Page show > Run JavaScript. Add the following code:
trackPage('jumps');
This code will track that jumps screen opens when there’s a transition from another page.
2. Click Save.
3. Select the jump button. Add the following event:
jump > Click > Run JavaScript with the following code and save:
trackEvent("Jumps", "Jump", "height", 1.5);
Testing the app
Since we’re invoking a native component, the app needs to be tested as a hybrid app, or installed on the device.
The final app will look like:
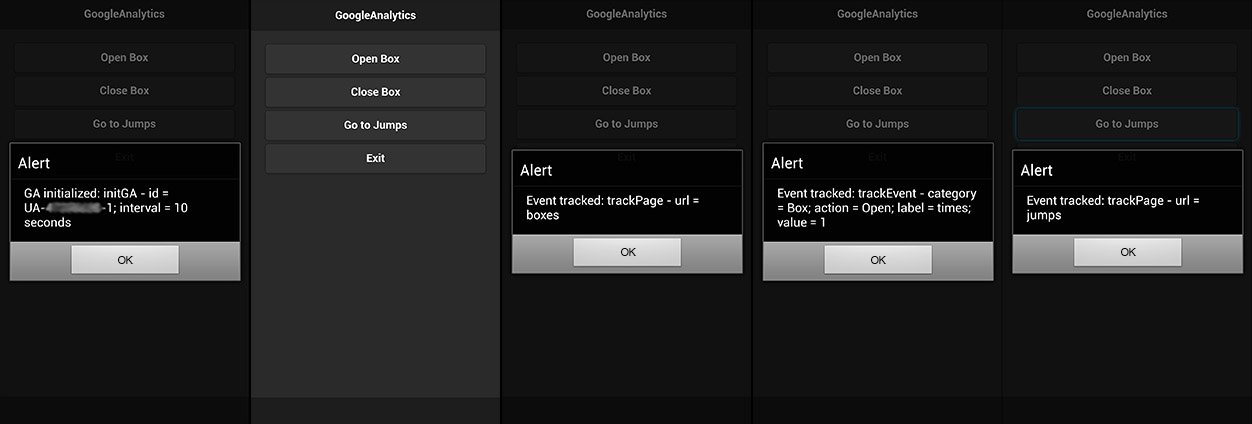
Android
Android testing options:
Build the Android binary and install it on your device. When the build is completed, you’ll see a QR code. Scanning the QR code will download the app to your phone. You can also email the app to your device.
iOS
iOS options:
Build the iOS binary and install it on your device. When the build is completed, you’ll see a QR code. Scanning the QR code will download the app to your phone.
Updated almost 5 years ago